Java generics question mark example
Java generics question mark example
I'm happy to help with your question about Java generics!
Java generics were introduced in Java 5 (also known as Java 1.5) and have since become a fundamental part of the language. Generics allow you to define reusable classes, interfaces, and methods that can work with any type of object, not just objects of the same class or interface.
Let's start with an example. Suppose we want to write a simple Pair
class that holds two elements of different types:
public class Pair {
private T1 first;
private T2 second;
public Pair(T1 first, T2 second) {
this.first = first;
this.second = second;
}
public T1 getFirst() {
return first;
}
public T2 getSecond() {
return second;
}
}
In this example, we're using a type parameter T1
and another type parameter T2
. These type parameters represent the types of the two elements in our Pair
class. When we create an instance of Pair
, we need to specify the actual types for these type parameters.
Here's an example of how we might use this Pair
class:
public static void main(String[] args) {
Pair<String, Integer> pair1 = new Pair<>("Hello", 42);
System.out.println("First: " + pair1.getFirst());
System.out.println("Second: " + pair1.getSecond());
Pair<Integer, Double> pair2 = new Pair<>(10, 3.14);
System.out.println("First: " + pair2.getFirst());
System.out.println("Second: " + pair2.getSecond());
}
In this example, we're creating two instances of Pair
: one for holding a string and an integer, and another for holding an integer and a double. The type parameters are inferred from the types we pass to the constructor.
Now, let's talk about the importance of using generics in Java. One key benefit is that it allows you to create code that is more flexible and reusable. Without generics, you would need to write separate classes or interfaces for each specific combination of types. With generics, you can write a single class or interface that can work with any type of object.
For example, if we wanted to write a List
class without generics, we might do something like this:
public class MyArrayList {
private Object[] elements;
public MyArrayList(int size) {
elements = new Object[size];
}
public void add(Object obj) {
// implementation elided...
}
public Object get(int index) {
return elements[index];
}
}
This would work, but it's a bit cumbersome. With generics, we can write a List
class that is more flexible and reusable:
public class MyArrayList {
private T[] elements;
public MyArrayList(int size) {
elements = (T[]) new Object[size];
}
public void add(T obj) {
// implementation elied...
}
public T get(int index) {
return elements[index];
}
}
In this example, we're using a type parameter T
to represent the type of the objects in our List
. This allows us to create instances of MyArrayList
that can hold any type of object.
I hope this helps! Let me know if you have any further questions.
What does a question mark (?) Designate in Java?
I'd be happy to help you with that!
In Java, the question mark (?) is not used as a designator for anything specific. It's actually part of the syntax for catching exceptions.
When using try-catch blocks in Java, the question mark is used along with the catch keyword to specify the type of exception to be caught. The general syntax is:
try {
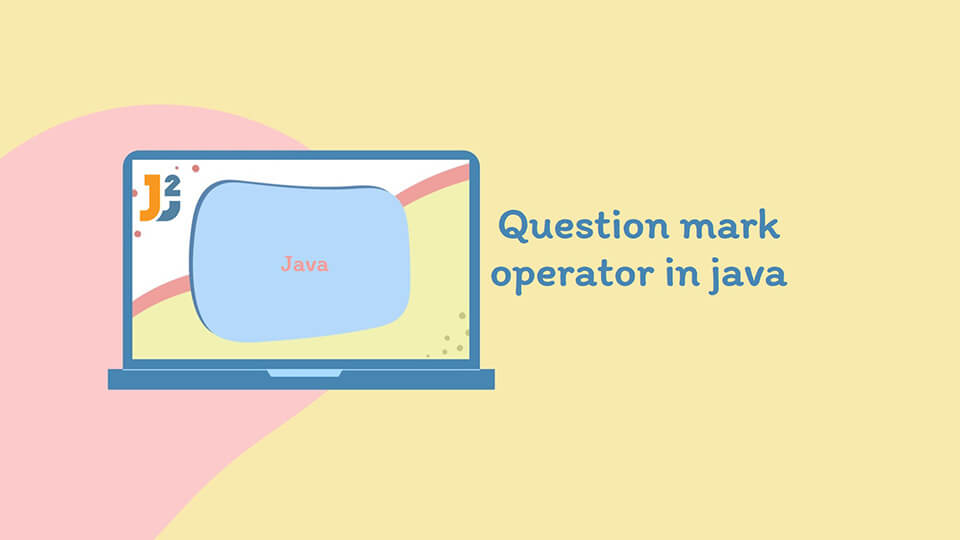
// code that may throw an exception
} catch (ExceptionType ? e) {
// code to handle the exception
}
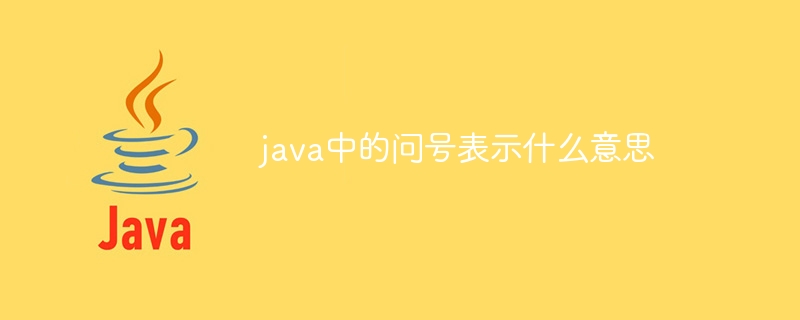
In this context, the question mark is not actually part of the exception type; it's more like a wildcard character indicating that any exception of the specified type (or its subclass) should be caught.
This syntax was introduced in Java 7 as part of the try-catch improvement features. Prior to Java 7, you would typically catch Exception and then use instanceof or isInstance() to check if the caught exception is an instance of a specific exception type.
Here's an example:
try {
// code that may throw a NullPointerException
} catch (NullPointerException ? e) {
System.out.println("A null pointer exception was caught!");
}
In this case, the question mark indicates that any NullPointerException should be caught and handled by the corresponding code block.