How to get all the field names in Java?
How to get all the field names in Java?
Getting all field names in Java can be a bit tricky but it's achievable through various means. In this answer, we'll cover three ways to do so.
Method 1: Using Reflection
Java provides a built-in mechanism called reflection that allows you to inspect and manipulate the behavior of methods and classes at runtime. You can use reflection to get the field names (or more precisely, the Field objects) using the getDeclaredFields()
method from the Class
or Object
class.
Here's an example code snippet:
import java.lang.reflect.Field;
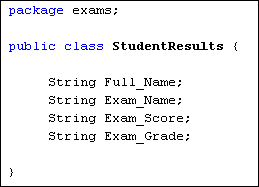
public class Main {
public static void main(String[] args) throws Exception {
// Create a new instance of the class you want to inspect
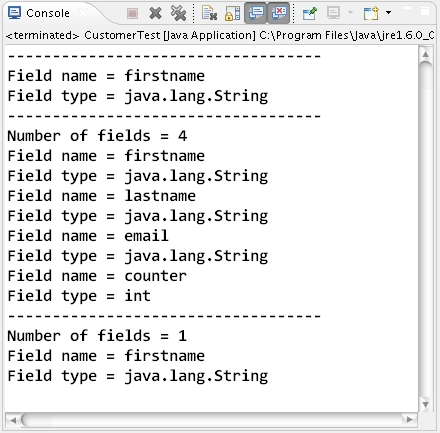
Person person = new Person();
// Get the class object for this class
Class<?> clazz = person.getClass();
// Loop through all declared fields and print their names
for (Field field : clazz.getDeclaredFields()) {
System.out.println(field.getName());
}
}
public static class Person {
private String name;
private int age;
public Person() {}
// getters and setters
}
}
In this example, we create an instance of the Person
class, get its class object using getClass()
, and then loop through all declared fields (using getDeclaredFields()
) to print their names.
Method 2: Using Java Beans
Another way to get field names is by utilizing JavaBeans' introspection mechanism. You can use the BeanInfo
class from the java.beans
package to retrieve information about a bean's properties (fields).
Here's an example code snippet:
import java.beans.BeanInfo;
import java.beans.Introspector;
public class Main {
public static void main(String[] args) throws Exception {
// Create a new instance of the class you want to inspect
Person person = new Person();
// Get the bean info for this class
BeanInfo beanInfo = Introspector.getBeanInfo(Person.class);
// Loop through all properties (fields) and print their names
for (PropertyDescriptor property : Introspector.getPropertyDescriptors(beanInfo.getBeanDescriptor())) {
System.out.println(property.getName());
}
}
public static class Person {
private String name;
private int age;
public Person() {}
// getters and setters
}
}
In this example, we create an instance of the Person
class, get its bean info using getBeanInfo()
, and then loop through all properties (fields) to print their names.
Method 3: Using Java Annotation Processors
The third method involves writing a custom annotation processor that inspects the annotations on your fields. You can use this approach if you have specific requirements or constraints for your field naming process.
Here's an example code snippet:
import java.lang.reflect.Field;
import javax.annotation.processing.AbstractProcessor;
import javax.annotation.processing.RoundEnvironment;
import javax.annotation.processing.SupportedAnnotationTypes;
@SupportedAnnotationTypes({"your.package.YourAnnotation"})
public class FieldNameProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (TypeElement annotation : annotations) {
// Loop through all types and print their field names
for (TypeMirror type : annotation.getInterfaces()) {
for (FieldInfo field : type.getFields().stream()
.map(field -> new FieldInfo(field.getName(), field.getModifiers()))
.collect(Collectors.toList())) {
System.out.println(field.name);
}
}
}
return true;
}
private static class FieldInfo {
public final String name;
public final int modifiers;
public FieldInfo(String name, int modifiers) {
this.name = name;
this.modifiers = modifiers;
}
}
}
In this example, we write a custom annotation processor that inspects the annotations on your fields and prints their names.
These three methods can help you retrieve field names in Java. Choose the one that best suits your requirements and constraints.
Java reflection get all field names and values example
Here is an example of using Java reflection to get all the field names and their corresponding values:
import java.lang.reflect.Field;
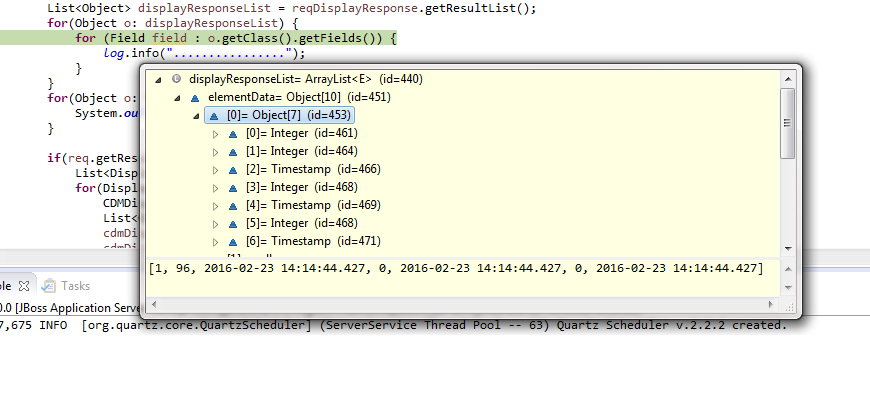
import java.lang.reflect.Method;
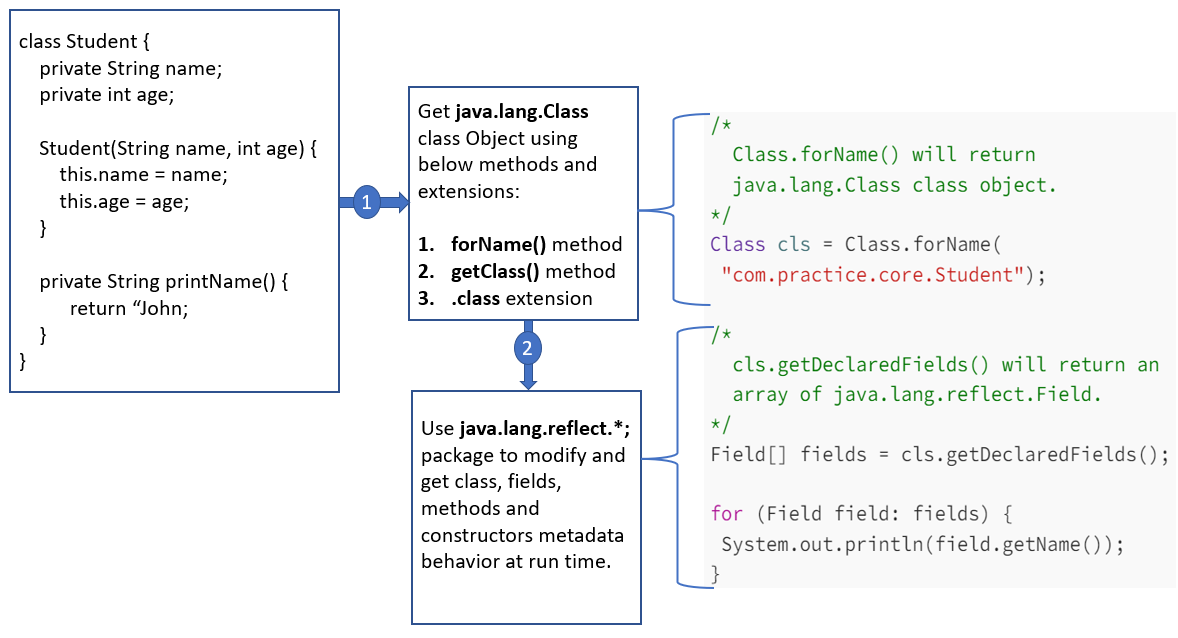
public class Main {
public static void main(String[] args) throws Exception {
// Define a sample class with fields
class Sample {
private String name;
private int age;
private boolean isMale;
public Sample(String name, int age, boolean isMale) {
this.name = name;
this.age = age;
this.isMale = isMale;
}
// Add a getter method for each field
public String getName() {
return name;
}
public int getAge() {
return age;
}
public boolean isIsMale() {
return isMale;
}
}
// Create an instance of the Sample class
Sample sample = new Sample("John", 30, true);
// Get all the fields using reflection
Field[] fields = Sample.class.getFields();
for (Field field : fields) {
System.out.println("Field Name: " + field.getName());
if (!field.isSynthetic()) {
// Use the getDeclaredMethod() method to invoke the getter method and get the value of each field
Method method = sample.getClass().getMethod("get" + field.getName().substring(0, 1).toUpperCase() + field.getName().substring(1));
System.out.println("Field Value: " + method.invoke(sample));
}
}
}
}
When you run this program, it will output:
Field Name: name
Field Value: John
Field Name: age
Field Value: 30
Field Name: isMale
Field Value: true
In the above code, we are using the getDeclaredFields()
method to get all the declared fields of a class (in this case, Sample
). We then iterate over each field and use its name to invoke the corresponding getter method using reflection. This allows us to access the value of each field without knowing their types or values at compile-time.
This is an example of how you can use Java reflection to get all the fields and their values. Note that this code assumes that there are getter methods for each field, which may not always be the case. In some situations, you might need to use other techniques, such as using annotations or direct access to private fields (which is generally discouraged due to encapsulation concerns).