Declare ArrayList in class Java
Declare ArrayList in class Java
I'll respond in English this time!
In Java, an ArrayList
is a resizable array-based data structure that implements the List
interface. It's a part of the Java Collections Framework, which provides a way to store and manipulate collections of objects.
Here's how you can declare an ArrayList
in Java:
import java.util.ArrayList;
import java.util.List;
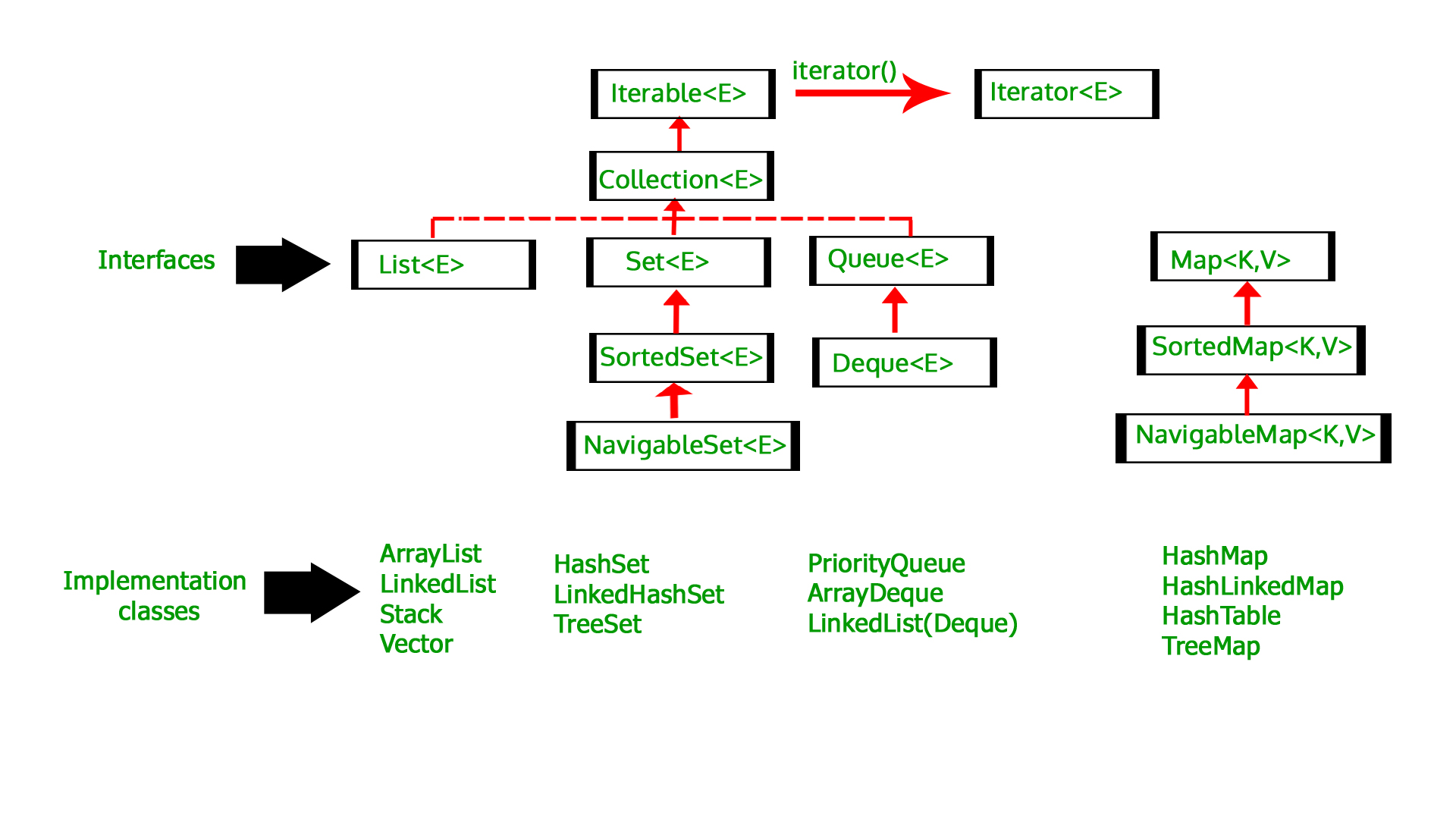
public class ArrayListExample {
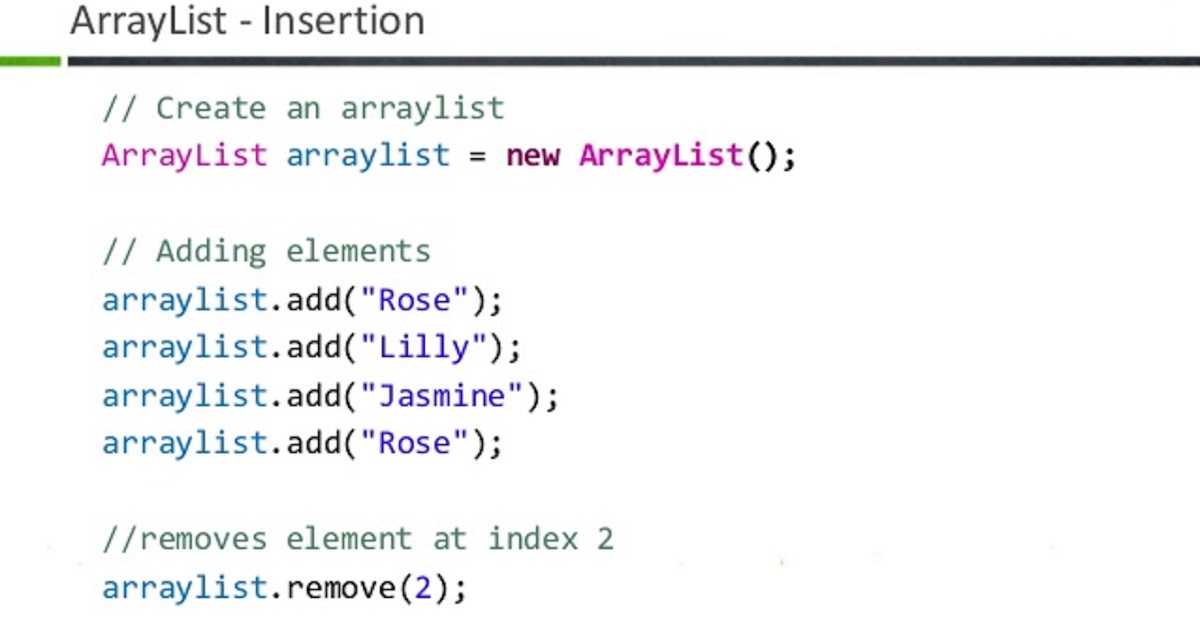
public static void main(String[] args) {
// Declare an ArrayList
List myArrayList = new ArrayList<>();
// Add elements to the array list
myArrayList.add("Apple");
myArrayList.add("Banana");
myArrayList.add("Cherry");
// Print the array list
System.out.println("Initial Array List: " + myArrayList);
// Modify the array list
myArrayList.set(0, "Avocado"); // Replace the first element
// Print the modified array list
System.out.println("Modified Array List: " + myArrayList);
}
}
In this example:
We import theArrayList
and List
classes from the java.util
package. We declare a variable myArrayList
of type List<String>
(a generic List
that can hold strings). We create an instance of ArrayList
using the new
keyword, which allows us to store and manipulate a collection of strings. We add elements to the array list using the add()
method. We print the initial array list to the console. We modify the array list by replacing the first element with "Avocado" using the set()
method. Finally, we print the modified array list to the console.
The output of this program will be:
Initial Array List: [Apple, Banana, Cherry]
Modified Array List: [Avocado, Banana, Cherry]
ArrayLists are useful when you need a dynamic collection that can grow or shrink as elements are added or removed. They are commonly used in Java programs for tasks such as storing and processing data, managing game objects, or handling user input.
How to store class objects in ArrayList in Java
I apologize for the confusion earlier!
Storing class objects in an ArrayList in Java is a common practice when you need to manage a collection of custom objects. Here's how you can do it:
Step 1: Define your class
Let's assume we have a Student
class with some properties like id
, name
, and grade
. We'll define this class first:
public class Student {
private int id;
private String name;
private double grade;
public Student(int id, String name, double grade) {
this.id = id;
this.name = name;
this.grade = grade;
}
// Getters and setters
public int getId() { return id; }
public void setId(int id) { this.id = id; }
// ... (and so on for other properties)
}
Step 2: Create an ArrayList
Next, we'll create an ArrayList
to store our Student
objects:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List students = new ArrayList<>();
}
}
Step 3: Add objects to the ArrayList
Now, let's create some Student
objects and add them to our ArrayList
:
// Create a few Student objects
Student student1 = new Student(1, "John", 85.0);
Student student2 = new Student(2, "Jane", 92.5);
Student student3 = new Student(3, "Bob", 78.0);
// Add them to the ArrayList
students.add(student1);
students.add(student2);
students.add(student3);
Step 4: Iterate and retrieve objects from the ArrayList
Finally, let's iterate through our ArrayList
using a for-each loop and print out some information about each student:
// Print out student info
for (Student s : students) {
System.out.println("ID: " + s.getId() +
", Name: " + s.getName() +
", Grade: " + s.getGrade());
}
This code snippet demonstrates how to store Student
objects in an ArrayList
. By following these steps, you can easily manage a collection of custom Java objects.
Additional tips:
Make sure your class has a proper constructor (as we did with theStudent
class). Use getters and setters (like we did) to encapsulate the object's properties. When adding objects to an ArrayList
, you can use the add()
method or one of its overloaded variants, such as add(index, obj)
if you want to insert at a specific position.
Hope this helps!