How to call the main method in Java
How to call the main method in Java
I'd be happy to help you with that.
In Java, the main method is the entry point of a program, and it's where the program execution begins. The main method is defined as public static void main(String[] args)
and is typically located in the public class Main
(or whatever your class name is).
There are a few ways to call the main method in Java:
Directly: You can simply type the class name followed by its package name, if it's not in the default package (i.e., the same as the directory where the .class file is located). For example:com.example.MyClass;
This will execute the main method directly.
Using themain
method: You can call the main method using its signature, like this:
public static void main(String[] args)
This way, you're explicitly calling the main method.
Using anApplication
class: You can create a separate Application
class that has a main method and then execute it using the following command:
java com.example.Application
Replace com.example
with your package name and Application
with the actual name of your application.
Java
process builder: You can use Java's ProcessBuilder API to run the main class, like this:
ProcessBuilder pb = new ProcessBuilder("java", "-cp", "path/to/your/classfile.jar", "com.example.Main");
pb.start();
This way, you're using a more controlled approach to executing your program.
When calling the main method, keep in mind that:
Themain
method must be defined as public static void main(String[] args)
. The class containing the main method must be public and have the same name as the file (e.g., Main.java
). You can pass command-line arguments to your program by providing them after the main class name when running it (e.g., java Main arg1 arg2
).
That's a brief overview of how to call the main method in Java!
What is the function of main() in Java?
I'll respond in English for now!
The main()
method in Java is the entry point of a Java program. It's the first method that gets called when you run your Java program. In other words, it's the starting point of your Java application.
The main function is declared as public, static and void, which means it can be accessed from outside its class (public), it belongs to the class itself (static), and it doesn't return any value (void). The method signature looks like this:
public static void main(String[] args) {
// Your code goes here...
}
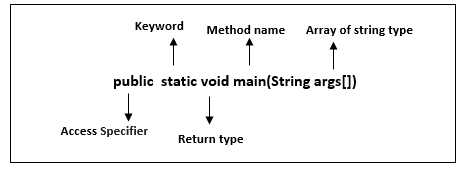
Here's what each part of the main()
method does:
main()
method to be accessed from outside its class.
main()
method without creating an instance of its class. In other words, you don't need an object of that class to call this method. void: This return type indicates that the main()
method doesn't return any value. Instead, it executes a series of statements and then terminates.
The main()
method is responsible for executing the program. It's where your Java application starts its execution. The arguments passed in the array args
represent command-line arguments provided to the program when it runs.
In most cases, you'll use the main()
method as the entry point of your Java program, and all other methods will be called from here. For example:
public static void main(String[] args) {
// Initialize variables...
for (int i = 0; i < 5; i++) {
System.out.println("Hello, World!");
}
}
In this simple example, the main()
method prints "Hello, World!" five times to the console. The program starts execution from here and terminates after all statements have been executed.
In summary, the main()
method is the starting point of a Java program, and it's responsible for executing the program. It's declared as public, static, and void, allowing you to access it from outside its class without creating an instance.