What is an abstract method in Java with an example?
What is an abstract method in Java with an example?
In Java, an abstract method is a method that has a declaration but does not have an implementation. It is a method that must be implemented by any non-abstract subclass of the class that declares it. Abstract methods are declared using the abstract
keyword and do not have a body.
Here's an example:
public abstract class Animal {
public abstract void makeSound();
}
public class Dog extends Animal {
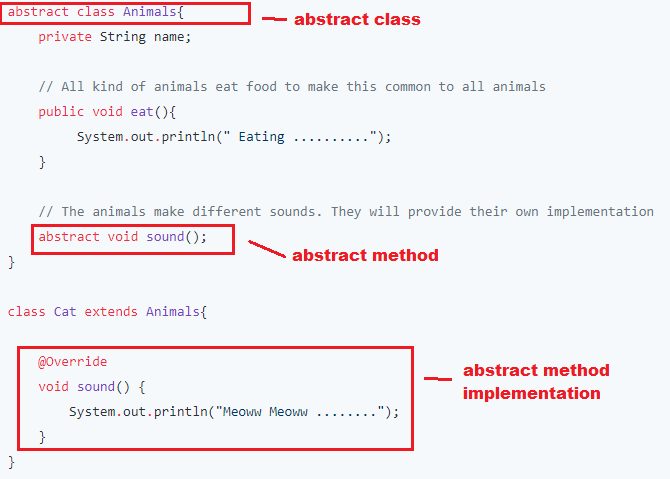
@Override
public void makeSound() {
System.out.println("The dog goes woof!");
}
}
public class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("The cat meows!");
}
}
In this example, Animal
is an abstract class with an abstract method makeSound()
. The Dog
and Cat
classes are subclasses of Animal
and must implement the makeSound()
method.
When you create a new instance of Dog
or Cat
, you can call the makeSound()
method:
public static void main(String[] args) {
Dog dog = new Dog();
Cat cat = new Cat();
dog.makeSound(); // The dog goes woof!
cat.makeSound(); // The cat meows!
}
This demonstrates how abstract methods provide a way for you to define an interface (or contract) that must be implemented by any class that inherits from the original class. This is useful when you want to ensure that all subclasses implement specific behavior.
Abstract methods are not the same as interface methods. Interface methods can have default implementations, whereas abstract methods do not have an implementation at all. Abstract classes and interfaces both provide a way to define an interface for a family of related classes, but they do it in different ways.
In this example, we use an abstract class to achieve polymorphism (the ability to treat objects of different classes as if they were of the same class). This is useful when you want to group related classes together and provide a way to share behavior between those classes.
Java abstraction tutorial w3schools
Here is a comprehensive guide to Java abstract classes and methods on W3Schools:
Java Abstract Classes
In Java, an abstract class is a class that cannot be instantiated and is meant to be inherited by other classes. It can have both abstract and concrete methods.
To declare an abstract class, you use the abstract
keyword before the class name:
public abstract class Animal {
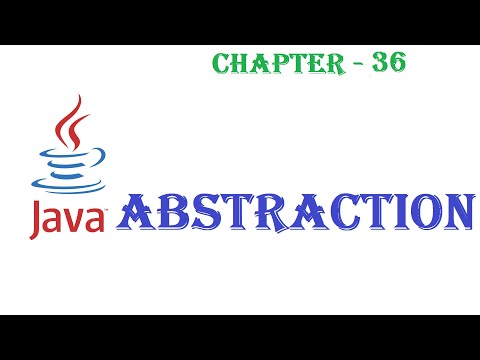
// ...
}
Abstract classes are often used as base classes for a group of related classes that share common attributes and methods.
Java Abstract Methods
An abstract method is a method declared in an abstract class that must be implemented by any non-abstract subclass. It does not have a body and can only be called from within the same class or its subclasses.
To declare an abstract method, you use the abstract
keyword before the method name:
public abstract class Animal {
public abstract void sound();
}
Any non-abstract subclass of Animal
must implement the sound()
method.
Creating a Concrete Subclass
A concrete subclass is a subclass that provides implementations for all the abstract methods declared in its superclass. Here's an example of a concrete subclass:
public class Dog extends Animal {
public void sound() {
System.out.println("Woof!");
}
}
In this example, Dog
is a concrete subclass of Animal
. It implements the sound()
method by printing "Woof!" to the console.
Using an Abstract Class
To use an abstract class, you create an instance of one of its concrete subclasses. Here's an example:
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Dog();
myAnimal.sound(); // Output: Woof!
}
}
In this example, we create a Dog
object and assign it to a variable of type Animal
. We can then call the sound()
method on that variable.
Benefits of Abstract Classes
Abstract classes provide several benefits:
Encapsulation: They encapsulate common attributes and methods for a group of related classes. Inheritance: They allow you to inherit behavior from a superclass. Polymorphism: They enable polymorphic behavior by providing multiple implementations of an interface.Conclusion
Java abstract classes and methods are powerful tools that can help you create more flexible and reusable code. By using abstract classes, you can encapsulate common attributes and methods for a group of related classes, and provide a way to inherit behavior from a superclass.
I hope this tutorial has helped you understand how to use Java abstract classes and methods. If you have any questions or need further clarification, feel free to ask!