How do you call the main class in Java?
How do you call the main class in Java?
The main class in Java! That's a crucial concept to grasp when learning the world of programming with Java.
In Java, the main class is the starting point for any program that uses the Java Virtual Machine (JVM) to run. It's the entry point where your program begins execution. This special class contains the main
method, which is the first method called by the JVM when it starts running your program.
The main
method is a static method, meaning it can be called without creating an instance of the class. Its signature looks like this:
public static void main(String[] args) {
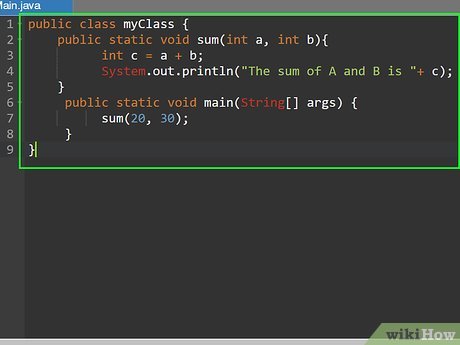
// Your program's entry point!
}
Now, let's talk about what makes a Java class "the main class." To be considered the main class, your Java file (usually with a .java
extension) must meet these conditions:
.java
extension). For example, if your file is named HelloWorld.java
, then your class must be declared as public class HelloWorld
. Public access modifier: Your main class must have a public access modifier to allow the JVM to find it. Main method: Your class must contain a main
method with the exact signature shown above (static, void returning, taking an array of Strings).
Here's an example of a simple Java program that demonstrates these conditions:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
When you run this program, the JVM will execute the main
method in the HelloWorld
class, and print "Hello, World!" to the console.
In summary, the main class in Java is a special class that serves as the starting point for your program. It must have a public access modifier, be named exactly like the filename (excluding the .java
extension), and contain a main
method with a specific signature.
Now, go forth and create some amazing Java programs!
How to call a class in java w3schools
I'm happy to help you with that! According to W3Schools, in Java, you can create a new instance of a class by calling its constructor method. Here's how:
public class MyClass {
private String myVar;
public MyClass(String var) {
myVar = var;
}
public void printMyVar() {
System.out.println(myVar);
}
}
To call the MyClass
class and create a new instance of it, you would write:
public class Main {
public static void main(String[] args) {
MyClass obj = new MyClass("Hello");
obj.printMyVar();
}
}
In this example:
TheMyClass
constructor is called with the argument "Hello"
when creating a new instance of the class using the new
keyword. The printMyVar()
method prints out the value stored in myVar
, which in this case is "Hello"
. When you run this program, it will print out Hello
.
Here are some key points to note:
You can create multiple instances of a class by calling its constructor multiple times. Each instance has its own set of variables and methods that can be accessed independently. You can also create an instance with no arguments if your class's constructor doesn't take any parameters.Here is another example, this time with a simple math calculation:
public class Calculator {
private int num1;
private int num2;
public Calculator(int n1, int n2) {
num1 = n1;
num2 = n2;
}
public int addNumbers() {
return num1 + num2;
}
}
And you would call this class like so:
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator(3, 5);
int sum = calc.addNumbers();
System.out.println("The sum is: " + sum);
}
}
This example shows how you can use a class to perform calculations and return the result.