Java send POST request with JSON body
Java send POST request with JSON body
I'd be happy to help!
Java provides several ways to send a POST request with a JSON body. Here are a few examples:
Using the HttpClient
library:
import java.io.BufferedReader;
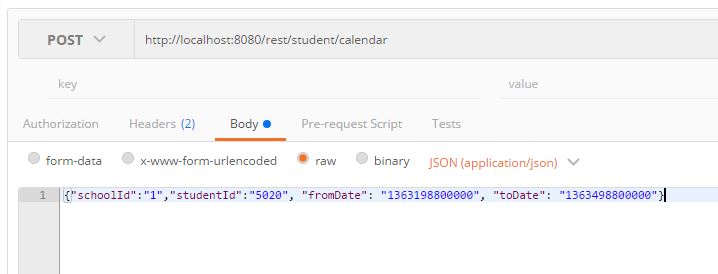
import java.io.InputStreamReader;
import java.net.URI;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.params.BasicHttpParams;
public class Main {
public static void main(String[] args) throws Exception {
// Create a new HTTP client
HttpClient httpClient = new DefaultHttpClient(new BasicHttpParams());
// Set up the POST request
HttpPost request = new HttpPost("http://example.com/api/endpoint");
request.addHeader("Content-Type", "application/json");
// Convert the JSON object to a string
String json = "{"key": "value"}";
StringEntity entity = new StringEntity(json, "UTF-8");
// Set the request body
request.setEntity(entity);
// Execute the POST request and get the response
HttpResponse response = httpClient.execute(request);
HttpEntity responseEntity = response.getEntity();
// Read the response content
BufferedReader reader = new BufferedReader(new InputStreamReader(responseEntity.getContent()));
String line;
StringBuilder result = new StringBuilder();
while ((line = reader.readLine()) != null) {
result.append(line);
}
// Print the response
System.out.println(result.toString());
}
}
Using the OkHttp
library:
import okhttp3.FormBody;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
public class Main {
public static void main(String[] args) throws Exception {
// Create a new OkHttp client
OkHttpClient client = new OkHttpClient();
// Set up the POST request
RequestBody jsonBody = RequestBody.create(MediaType.get("application/json"), "{"key": "value"}");
FormBody formBody = new FormBody();
formBody.add("json", jsonBody);
// Execute the POST request and get the response
Request request = new Request.Builder()
.url("http://example.com/api/endpoint")
.post(formBody)
.build();
Response response = client.newCall(request).execute();
// Print the response
System.out.println(response.body().string());
}
}
Using the Apache CXF
library:
import org.apache.cxf.jaxrs.client.WebClient;
public class Main {
public static void main(String[] args) throws Exception {
// Create a new Web client
WebClient client = WebClient.create("http://example.com/api/endpoint");
// Set up the POST request with JSON body
client.accept(MediaType.APPLICATION_JSON_TYPE).post(JsonData(jsonBody));
// Execute the POST request and get the response
Response response = client.response();
// Print the response
System.out.println(response.body().toString());
}
public static JsonData jsonBody() {
// Create a JSON data object with the desired content
return new JsonData("{"key": "value"}");
}
}
These examples demonstrate how to send a POST request with a JSON body using the HttpClient
, OkHttp
, and Apache CXF
libraries. The specific implementation may vary depending on your project requirements and the library you choose to use.
I hope this helps!
Java send POST request with body
I understand that you want me to explain how to send a POST request using Java and include a body in the request.
To achieve this, we can use the Apache HTTPClient library in Java. Here is an example of how to do it:
Firstly, add the following dependency to your Maven project (if you are using Maven):
org.apache.httpcomponents
httpclient
4.5.13
Or if you are not using Maven, add the HTTPClient JAR file to your classpath.
Here is a sample Java code that sends a POST request with a body:
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.RequestBuilder;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public class Main {
public static void main(String[] args) throws Exception {
// Create an HTTP client
CloseableHttpClient httpClient = HttpClients.createDefault();
// Build the POST request
RequestBuilder builder = RequestBuilder.post("http://example.com/api/endpoint");
StringEntity entity = new StringEntity("{"name":"John","age":30}", "UTF-8");
// Set the request content type to JSON
builder.setEntity(entity);
builder.addHeader("Content-Type", "application/json; charset=utf-8");
// Send the POST request and get the response
HttpResponse response = builder.build().execute(httpClient);
// Read the response
int status = response.getStatusLine().getStatusCode();
if (status == 200) {
System.out.println("POST request sent successfully!");
} else {
System.out.println("Error: " + status);
}
}
}
This code sends a POST request to http://example.com/api/endpoint
with a JSON body that contains two key-value pairs: "name"
with value "John"
, and "age"
with value 30
. The response is then printed out.
You can adjust the URL, JSON body, and headers according to your specific requirements.