Read and write XML file in Java
Read and write XML file in Java
Reading and writing XML files in Java is a common task that can be achieved using various libraries and APIs. In this answer, I'll provide an overview of how to read and write XML files in Java.
Reading XML Files in Java
Java provides several ways to read XML files. One popular approach is to use the JAXB (Java Architecture for XML Binding) API, which allows you to marshal XML data into Java objects and vice versa. Here's a simple example:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.JAXBException;
import java.io.File;
import java.io.StringWriter;
import java.io.Writer;
public class ReadXML {
public static void main(String[] args) throws JAXBException {
// Create a JAXB context
JAXBContext jc = JAXBContext.newInstance(Order.class);
// Unmarshal the XML file into an Order object
Unmarshaller um = jc.createUnmarshaller();
File xmlFile = new File("order.xml");
Order order = (Order) um.unmarshal(new FileReader(xmlFile));
// Process the Order object
System.out.println(order.getOrderID());
}
}
Writing XML Files in Java
To write an XML file, you can use a similar approach with JAXB:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
import javax.xml.bind.JAXBException;
import java.io.File;
import java.io.StringWriter;
public class WriteXML {
public static void main(String[] args) throws JAXBException {
// Create a JAXB context
JAXBContext jc = JAXBContext.newInstance(Order.class);
// Marshaling the Order object into XML
Marshaller m = jc.createMarshaller();
ObjectFactory factory = new ObjectFactory();
// Create an Order object
Order order = new Order();
order.setOrderID("1234");
// Marshal the Order object into XML
StringWriter writer = new StringWriter();
m.marshal(new JAXBElement(new QName("order"), Order.class, order), writer);
String xmlString = writer.toString();
// Save the XML to a file
File file = new File("output.xml");
Writer output = new FileWriter(file);
output.write(xmlString);
}
}
Using DOM Parser in Java
Java also provides a DOM (Document Object Model) parser for reading and writing XML files. Here's an example:
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
public class ReadXMLDOM {
public static void main(String[] args) {
// Parse the XML file into a DOM tree
Document doc = javax.xml.parsers.DocumentBuilderFactory.newInstance().newDocumentBuilder().parse(new File("order.xml"));
// Get the root element
Element root = doc.getDocumentElement();
// Process the elements in the XML file
NodeList nodeList = root.getChildNodes();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
System.out.println(node.getTagName());
}
}
}
}
Using StAX API in Java
StAX is another way to read and write XML files. Here's an example:
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLEventWriter;
import javax.xml.stream.XMLEventWrapper;
public class ReadXMLStax {
public static void main(String[] args) throws Exception {
// Parse the XML file using StAX
XMLInputFactory factory = XMLInputFactory.newInstance();
XMLEventReader eventReader = factory.createXMLEventReader(new File("order.xml"));
// Process the events in the XML file
while (eventReader.hasNext()) {
XMLEvent event = eventReader.nextEvent();
if (event.isStartElement()) {
System.out.println(event.asStartElement().getName());
}
}
}
}
These are just a few examples of how to read and write XML files in Java. There are many other ways to do this, depending on your specific requirements and the complexity of your XML files.
References
Oracle: Using JAXB to Marshal and Unmarshal Objects Oracle: Reading and Writing XML with StAX W3C: Document Object Model (DOM) Level 2 Core SpecificationWhat is build XML in Java?
Building an XML file in Java! That's a fascinating topic!
To create an XML file in Java, you can use various libraries and tools. One of the most popular ones is the Java API for XML Processing (JAXP). JAXP provides a way to parse, transform, and validate XML documents.
Here are some general steps to build an XML file using Java:
Choose an XML parser: You have several options for XML parsers in Java, including DOM4J, Xerces, Jaxen, and Apache Xalan. Each has its own strengths and weaknesses. Create a new XML document: Use your chosen XML parser to create a new Document Object Model (DOM) or parse an existing XML file.Here's some sample code using the DOM4J library:
import org.dom4j.Document;
import org.dom4j.Element;
import org.dom4j.io.OutputFormat;
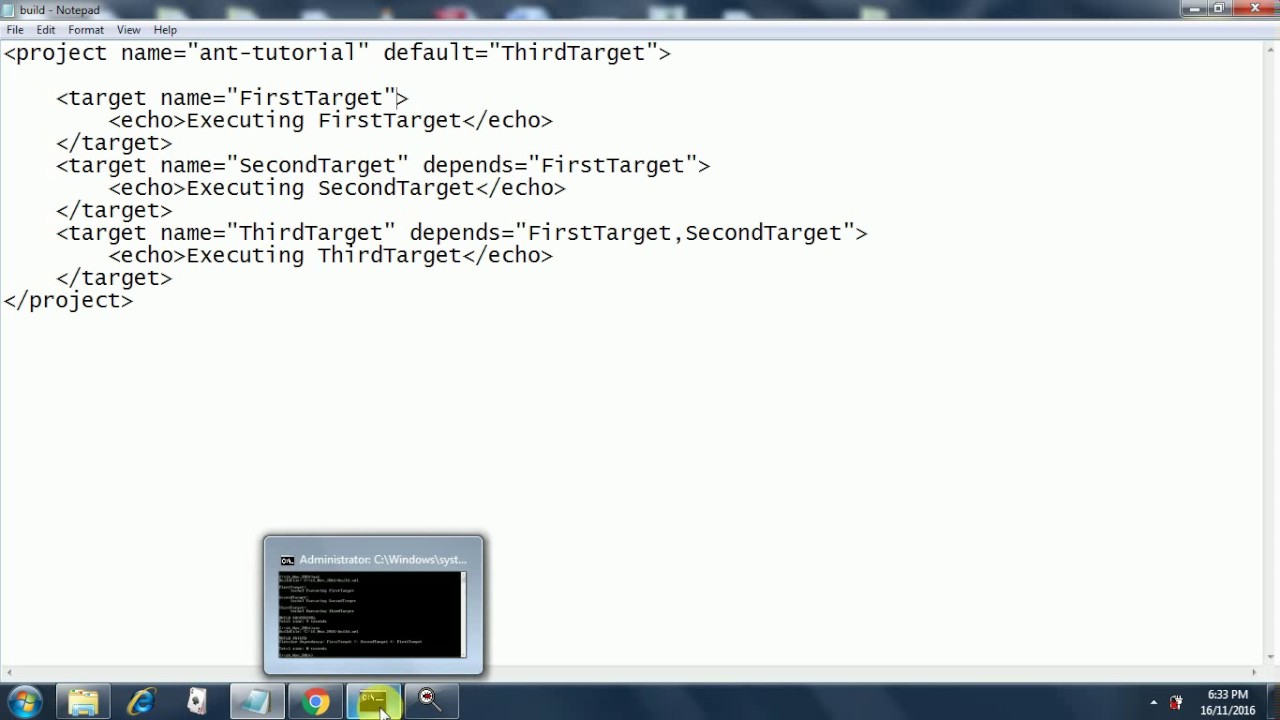
import org.dom4j.io.XMLWriter;
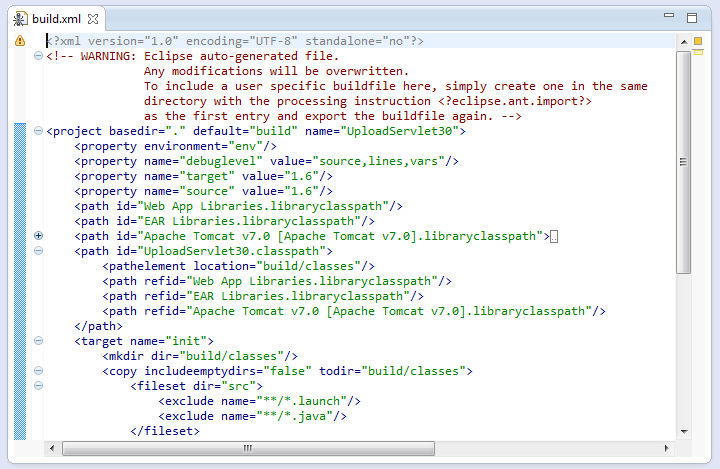
// Create a new document
Document doc = Document.createDocument();
// Create an Element and add it to the document
Element root = doc.addElement("root");
root.addElement("person")
.addAttribute("name", "John")
.addAttribute("age", "30");
// Write the XML file to a file
OutputFormat format = OutputFormat.createPrettyPrint();
XMLWriter writer = new XMLWriter(new FileWriter("example.xml"), format);
writer.write(doc);
This code creates a simple XML document with a root
element, a person
element, and two attributes. The resulting XML file will look like this:
Transform or validate the XML document: Depending on your needs, you might want to transform or validate your XML document using XSLT (XSL Transformations) or an XML schema. Read and process the XML file: Once you have created the XML file, you can read it back into your Java program using a suitable parser and then process its contents.
Some common use cases for building XML files in Java include:
Configuring applications or systems Exchanging data between different systems or languages Storing or retrieving structured dataIn conclusion, building an XML file in Java requires choosing the right XML parser, creating a new document, adding elements and attributes, and writing it to a file. The resulting XML file can be used for various purposes, including configuration, data exchange, or storage.
I hope this explanation has been helpful! Let me know if you have any questions or need further clarification.