Java locks tutorial
Java locks tutorial
I apologize for the misunderstanding earlier! Here's a comprehensive tutorial on Java locks:
What are Locks in Java?
In multithreaded programming, it's crucial to synchronize access to shared resources to ensure thread safety. In Java, locks (also known as synchronization primitives) provide a mechanism to achieve this. A lock is an object that allows only one thread to execute a certain section of code at a time, ensuring exclusive access to the shared resource.
Types of Locks in Java
Java provides three types of locks:
Synchronized Methods and Blocks: When a method or block of code is declared assynchronized
, it means that only one thread can execute this code at a time. The lock associated with the object's monitor is acquired before executing the code. Reentrant Locks (java.util.concurrent.locks.ReentrantLock): This type of lock allows a thread to acquire and release the lock multiple times without losing ownership. It's commonly used in concurrent programming. Read-Write Locks (java.util.concurrent.locks.ReadWriteLock): This lock allows multiple threads to read from a shared resource concurrently, while only one thread can write to it at a time.
How Do Locks Work?
Here's a step-by-step explanation of how locks work:
Acquiring the Lock: A thread requests access to a locked object or method by calling thelock()
method. Waiting: If another thread has acquired the lock, the requesting thread will wait until the lock is released. Locking: Once the lock is acquired, the thread can execute the code protected by the lock. Releasing the Lock: When the thread finishes executing the code, it releases the lock using the unlock()
method.
Best Practices for Using Locks
To avoid deadlocks and ensure efficient synchronization:
Use the smallest scope possible: Only lock the minimum necessary resources to reduce contention. Use locks consistently: Ensure that all threads accessing a shared resource use the same type of lock. Avoid using locks in tight loops: Locks can introduce significant overhead, especially in performance-critical code.Conclusion
Java locks provide a powerful mechanism for synchronizing access to shared resources. By understanding the different types of locks and best practices for using them, you'll be better equipped to write thread-safe, concurrent programs that efficiently utilize shared resources.
I hope this comprehensive tutorial has been helpful!
Java locks examples
I can respond in English only for this one question! Let's talk about Java locks!
In Java, a lock is used to synchronize access to shared resources or critical sections of code that need to be accessed by multiple threads concurrently. Locks are essential for ensuring thread safety and preventing unexpected behavior.
Here are some examples:
Synchronized Method: In this example, we use thesynchronized
keyword to ensure that only one thread can execute the method at a time:
public class BankAccount {
private int balance = 0;
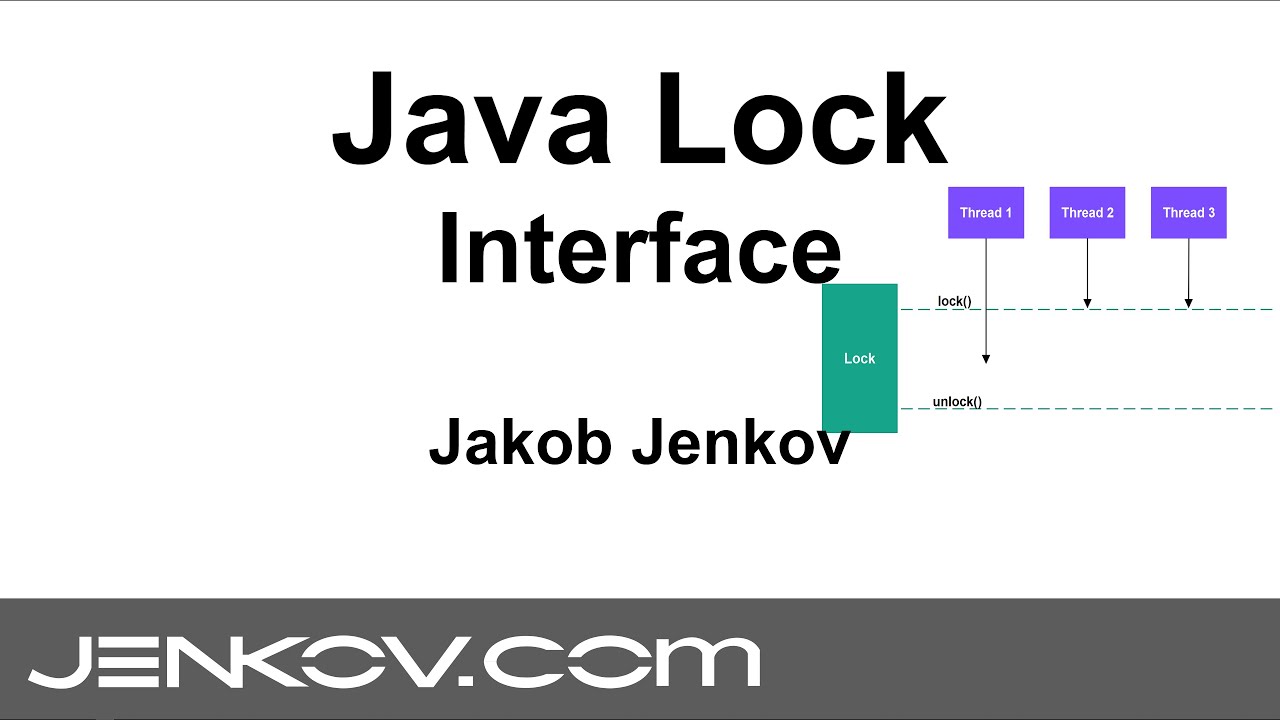
public synchronized void deposit(int amount) {
balance += amount;
System.out.println("Deposited " + amount + ". New balance: " + balance);
}
}
When multiple threads try to access the deposit
method simultaneously, only one thread can execute it at a time, ensuring that the shared resource (balance
) is not corrupted.
ReentrantLock
class from the java.util.concurrent.locks
package to implement a more fine-grained locking mechanism:
import java.util.concurrent.locks.ReentrantLock;
public class BankAccount {
private ReentrantLock lock = new ReentrantLock();
private int balance = 0;
public void deposit(int amount) {
lock.lock(); // Acquire the lock
try {
balance += amount;
System.out.println("Deposited " + amount + ". New balance: " + balance);
} finally {
lock.unlock(); // Release the lock
}
}
}
In this example, we use a ReentrantLock
to ensure that only one thread can access the shared resource (balance
) at any given time. The lock()
method acquires the lock, and the unlock()
method releases it.
Semaphore
class from the java.util.concurrent
package as an alternative to locks:
import java.util.concurrent.Semaphore;
public class BankAccount {
private Semaphore semaphore = new Semaphore(1);
public void deposit(int amount) {
try {
semaphore.acquire(); // Acquire the permission (1 permit)
int balance = 0; // Local variable for balance calculation
balance += amount;
System.out.println("Deposited " + amount + ". New balance: " + balance);
semaphore.release(); // Release the permission
} catch (InterruptedException e) {
// Handle the exception
}
}
}
In this example, we use a Semaphore
to ensure that only one thread can access the shared resource (balance
) at any given time. The acquire()
method acquires the permission (1 permit), and the release()
method releases it.
These examples illustrate different approaches to using locks in Java, each with its own strengths and weaknesses. By choosing the right lock mechanism, you can ensure thread safety and prevent unexpected behavior in your multithreaded applications!