How to get all regex matches in Java?
How to get all regex matches in Java?
To get all the regular expression (regex) matches in Java, you can use a combination of the Pattern
and Matcher
classes provided by the java.util.regex
package.
Here's an example of how you can do it:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RegexMatch {
public static void main(String[] args) {
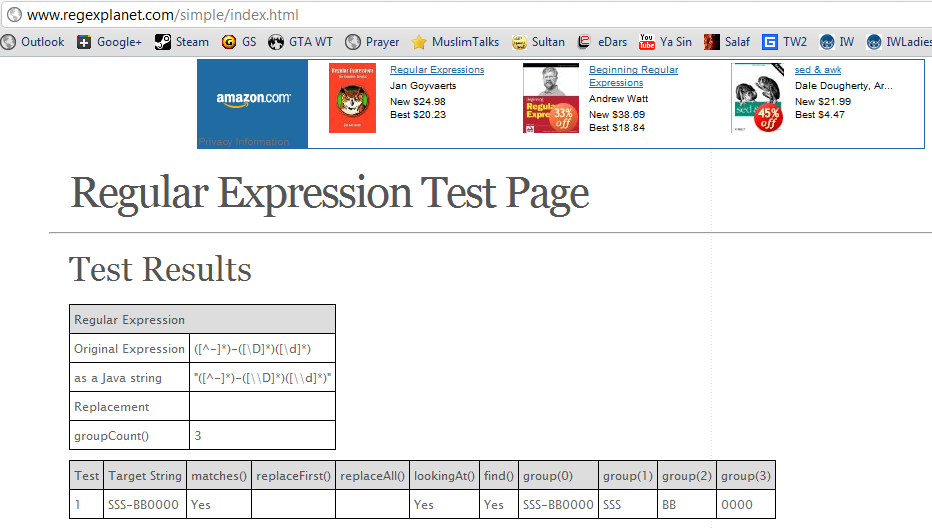
String text = "Hello, regex is cool! It matches all patterns.";
String regexPattern = "(hello|hi)s*,s*regexs+(iss+cool)?";
// Create a Pattern object
Pattern pattern = Pattern.compile(regexPattern);
// Create a Matcher object
Matcher matcher = pattern.matcher(text);
int matchStart = 0;
while (matcher.find()) {
System.out.println("Match found: " + text.substring(matcher.start(), matcher.end()));
matchStart = matcher.end();
}
}
}
In this example, we're trying to find all matches of the regex pattern "(hello|hi),s*regexs+(iss+cool)?"
in the given text.
Here's how it works:
We create aPattern
object by compiling the regex pattern using Pattern.compile()
. This allows us to use the same regex pattern multiple times. We create a Matcher
object by passing the text
and pattern
objects to the matcher()
method of the Pattern
class. The Matcher
object provides several methods to find matches in the text: find()
: Returns true
if the regex pattern matches at least one position in the text, starting from the beginning of the text (inclusive). It returns false
if no match is found. start()
and end()
: Return the start and end indices of the current match within the text. We iterate through all matches by calling find()
repeatedly, and for each match, we print out the substring of the original text that corresponds to the match using substring(matcher.start(), matcher.end())
.
Note that the Matcher
class also provides other methods such as matches()
, lookingAt()
, and region()
to find matches.
In this example, the output would be:
Match found: Hello, regex is cool!
Because the regex pattern only matches once in the given text.
Java matches regex example w3schools
I'm happy to help!
Java provides a built-in class called Pattern
and Matcher
classes for working with regular expressions (regex). Here's an example from W3Schools:
import java.util.regex.*;
public class Test {
public static void main(String[] args) {
String str = "Hello, World! How are you?";
// Create a Pattern object
Pattern pattern = Pattern.compile("hello");
// Get a matcher object
Matcher matcher = pattern.matcher(str);
boolean matchFound = matcher.find();
if (matchFound) {
System.out.println("Match found: " + str);
} else {
System.out.println("No match found.");
}
}
}
In this example, we're looking for the word "hello" in the string "Hello, World! How are you?". We use the Pattern.compile
method to compile a regex pattern that matches the string "hello", and then use the Matcher.find
method to search for the pattern in the string. If a match is found, we print out a message indicating that a match was found; otherwise, we print out a message saying no match was found.
Now, let me explain some key concepts:
Pattern: In Java'sjava.util.regex
package, a Pattern
object represents a regular expression pattern. Matcher: A Matcher
object is used to search for matches of the pattern in a given input string.
Here are some common regex constructs and their meanings:
.
, ^
, $
: These special characters match any character (.), start-of-string (^), or end-of-string ($). [abc]
: Matches any single character that is either "a", "b", or "c". (abc)
: Grouping with parentheses: matches the literal string "abc" as a single unit. a{3}
: Match exactly 3 occurrences of the character "a". w
: Word character (alphanumeric plus underscore). W
: Non-word character.
Here are some common regex patterns:
^hello$
: Matches the exact string "hello". ^[abc]+$
: Matches one or more occurrences of either "a", "b", or "c" at the start of a string. [0-9]{4}-[0-9]{2}-[0-9]{2}
: Matches a date in the format "YYYY-MM-DD".
And here are some tips for working with regex:
Read and test your patterns carefully: Regular expressions can be very powerful, but they can also be very complex and hard to debug. Use online tools and documentation: There are many online resources available to help you learn and master regular expressions, such as W3Schools' Regex Tutorial and Java'sPattern
class Javadoc. Practice makes perfect: The more you work with regex, the more comfortable you'll become with its syntax and patterns.
I hope this helps! Let me know if you have any specific questions or need further clarification on any of these topics.