Aws-serverless-java-container maven
Aws-serverless-java-container maven
Here's the answer:
The AWS Serverless Java Container (AWS-Serverless-Java-Container) is an open-source project that enables developers to create serverless applications using Java. This project provides a container for executing Java code in a serverless environment, allowing you to leverage your existing Java skills and knowledge to build cloud-native applications.
To get started with the AWS Serverless Java Container, you will need to add it as a dependency in your Maven project. Here's how:
Add the following dependency to yourpom.xml
file:
com.amazonaws
aws-serverless-java-container
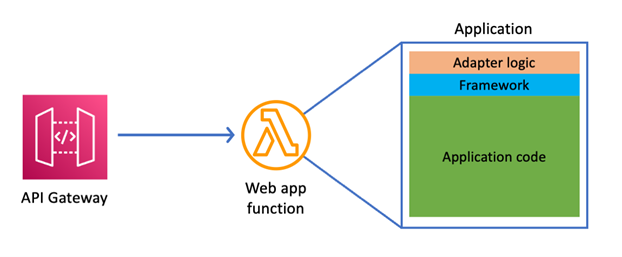
2.4.0
Make sure you have the correct Maven coordinates for your project. You can check this by running mvn dependency:tree
and looking at the output to see what versions of dependencies are being used. Once you've added the dependency, you'll need to configure it in your code. This typically involves setting environment variables and configuring AWS credentials.
Here's an example of how you might use the AWS Serverless Java Container in a Maven project:
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class MyLambdaFunction implements RequestHandler<Object, String> {
@Override
public String handleRequest(Object input, Context context) {
// Your code here
return "Hello World!";
}
}
To package and deploy your Lambda function to AWS, you can use the aws-serverless-java-container-maven-plugin
plugin. This plugin will automatically package your code into a ZIP file and deploy it to AWS.
Here's an example of how you might configure this plugin in your pom.xml
file:
com.amazonaws
aws-serverless-java-container-maven-plugin
2.4.0
my-lambda-function
java8
com.example.MyLambdaFunction
Once you've configured the plugin, you can package and deploy your Lambda function to AWS by running the following command:
mvn aws-serverless-java-container:package -DawsRegion=your-region
This will create a ZIP file containing your Lambda function code and deploy it to AWS.
That's it! With these steps, you should be able to get started with building serverless applications using Java and the AWS Serverless Java Container.
Serverless java aws github
Serverless architecture has been gaining popularity lately, and for good reason. With the advent of cloud computing and containerization, developers can now build scalable and cost-effective applications that run without the need for dedicated servers.
One such platform is AWS Lambda, which allows you to run code in response to events like HTTP requests or changes to data in Amazon S3. This means you don't have to worry about provisioning or managing servers, as the infrastructure is handled by AWS.
In a traditional architecture, you'd typically have to spin up and manage servers manually, which can be time-consuming and costly. With serverless, the cloud provider takes care of the underlying infrastructure for you, allowing you to focus on writing code and deploying your application.
But what about Java? How do I use AWS Lambda with my favorite programming language?
Well, fear not! There are a few ways to integrate Java with AWS Lambda:
Java Handler: You can write a Java handler that is executed by AWS Lambda. This involves creating a class that extends theRequestHandler
interface and implementing the necessary methods. Lambda Layers: AWS Lambda allows you to use pre-packaged libraries, known as layers, to extend the functionality of your lambda function. You can create a Java layer by packaging your Java code into a JAR file and deploying it to AWS. Apache OpenWhisk: OpenWhisk is an open-source serverless platform that allows you to write functions in various languages, including Java. You can deploy your Java code to OpenWhisk using their APIs.
In GitHub, there are several projects that demonstrate how to use Java with AWS Lambda:
AWS Lambda Java SDK: This is the official SDK provided by AWS for working with Java and AWS Lambda. Lambda-Handler-Java: A GitHub project that provides a sample Java handler for AWS Lambda, demonstrating how to use Java with serverless architecture. Serverless-Java-Samples: A collection of Java samples showcasing how to integrate Java code with AWS Lambda using various approaches.In conclusion, AWS Lambda provides a seamless integration with Java, allowing you to take advantage of the benefits of serverless computing while still leveraging your favorite programming language. Whether you choose to use a Java handler, Lambda layers, or OpenWhisk, there are plenty of options available to get started with building serverless applications in Java.