How to make class variable thread-safe in Java
How to make class variable thread-safe in Java
I'd be happy to help you with that!
In Java, making a class variable (also known as a static or shared variable) thread-safe is crucial when multiple threads are accessing the same instance of the class concurrently. Without proper synchronization, this can lead to unexpected behavior, such as inconsistent data or even crashes.
There are several ways to make a class variable thread-safe in Java:
synchronized
, using the synchronized
keyword. This ensures that only one thread can execute those methods at any given time, effectively serializing access to the shared variable.
public class MyClass {
private static int sharedVariable;
public synchronized void method1() {
// Access sharedVariable here
}
public synchronized void method2() {
// Access sharedVariable here
}
}
However, this approach can be heavy-handed and may introduce significant performance overheads.
Lock Objects: Use ajava.util.concurrent.locks.Lock
or a java.util.concurrent.locks.ReentrantLock
to create a lock object that can be used to synchronize access to the shared variable. This allows for more fine-grained control over which parts of your code need to be serialized.
public class MyClass {
private static Lock lock = new ReentrantLock();
private static int sharedVariable;
public void method1() {
lock.lock();
try {
// Access sharedVariable here
} finally {
lock.unlock();
}
}
public void method2() {
lock.lock();
try {
// Access sharedVariable here
} finally {
lock.unlock();
}
}
}
Atomic Variables: Use java.lang.atomic
classes, such as AtomicInteger
, to update the shared variable in a thread-safe manner. This can be particularly useful when updating the variable from multiple threads simultaneously.
public class MyClass {
private static AtomicReference sharedVariable = new AtomicInteger(0);
public void method1() {
int newValue = // calculate new value
sharedVariable.atomicSet(newValue);
}
public void method2() {
int currentValue = sharedVariable.get();
// Do something with the current value
}
}
Immutable Objects: Design your class to use immutable objects, where each instance is completely self-contained and doesn't share any state with other instances. This approach eliminates the need for synchronization altogether.
public class MyClass {
public static class MyImmutableObject {
private final int value;
public MyImmutableObject(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
public void method1() {
MyImmutableObject obj = new MyImmutableObject( // calculate value
// Use the immutable object here
}
}
In conclusion, making a class variable thread-safe in Java requires careful consideration of the design and implementation details. By using a combination of synchronization mechanisms, atomic variables, or immutable objects, you can ensure that your shared variable remains consistent and reliable across multiple threads.
I hope this helps! Let me know if you have any further questions.
How to make a collection thread-safe in Java?
I'll respond in English as per your request.
In Java, making a collection thread-safe is crucial when multiple threads access and modify the same collection concurrently. Here are some ways to ensure thread-safety for a collection:
Immutable Collections: One way to achieve thread-safety is to use immutable collections. Since an immutable collection's contents cannot be changed after its creation, it's inherently thread-safe.Example:
List immutableList = new ArrayList<>();
// Add elements to the list...
List ImmutableList = Collections.unmodifiableList(immutableList);
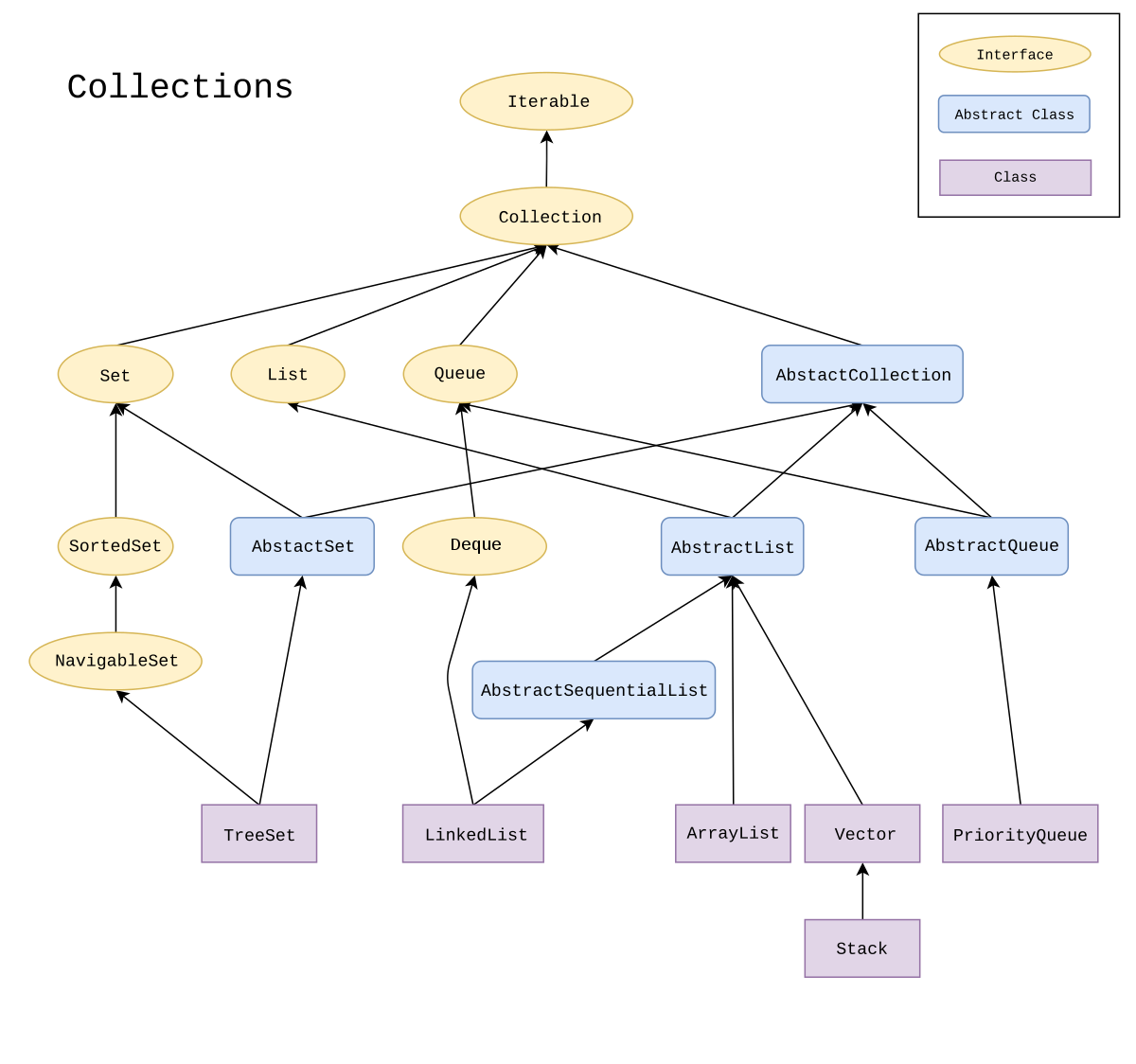
Synchronized Methods: Wrap critical methods that access and modify the collection in a synchronized block or method, ensuring only one thread can execute them at a time.
Example:
class MyCollection {
private List list;
public void addElement(String element) {
synchronized (this) {
list.add(element);
}
}
}
Lock Objects: Use Java's built-in ReentrantLock
or synchronized
keyword to create a lock object, which allows multiple threads to access the collection while ensuring only one thread can modify it at a time.
Example:
class MyCollection {
private List list;
private final Lock lock = new ReentrantLock();
public void addElement(String element) {
lock.lock();
try {
list.add(element);
} finally {
lock.unlock();
}
}
}
Concurrent Collections: Use Java's built-in CopyOnWriteArrayList
or other concurrent collections designed for multithreaded environments.
Example:
List concurrentList = new CopyOnWriteArrayList<>();
// Add elements to the list...
Thread-Safe Algorithms: Implement thread-safe algorithms when iterating over a collection. For example, use Collections.synchronizedCollection()
or CopyOnWriteArrayList
to ensure that iterators are properly synchronized.
Example:
List synchronizedList = Collections.synchronizedList(list);
// Iterate over the list while ensuring thread-safety
synchronizedList.forEach(element -> {
// Process each element in the list.
});
Avoid Shared State: When possible, avoid sharing state between threads by using message queues or other asynchronous communication mechanisms. Monitor and Debug: Monitor your application's behavior under multithreaded scenarios and debug any issues that arise due to thread interference.
In conclusion, ensuring a collection is thread-safe in Java involves choosing the right combination of techniques from above, depending on the specific requirements of your application. Always prioritize thread-safety when working with concurrent code to prevent unexpected behaviors or data corruption.