Which inheritance cannot be used in java with example
Which inheritance cannot be used in java with example
I'd be happy to help!
In Java, there are two types of inheritance: Single Inheritance and Multilevel Inheritance. However, one type of inheritance that is not possible in Java is Multiple Inheritance.
Multiple Inheritance is when a subclass inherits properties and behaviors from more than one superclass. For example:
Let's say we have three classes - Animal
, Mammal
, and Reptile
. Mammal
is a subclass of Animal
, and Reptile
is also a subclass of Animal
.
class Animal {
public void eat() {
System.out.println("Eating...");
}
}
class Mammal extends Animal {
public void walk() {
System.out.println("Walking...");
}
}
class Reptile extends Animal {
public void crawl() {
System.out.println("Crawling...");
}
}
Now, if we want to create a class that is both a Mammal
and a Reptile
, we can't do it using inheritance in Java. This is because Java does not support multiple inheritance, i.e., a subclass cannot extend more than one superclass directly.
If you try to compile the following code:
class Hybrid extends Mammal, Reptile {
public void hybridBehavior() {
System.out.println("This is a hybrid behavior...");
}
}
You'll get an error message saying "Hybrid.java:3: error: illegal generic type".
The reason for this limitation is that multiple inheritance can lead to the "Diamond Problem" - which occurs when two classes, A
and B
, both inherit from a third class, C
. If A
and B
both have their own version of a method inherited from C
, then it's unclear which version should be called.
In contrast, Java's single inheritance allows for more predictable and avoidable ambiguity in the inheritance hierarchy.
So, to summarize: In Java, multiple inheritance is not supported because it can lead to ambiguous situations like the "Diamond Problem" that are difficult to handle. Instead, developers use interfaces or composition to achieve similar functionality.
Which inheritance is not used in Java?
I'd be happy to explain!
Inheritance is a fundamental concept in object-oriented programming (OOP), and it's widely used in various programming languages, including Java. However, there are some special cases where inheritance isn't directly applied.
One such case is multiple inheritance. In Java, you can only inherit from a single parent class. This means that if you have two or more interfaces that have the same method signature, you can implement all of them without any issues. However, if you try to extend multiple classes in Java using the extends
keyword, you'll get an error. For example:
class A { }
class B { }
class C extends A, B { } // Error: Multiple inheritance is not allowed in Java
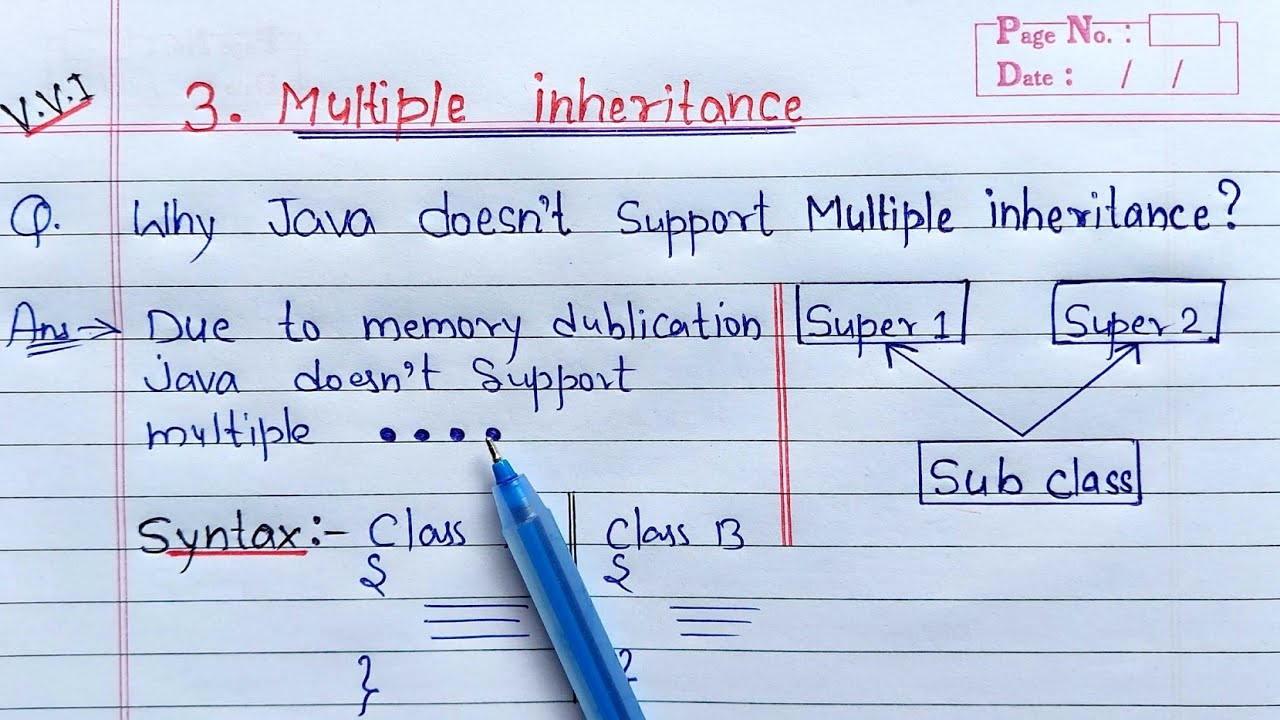
Instead of multiple inheritance, Java provides another mechanism called "interface implementation" to achieve similar results. You can implement multiple interfaces without extending any classes.
Another case where inheritance isn't used directly is when you're working with abstract classes and interfaces. In Java, an abstract class can extend another abstract class, or it can implement one or more interfaces. However, you cannot extend a non-abstract class (also known as a concrete class). For example:
abstract class A { } // Abstract class
class B extends Object { } // Concrete class
abstract class C extends A, B { } // Error: Cannot extend a concrete class
To overcome this limitation, you can use composition instead of inheritance. This is known as the "HAS-A" relationship, where one class contains or has another class.
Additionally, there are cases where you might not need to explicitly use inheritance in your Java code. For instance:
When working with anonymous classes (inner classes), you're creating a new class that extends another class without declaring it explicitly. When using lambda expressions or method references, you're effectively creating an anonymous class that implements a specific interface.In summary, while Java does support inheritance in certain contexts, there are limitations and alternative mechanisms to achieve similar results. By understanding these nuances, you can write more effective and efficient code in Java.