When to use async Java Spring Boot?
When to use async Java Spring Boot?
When deciding whether to use Async Java with Spring Boot, you should consider the following factors:
I/O-bound vs CPU-bound operations: If your application primarily performs I/O-bound operations (e.g., database queries, file reading/writing), async programming can significantly improve performance and scalability. On the other hand, if your application is CPU-bound (e.g., complex mathematical calculations), you might not see significant benefits from using async. Concurrency requirements: If your application needs to process multiple requests concurrently, async programming can help manage concurrency efficiently. This is particularly important in scenarios where request processing time varies significantly or when handling a high volume of requests. Server resources and memory utilization: Async programming can help optimize server resource utilization by allowing multiple threads to run concurrently, reducing idle times between requests. This is especially beneficial for applications that handle large volumes of concurrent requests.In Spring Boot, you can use async programming to:
Decouple blocking operations: Use @Async annotations on methods that perform I/O-bound operations (e.g., database queries) to decouple them from the main request processing flow. This helps prevent blocking and improves overall system responsiveness. Offload CPU-intensive tasks: When handling CPU-intensive tasks, like image processing or complex calculations, async programming can help offload these tasks onto separate threads, freeing up the main thread for other requests. Implement scalable event-driven architecture: Spring Boot's async capabilities allow you to build scalable, event-driven architectures that can handle high volumes of concurrent requests and events.To take full advantage of async Java with Spring Boot, consider:
Using a Thread Pool Executor: Configure an appropriate thread pool executor (e.g., fixed-thread, cached-thread) to manage the number of threads available for async processing. Setting proper timeout values: Define timeouts for async operations to prevent long-running tasks from blocking other requests or causing performance issues. Monitoring and optimizing: Regularly monitor your application's performance and adjust async configuration settings as needed to ensure optimal resource utilization.Some common use cases for async Java with Spring Boot include:
File uploading and processing: Use async programming to handle file uploads and processing, allowing the main request to continue without blocking. Image processing and resizing: Offload image processing and resizing tasks onto separate threads to improve overall system responsiveness. Notification handling: Implement async programming to handle notifications (e.g., email or SMS) asynchronously, reducing the load on your application's main thread.By understanding when and how to use async Java with Spring Boot, you can build scalable, efficient, and responsive applications that effectively manage concurrency and optimize resource utilization.
Java async annotation example
Here's an example of a Java async annotation using the Spring Framework and its annotation-based programming model:
Let's assume we have a service class MyService
that has a method performAsyncTask()
which takes a long time to execute. We want to annotate this method with @Async so that it runs asynchronously.
@Service
public class MyService {
@Async("taskExecutor")
public void performAsyncTask() {
// Simulate some lengthy operation
for (int i = 0; i < 10000; i++) {
Thread.sleep(10);
System.out.println("Iteration " + i + " of a very long task");
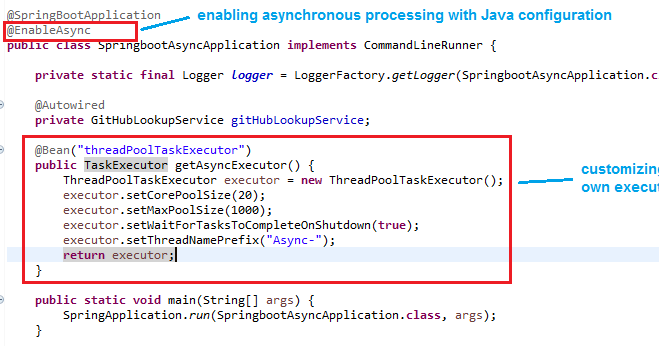
}
}
}
In the above example, @Async
is used to mark the performAsyncTask()
method as an asynchronous task. The argument "taskExecutor"
is a reference to the Spring Task Executor that will be used to execute this task.
Here's how we can configure the task executor in our application context:
@Configuration
public class AppConfig {
@Bean
public AsyncTaskExecutor taskExecutor() {
SimpleAsyncTaskExecutor simpleAsyncTaskExecutor = new SimpleAsyncTaskExecutor();
simpleAsyncTaskExecutor.setConcurrencyLimit(5);
return simpleAsyncTaskExecutor;
}
}
In the above example, we are using a SimpleAsyncTaskExecutor
to execute our tasks. This executor will create a new thread for each task and limit the number of concurrent threads to 5.
Now, let's see how we can use this service class from another part of our application:
@RestController
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/start-task")
public String startAsyncTask() {
myService.performAsyncTask();
return "Started the async task";
}
}
In the above example, we are using the MyService
to start an asynchronous task. The performAsyncTask()
method will be executed asynchronously and the control will immediately return back to our controller.
We can also use the @Async
annotation on a method of a class that implements the InitializingBean
interface to trigger the asynchronous execution of the method after the bean has been initialized:
public class My InitializingBean implements InitializingBean {
@Override
public void afterPropertiesSet() throws Exception {
performAsyncTask();
}
@Async("taskExecutor")
public void performAsyncTask() {
// Simulate some lengthy operation
for (int i = 0; i < 10000; i++) {
Thread.sleep(10);
System.out.println("Iteration " + i + " of a very long task");
}
}
}
In the above example, the performAsyncTask()
method will be executed asynchronously after the bean has been initialized.
That's it! This is a basic example of using Java annotations to create asynchronous tasks in Spring.