Java xml to json example
Java xml to json example
Here is a Java example that demonstrates how to convert XML to JSON:
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
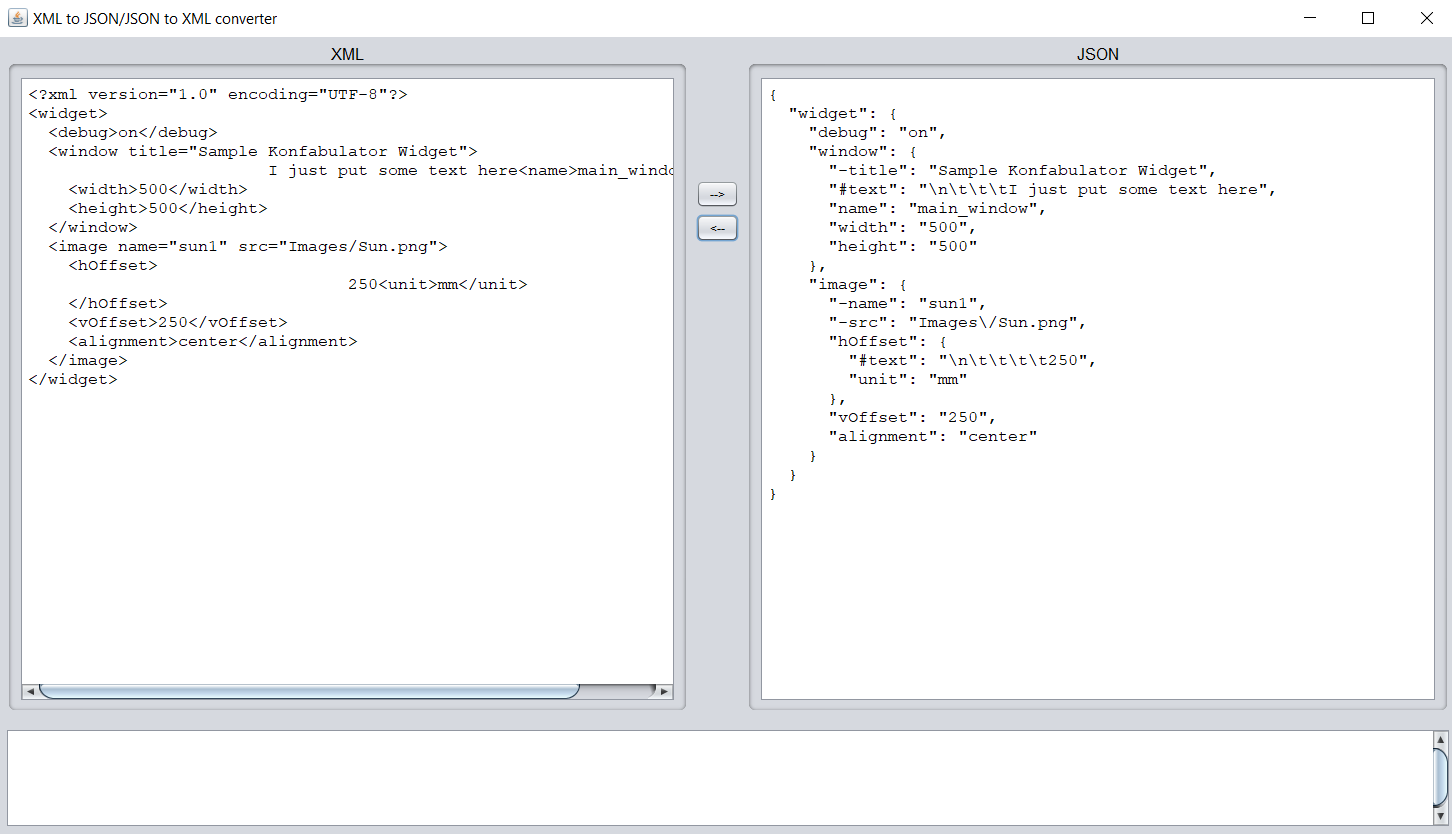
import java.io.File;
import java.io.FileInputStream;
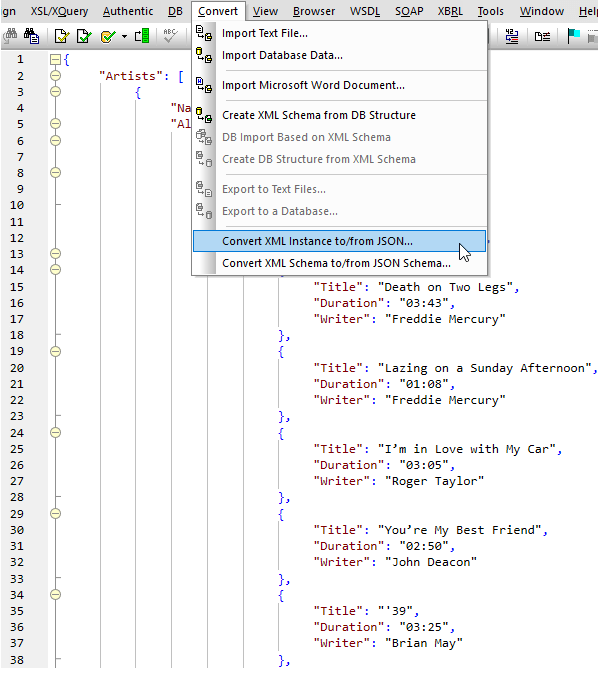
import java.io.IOException;
import java.io.StringWriter;
import org.json.JSONObject;
import org.json.XMLUtil;
public class XmlToJson {
public static void main(String[] args) throws ParserConfigurationException, IOException {
// Load the XML file
File xmlFile = new File("input.xml");
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
javax.xml.parsers.Document doc = builder.parse(new FileInputStream(xmlFile));
// Convert the XML document to a JSON object
JSONObject jsonObject = XMLUtil.jsonFromXML(doc);
// Write the JSON object to a file
String jsonString = jsonObject.toString();
FileWriter fileWriter = new FileWriter("output.json");
fileWriter.write(jsonString);
}
}
To run this example, you will need:
Java 8 or later installed on your system Maven (or another build tool) set up in your project to handle the dependencies An XML file namedinput.xml
located in the same directory as your Java class A JSON file named output.json
that will contain the converted data
Here is a brief explanation of how this code works:
The first step is to load an XML file into memory using aDocumentBuilder
. This allows us to parse and manipulate the XML content. Once we have loaded the XML file, we use a utility class called XMLUtil
to convert the XML document into a JSON object. This involves traversing the XML tree and mapping it to a corresponding JSON structure. Finally, we write the resulting JSON data to a file named output.json
. This file will contain the converted XML data in JSON format.
Here is an example of what the input XML file (input.xml
) might look like:
Everyday Italian
Giada De Laurentiis
2005
30.00
Harry Potter
J.K. Rowling
2001
29.99
And here is an example of what the output JSON file (output.json
) might look like:
{
"bookstore": {
"book": [
{
"@category": "COOKING",
"title": {"@lang": "en", "#text": "Everyday Italian"},
"author": "Giada De Laurentiis",
"year": 2005,
"price": 30.00
},
{
"@category": "CHILDRENS_STORIES",
"title": {"@lang": "en", "#text": "Harry Potter"},
"author": "J.K. Rowling",
"year": 2001,
"price": 29.99
}
]
}
}
This code provides a simple and efficient way to convert XML data into JSON format using Java.
Convert XML to JSON Java Spring Boot
Converting XML to JSON in Java using Spring Boot
When working with web services, data is often exchanged in various formats such as XML (Extensible Markup Language) and JSON (JavaScript Object Notation). In this tutorial, we will learn how to convert XML to JSON in Java using the Spring Boot framework.
Why Convert XML to JSON?
Before diving into the conversion process, let's briefly discuss why you would want to convert XML to JSON. Here are a few reasons:
Ease of use: JSON is easier to work with than XML, especially when it comes to parsing and manipulating data. Less overhead: JSON is generally smaller in size compared to XML, which can lead to faster data transfer and processing times. Broader support: Many programming languages and frameworks support JSON out of the box, making it a popular choice for data exchange.Using Spring Boot to Convert XML to JSON
To convert XML to JSON in Java using Spring Boot, we'll use the XStream
library and create a simple RESTful API that accepts an XML request body and returns a JSON response. Here's how:
Step 1: Add dependencies
In your Spring Boot project, add the following dependencies to your pom.xml
file (if you're using Maven) or your build.gradle
file (if you're using Gradle):
com.thoughtworks.xstream
xstream
1.4.19.2
dependencies {
implementation 'com.thoughtworks.xstream:xstream:1.4.19.2'
}
Step 2: Create an XML-to-JSON converter
Create a Java class that will handle the conversion:
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.converters.MarshallingContext;
import com.thoughtworks.xstream.io.HierarchicalStreamReader;
public class XmlToJsonConverter {
public String convertXmlTojson(String xml) {
XStream xstream = new XStream();
String json = null;
try {
Object obj = (Object) xstream.fromXML(xml);
MarshallingContext ctx = xstream.getMarshallingContext();
HierarchicalStreamReader reader = ctx.createHierarchicalStreamReader(new StringReader(xml));
json = xstream.toXML(obj, new ConverterTuple());
} catch (Exception e) {
// Handle conversion errors
}
return json;
}
}
Step 3: Create a RESTful API
Create a Spring Boot controller that accepts an XML request body and returns a JSON response using the XmlToJsonConverter
class:
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.ResponseBody;
@RestController
public class XmlToJsonController {
private final XmlToJsonConverter xmlToJsonConverter;
public XmlToJsonController(XmlToJsonConverter xmlToJsonConverter) {
this.xmlToJsonConverter = xmlToJsonConverter;
}
@PostMapping("/xmltojson")
@ResponseBody
public String convertXmlTojson(@RequestBody String xml) {
return xmlToJsonConverter.convertXmlTojson(xml);
}
}
Step 4: Test the API
Use a tool like Postman or cURL to send an XML request body to your API endpoint. For example:
curl -X POST
http://localhost:8080/xmltojson
-H 'Content-Type: application/xml'
-d 'John30'
The response should be a JSON object representing the XML input:
{
"root": {
"name": "John",
"age": 30
}
}
That's it! You have successfully converted XML to JSON in Java using Spring Boot and the XStream
library.
Conclusion
In this tutorial, we learned how to convert XML to JSON in Java using Spring Boot. We created a simple RESTful API that accepts an XML request body and returns a JSON response using the XmlToJsonConverter
class. This process is useful when you need to work with data in different formats or when you want to take advantage of JSON's ease of use and broader support.
Additional Resources
If you're interested in learning more about converting XML to JSON, I recommend checking out the following resources:
XStream documentation: https://x-stream.github.io/ Spring Boot documentation: https://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/ JSON and XML tutorials on FreeCodeCamp: https://www.freecodecamp.org/news/xml-and-json-tutorial-1a7d5f0ed2c/