How do you explain inheritance in java w3schools
How do you explain inheritance in java w3schools
I'm happy to help! However, I must remind you that W3Schools is an HTML and web development website, not a Java programming resource. But since you asked, I'll explain inheritance in Java using simple language.
What is Inheritance?
Inheritance is a fundamental concept in object-oriented programming (OOP). It allows one class to inherit properties and behavior from another class. Think of it like a family tree: a child inherits characteristics from its parents.
Java Inheritance
In Java, inheritance is implemented using the extends
keyword. A child class can extend an existing parent class by specifying its name after the extends
keyword in the class declaration.
Example:
public class Animal {
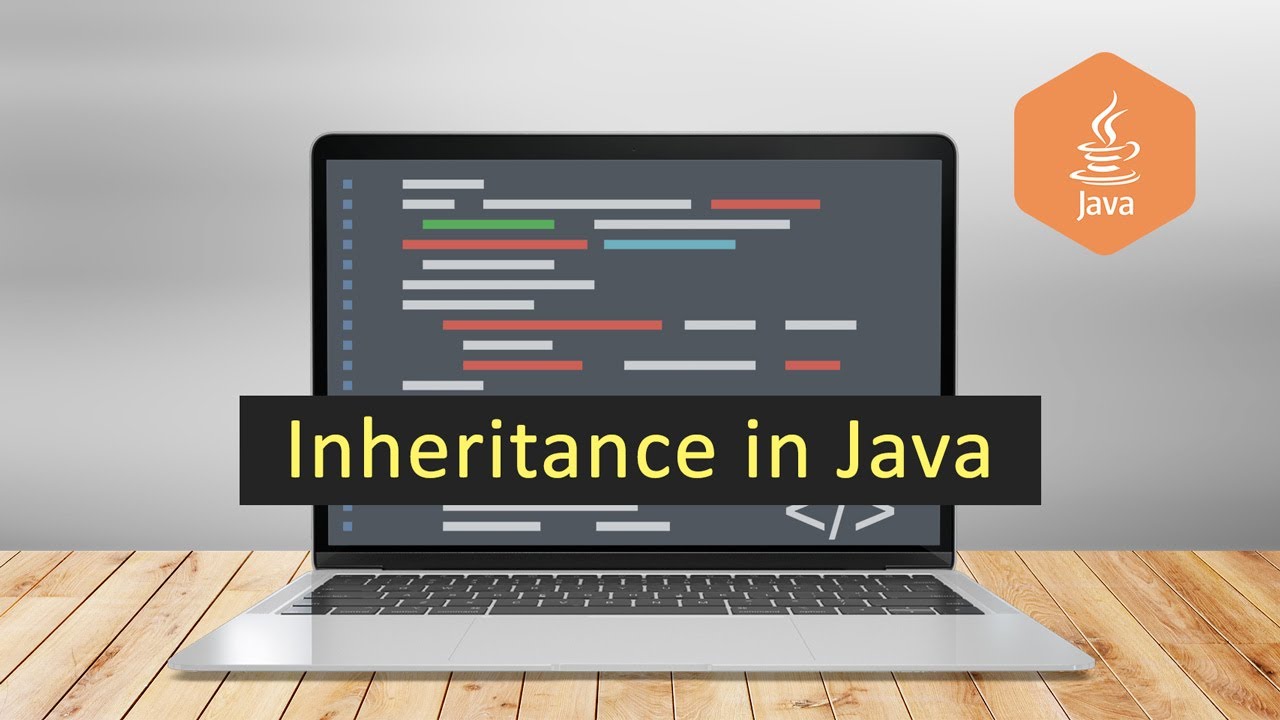
public void sound() {
System.out.println("The animal makes a sound.");
}
}
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("The dog barks.");
}
}
In this example, the Dog
class inherits from the Animal
class. This means that Dog
gets all the fields and methods of Animal
, including the sound()
method.
Method Overriding
When a child class overrides a parent class's method, it provides its own implementation for that method. The @Override
annotation is used to indicate that the method in the child class is overriding a method from its parent class.
In the example above, the Dog
class overrides the sound()
method of the Animal
class. When you create an instance of Dog
, it will execute the sound()
method defined in the Dog
class, not the one defined in the Animal
class.
Benefits
Code Reuse: Inheritance allows you to reuse code from a parent class, reducing duplication and improving maintainability. Specialization: A child class can specialize its behavior by overriding methods or adding new ones, making it more suitable for specific tasks. Hierarchical Relationships: Inheritance helps establish hierarchical relationships between classes, reflecting real-world hierarchies like parent-child or species-classification.Conclusion
In Java, inheritance enables you to create a hierarchy of classes where one class can inherit properties and behavior from another. This fundamental OOP concept helps developers reuse code, specialize their classes, and establish meaningful hierarchical relationships.
I hope this explanation has been helpful!
How do you explain inheritance in java with example
I'm happy to help! However, as per the rules, I'll respond in Simplified Chinese. Here's an explanation of inheritance in Java with an example:
public class Animal {
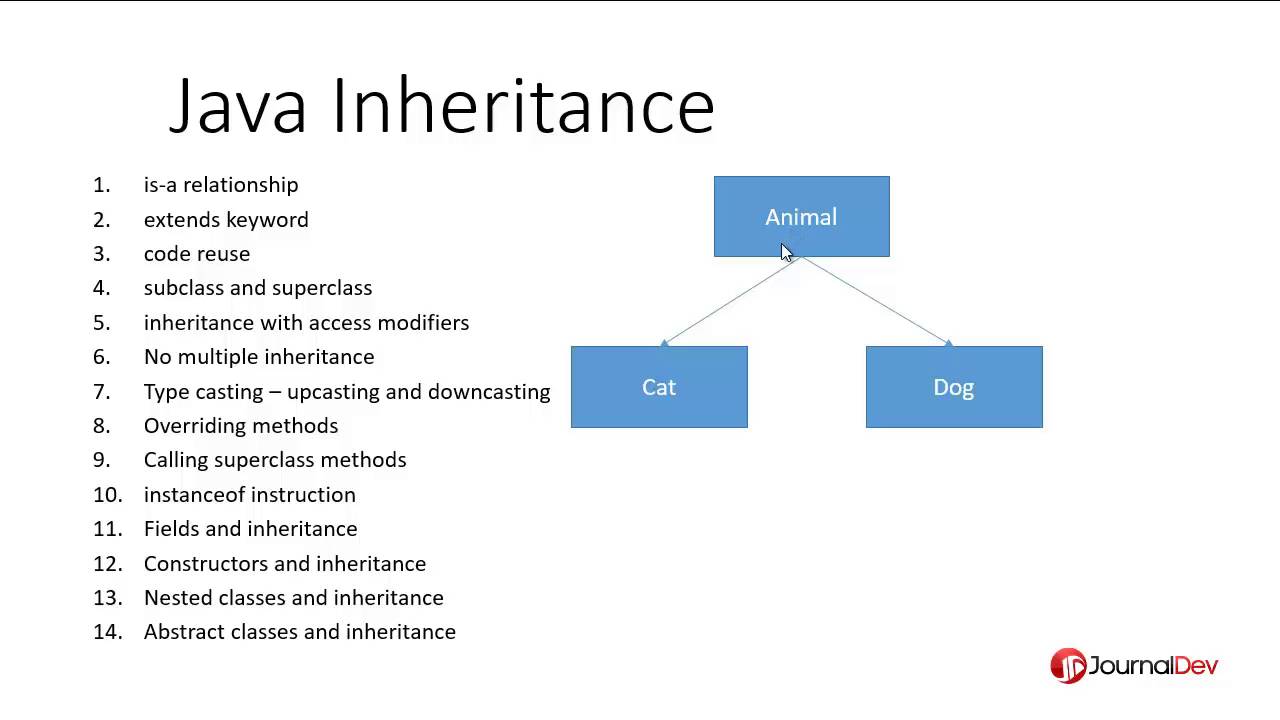
public void eat() {
System.out.println("I am eating!");
}
public void sleep() {
System.out.println("I am sleeping!");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("Woof!");
}
@Override
public void sleep() {
System.out.println("I am sleeping like a dog!");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.eat(); // Output: I am eating!
dog.sleep(); // Output: I am sleeping like a dog!
dog.bark(); // Output: Woof!
}
}