How to avoid concurrent modification exception in Java ArrayList?
How to avoid concurrent modification exception in Java ArrayList?
I'm happy to help!
The infamous Concurrent Modification Exception (CME) in Java! It's a common issue that can occur when multiple threads try to modify an ArrayList
concurrently, leading to unpredictable behavior and potential crashes.
To avoid this exception, you need to synchronize access to the ArrayList
when multiple threads are modifying it. Here are some strategies:
synchronized
keyword on a block of code or a method that modifies the ArrayList
. This ensures that only one thread can execute the critical section at a time.
public void modifyList() {
synchronized (list) {
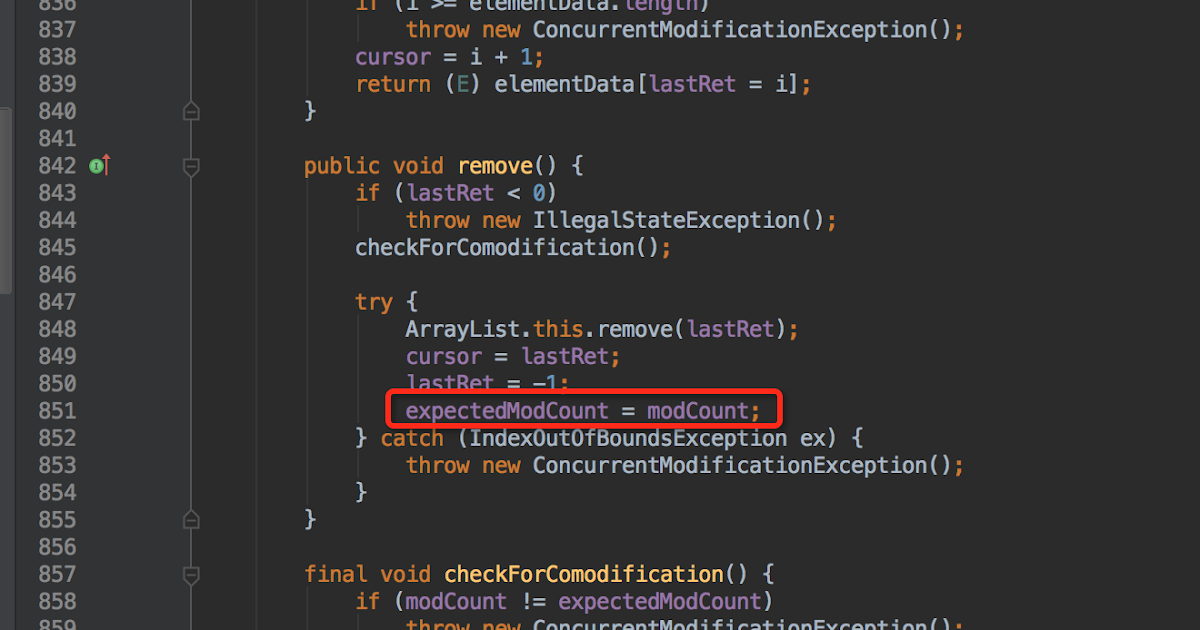
// perform modifications here
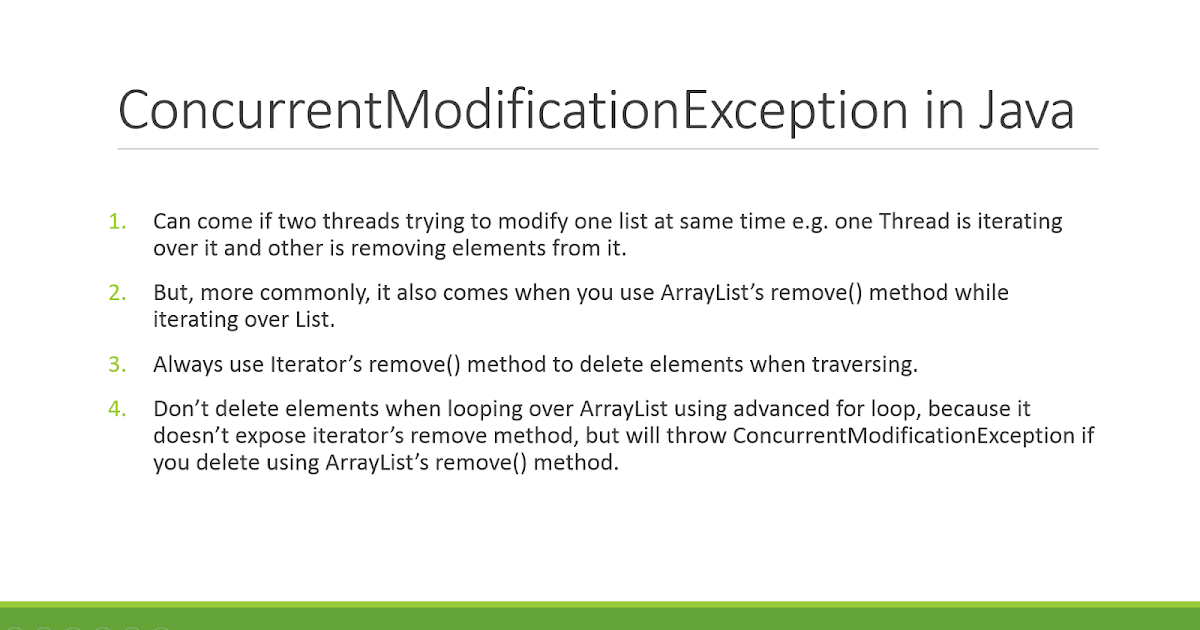
}
}
Use CopyOnWriteArrayList: Java provides a special implementation of ArrayList
called CopyOnWriteArrayList
. It's designed for use in multithreaded environments and uses a copy-on-write approach to avoid CMEs.
List list = new CopyOnWriteArrayList<>();
list.add("new element"); // no need to synchronize!
Use Collections.synchronized()
: You can wrap your ArrayList
with the Collections.synchronized()
method, which adds synchronization for you:
List list = Collections.synchronizedList(new ArrayList<>());
list.add("new element"); // now synchronized!
Use a thread-safe collection class: Instead of using ArrayList
, consider using a thread-safe collection class like CopyOnWriteArrayList
or ConcurrentLinkedQueue
.
Remember, when working with multithreaded code, it's essential to ensure that your data structures are properly synchronized or use thread-safe implementations.
Why CMEs occur:
When multiple threads try to modify an ArrayList
, Java doesn't have built-in support for concurrent modification. If one thread is in the process of modifying the list (e.g., adding or removing elements) and another thread tries to access or modify the same list, chaos ensues!
Consequences of CMEs:
If left unchecked, CMEs can lead to:
Unpredictable behavior: Your code may behave erratically, with unexpected results or exceptions. Deadlocks: Threads may block each other, causing your application to hang or become unresponsive. Data corruption: The integrity of your data structure may be compromised, leading to errors or incorrect results.By using the strategies outlined above, you can avoid Concurrent Modification Exceptions and ensure that your Java code runs smoothly and predictably in a multithreaded environment.
Java concurrent linked list
I'd be happy to discuss Java's Concurrent Linked List!
Java has several data structures designed for multi-threaded environments, which are crucial for scalable and efficient applications. The ConcurrentLinkedQueue
is one such structure that allows multiple threads to access the same queue without locking. But what about a linked list? Well, Java 1.7 introduced a new class called ConcurrentLinkedDeque
, which is a doubly-linked data structure optimized for concurrent access.
Here's how it works: each node in the deque has two references to its predecessor and successor nodes. This allows for efficient insertion and deletion at either end of the deque. The ConcurrentLinkedDeque
uses a combination of lock-free algorithms and atomic operations to ensure thread-safe updates, even in the presence of concurrent modifications.
Let's take a closer look at the key features:
Thread safety: The deque is designed with thread safety in mind. It uses a combination of volatile fields and atomic operations to ensure that the state of the deque remains consistent across all threads.Here's a simple example of how to use ConcurrentLinkedDeque
:
public class ConcurrentLinkedListExample {
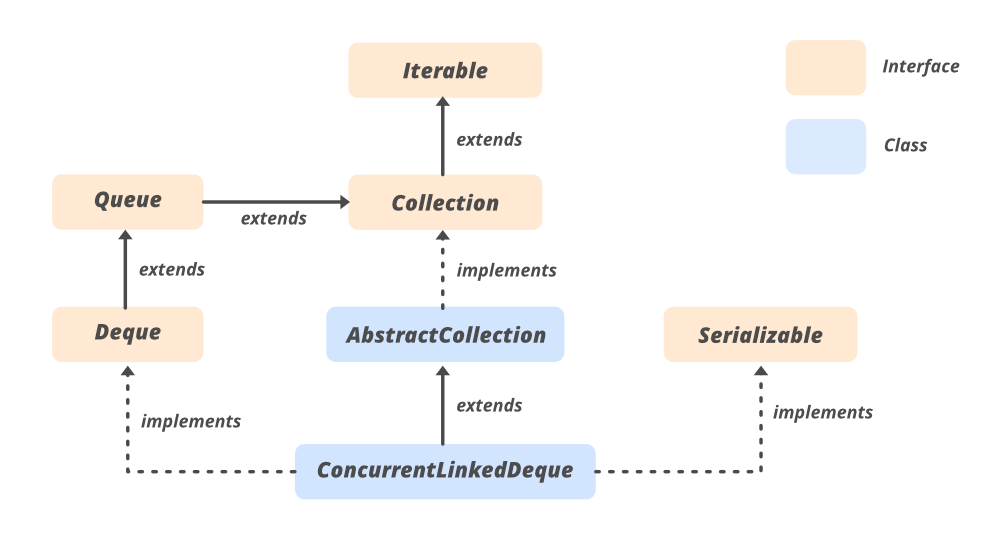
public static void main(String[] args) throws InterruptedException {
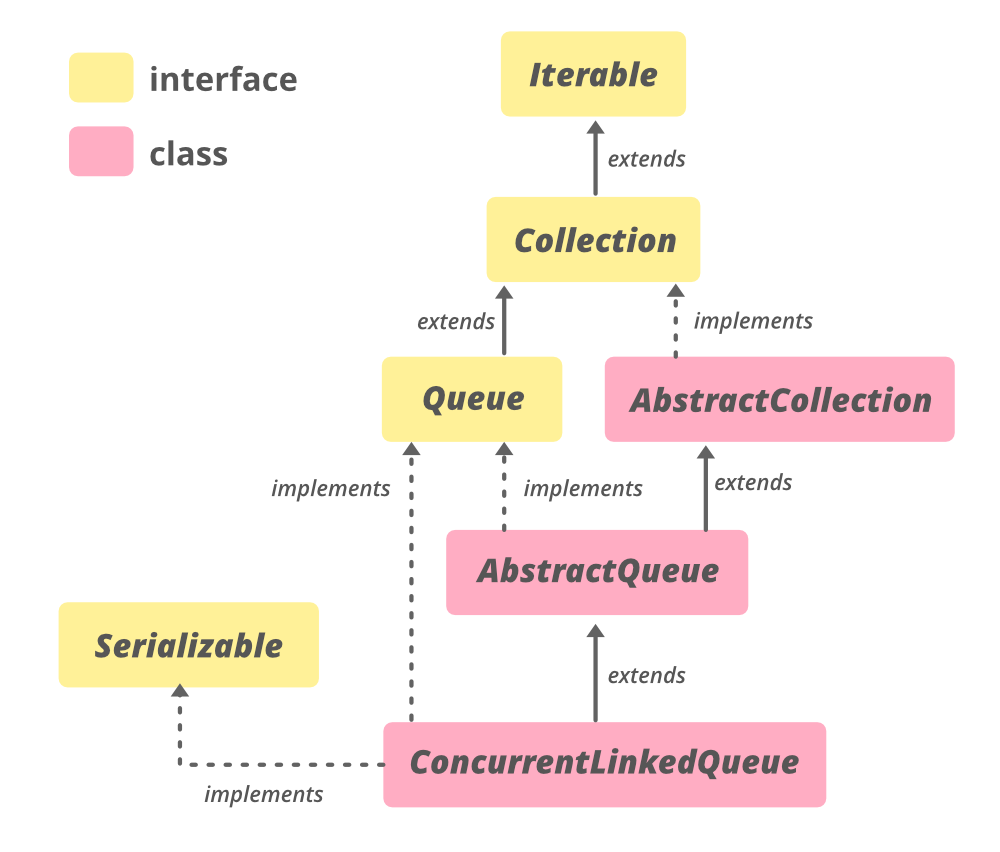
// Create the queue
ConcurrentLinkedDeque queue = new ConcurrentLinkedDeque<>();
// Add some elements
for (int i = 0; i < 10; i++) {
queue.add(i);
Thread.sleep(100); // simulate concurrent access
}
// Iterate over the elements
for (Integer element : queue) {
System.out.println(element);
}
}
}
In this example, we create a ConcurrentLinkedDeque
and add 10 elements to it. We then iterate over the elements using an iterator.
When should you use ConcurrentLinkedDeque
? Here are some scenarios:
ConcurrentLinkedDeque
provides a scalable solution. Message queues: This class is particularly useful for implementing message queues in distributed systems or messaging frameworks. Producer-consumer scenarios: Where one thread produces elements (e.g., messages) and another thread consumes them, this deque can efficiently handle the coordination between producers and consumers.
In conclusion, ConcurrentLinkedDeque
is a powerful tool for building scalable and efficient concurrent data structures in Java. It provides thread safety, lock-free operations, and efficient iteration, making it an excellent choice for high-concurrency applications.