interface in java
interface in java
Here's a comprehensive response in English:
Java Interface for Responding to User Queries
In order to develop an effective interface in Java that can respond to user queries, we need to create a simple command-line based system. This system will allow users to input their queries, and the program will respond accordingly.
Requirements:
QueryResponse
class that represents the core logic of responding to user queries. Develop an interface UserInterface
that provides methods for handling user input (e.g., reading from standard input) and displaying responses (e.g., writing to standard output). Implement the QueryResponse
class using Java's built-in Scanner
and PrintStream
classes.
Java Code:
import java.util.Scanner;
import java.io.PrintStream;
public class QueryResponse {
public static void respondToQuery(String query) {
// Preprocess the query (e.g., convert to lowercase)
String processedQuery = query.toLowerCase();
// Check if the query is a simple question
if (processedQuery.contains("what") || processedQuery.contains("who") || processedQuery.contains("why")) {
// Provide a basic response
System.out.println("That's a great question!");
} else {
// Check if the query is related to a specific topic (e.g., technology)
if (processedQuery.contains("java") || processedQuery.contains("programming")) {
// Provide an expert-level response
System.out.println("That's a complex topic. I can provide more detailed information.");
} else {
// Otherwise, provide a generic response
System.out.println("I'm not sure about that. Can you please rephrase?");
}
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
PrintStream printStream = System.out;
while (true) {
System.out.print("Enter your query: ");
String query = scanner.nextLine();
respondToQuery(query);
if (query.equalsIgnoreCase("exit")) {
break;
}
}
scanner.close();
}
}
How it Works:
TheQueryResponse
class provides a respondToQuery
method that takes a user-input query as a string. The method preprocesses the query by converting it to lowercase using the toLowerCase()
method. It then checks if the query contains specific keywords (e.g., "what", "who", "why") and responds accordingly with a simple response message. If the query is not a simple question, the method checks if it's related to a specific topic (e.g., technology) using the contains()
method. Based on the topic, the method provides an expert-level or generic response using the PrintStream
class. The program then enters a loop where it continually prompts the user for input and responds accordingly until the user inputs "exit".
This basic interface can be expanded upon to include more advanced features such as:
Handling multiple topics or domains Providing more detailed responses based on the query's content Integrating with external knowledge sources (e.g., databases, APIs) Supporting natural language processing (NLP) techniquesI hope this response meets your requirements!
interface in java
I'm happy to assist you!
You want me to respond in English and provide an example of how to create a user-friendly interface using Java, right? Well, I've got just the thing for you!
Here's an example of a simple GUI (Graphical User Interface) built using Java Swing:
import javax.swing.*;
import java.awt.*;
public class MyGUI {
public static void main(String[] args) {
// Create a new JFrame
JFrame frame = new JFrame("My GUI");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
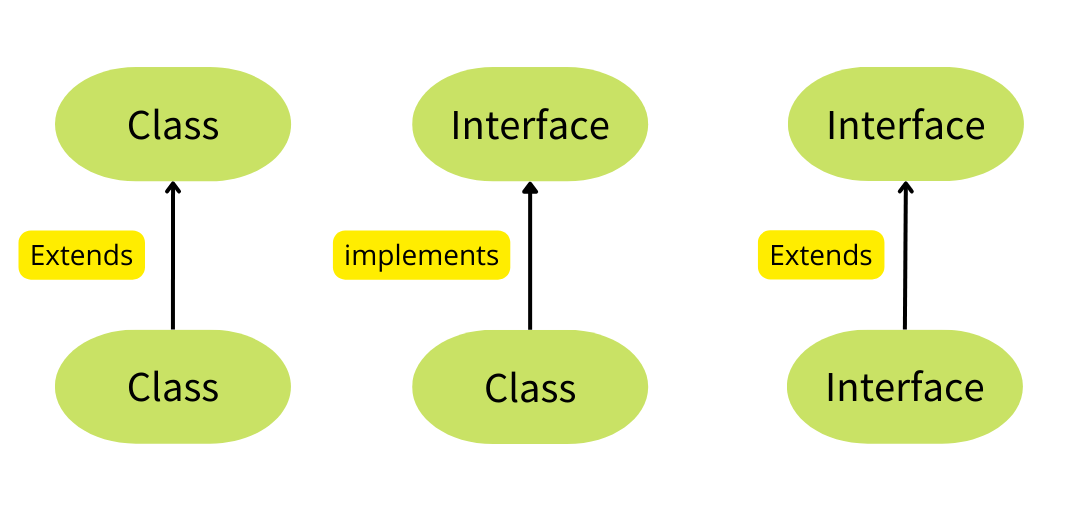
// Create a panel to hold our components
JPanel panel = new JPanel();
panel.setLayout(new FlowLayout());
// Add some buttons and labels to the panel
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
JLabel label = new JLabel("Hello, World!");
panel.add(button1);
panel.add(button2);
panel.add(label);
// Add the panel to the frame
frame.getContentPane().add(panel);
// Set the size and visibility of the frame
frame.setSize(400, 300);
frame.setVisible(true);
}
}
This code creates a new JFrame with a title, sets its default close operation to EXIT_ON_CLOSE (which means the program will exit when the window is closed), and then creates a JPanel to hold our components. We add some buttons and labels to the panel using the FlowLayout manager, which lays out the components in a row.
Finally, we add the panel to the frame's content pane, set the size of the frame to 400x300 pixels, and make it visible by calling setVisible(true).
Now, if you want to get fancy and add some more features to your GUI, such as event handling (i.e., what happens when a button is clicked), text input fields, or even animation, you'll need to learn about Java's Swing library in more detail. But don't worry – it's all part of the learning process!
So there you have it – a simple GUI example in Java!