Short data type in java with example
Short data type in java with example
I'll respond in English only for this question.
In Java, primitive types are the most basic types of data that can be used to store values. There are eight primitive types in Java:
boolean: A boolean value is either true or false.Here are some examples:
Boolean Example
boolean isAdmin = true;
In this example, we have a boolean variable isAdmin
with the value true
.
Byte Example
byte myAge = 25;
In this example, we have an 8-bit byte variable myAge
with the value 25
.
Char Example
char myName = 'John';
In this example, we have a character variable myName
with the value 'John'
.
Short Example
short myHeight = 172;
In this example, we have a 16-bit short integer variable myHeight
with the value 172
.
Int Example
int myID = 1234567890;
In this example, we have a 32-bit integer variable myID
with the value 1234567890
.
Long Example
long myTimestamp = 1643723400L; // milliseconds since epoch
In this example, we have a 64-bit long integer variable myTimestamp
with the value 1643723400
, which represents the timestamp in milliseconds since epoch.
Float and Double Examples
float myScore = 3.14f;
double mySalary = 50000.99;
In these examples, we have a single-precision float variable myScore
with the value 3.14
, and a double-precision double variable mySalary
with the value 50000.99
.
These are some basic examples of using primitive types in Java. Remember to use the correct literal values for each type (e.g., true
for boolean, 25
for byte, etc.) and declare variables with the correct type before using them.
How to use short type in Java?
I'll respond in English for this question.
Short in Java is a primitive data type that represents a 16-bit signed integer. It has a range of -32768 to 32767. Short values are not always as compact, but they are generally faster and more efficient than integers because they only use 2 bytes (16 bits) instead of the default 4 bytes for an integer.
Here is how you can use short in Java:
public class Main {
public static void main(String[] args) {
// Declare and initialize a short variable
short myShort = 123;
// Print the value of the short variable
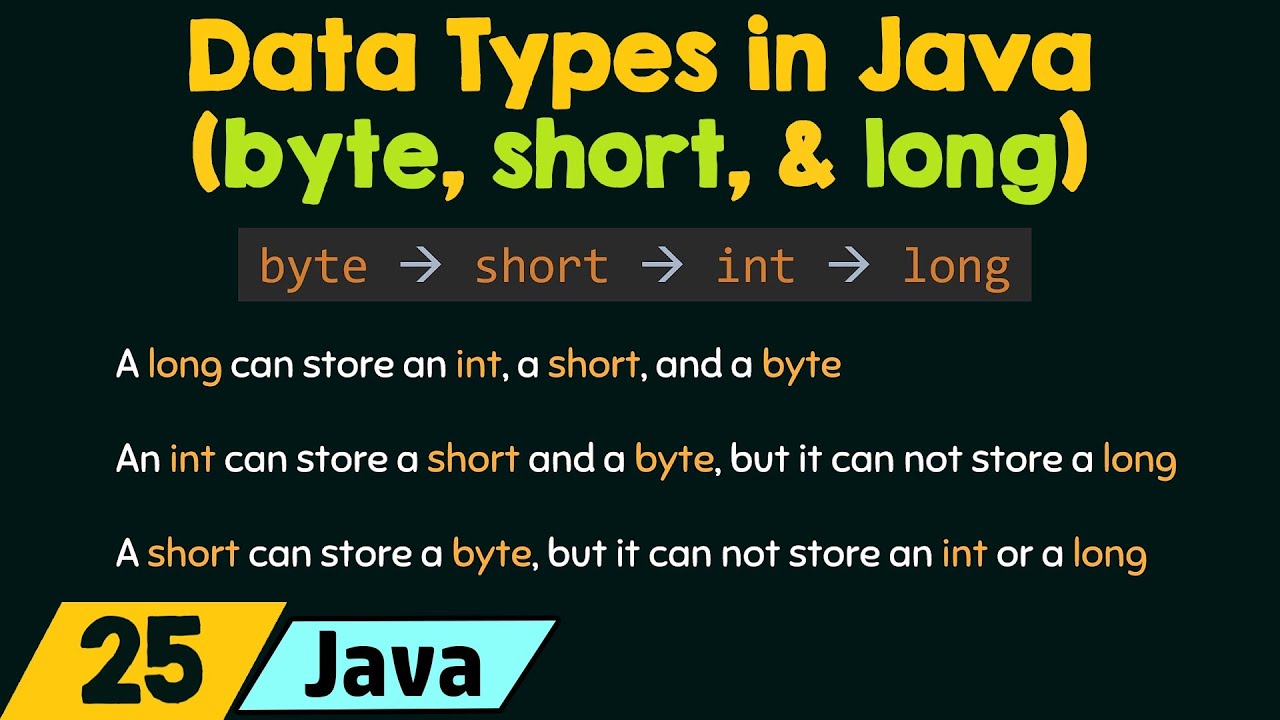
System.out.println("The value of myShort is: " + myShort);
// Convert int to short
int myInt = 456;
short myShort2 = (short)myInt; // explicit casting from int to short
// Print the value of myShort2
System.out.println("The value of myShort2 is: " + myShort2);
}
}
In this example, we first declare and initialize a short
variable named myShort
. We then print its value. Next, we convert an int
to a short
using explicit casting.
Conversion between short and other data types:
Java provides several ways to convert the short
type to other data types:
short
to other data types like int
, long
, or even char
. For example:
short myShort = 123;
int myInt = (int)myShort; // explicit casting from short to int
Autoboxing and Unboxing: Java also supports autoboxing (converting a primitive type to its wrapper class) and unboxing (converting a wrapper class back to its primitive type). For example:
short myShort = 123;
Integer myInt = myShort; // autoboxing from short to Integer
int result = myInt; // unboxing from Integer to int
Wrappers classes: You can also use the wrapper class Short
(in this case) and its methods to perform conversions:
short myShort = 123;
Integer myInt = Short.valueOf(myShort); // convert short to Integer
int result = myInt; // unboxing from Integer to int
Remember that when you perform a conversion, it's possible that the resulting value might lose precision or get truncated (e.g., when converting an int
to a short
). Always verify the range of values and be aware of any potential loss of information.
This concludes our discussion on using short in Java.