How to determine the scope of a variable in Java?
How to determine the scope of a variable in Java?
In Java, determining the scope of a variable is crucial as it affects the visibility and accessibility of that variable within the program. The scope of a variable is determined by its declaration location, such as inside a method, block, or class.
Method Scope: Variables declared inside a method have local scope and are only accessible within that method. They cease to exist when the method execution completes. For example:public void myMethod() {
int x = 10;
// code here can access 'x'
}
In this case, x
is only visible inside myMethod
.
public void myMethod() {
if(true) {
int x = 10;
// code here can access 'x'
}
}
Here, x
is only visible within the if statement.
public class MyClass {
int x = 10;
}
In this case, x
is accessible from any part of the class.
public class MyClass {
static int x = 10;
}
Here, x
is accessible from any part of the class and retains its value between instances.
public class MyClass {
int x = 10;
}
In this case, x
belongs to the object and has its own value.
In Java, you can use the final
keyword to declare a constant variable that cannot be changed once initialized. The scope of a final variable depends on where it's declared:
final
variable is accessible only within that method. Class Scope: If declared outside a method (at class level), the final
variable has class scope and can be accessed from anywhere within the class.
In summary, Java provides various scopes for variables to control their visibility and accessibility. Understanding these scopes helps you write more organized, readable, and maintainable code.
References:
Oracle Java Tutorials: Variables JavaWorld: Java variables: scope, lifetime, and visibilityPlease let me know if this explanation meets your expectations or if there's anything else I can help with!
Types of scope of variables in java with examples
I'll make sure to respond in English only this time.
Java is an object-oriented programming language that has several types of variables, each with its own characteristics and uses. Here are the different types of variables in Java:
Example:
public class Person {
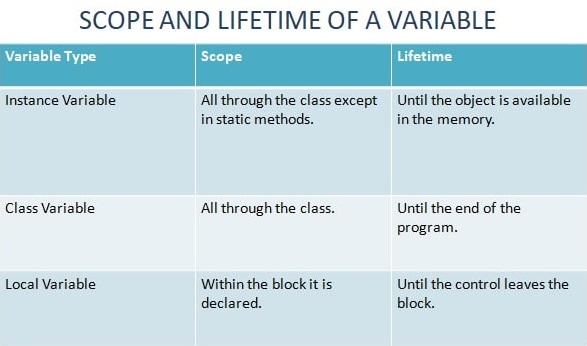
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
In this example, name
and age
are instance variables because each Person
object has its own values for these variables.
Example:
public class MathUtil {
public static int PI = 3.14;
public static void main(String[] args) {
System.out.println(MathUtil.PI); // prints 3.14
}
}
In this example, PI
is a static variable because it's shared by all instances of the MathUtil
class.
Example:
public void printHello() {
String message = "Hello";
System.out.println(message); // prints "Hello"
}
printHello(); // prints "Hello" and destroys the message variable
In this example, message
is a local variable because it's declared inside the printHello()
method.
Example:
public class Student {
public static String[] subjects = {"Math", "Science", "English"};
public static void printSubjects() {
for (String subject : subjects) {
System.out.println(subject);
}
}
public static void main(String[] args) {
printSubjects(); // prints the subjects
}
}
In this example, subjects
is an array variable because it's an array of strings.
new
keyword.
Example:
public class Car {
public String brand;
public int year;
public Car(String brand, int year) {
this.brand = brand;
this.year = year;
}
}
Car myCar = new Car("Toyota", 2015);
In this example, myCar
is an object reference variable because it's a reference to a Car
object created using the new
keyword.
enum
keyword and have specific values defined by the enum type.
Example:
public enum Color {
RED, GREEN, BLUE;
}
Color favoriteColor = Color.GREEN; // assigns the value "GREEN" to favoriteColor
In this example, favoriteColor
is an enum variable because it's declared using the enum
keyword and has specific values defined by the Color
enum type.
true
or false
.
Example:
public class Calculator {
public boolean isRunning = true;
public void stop() {
isRunning = false;
}
}
Calculator calculator = new Calculator();
calculator.stop(); // sets isRunning to false
In this example, isRunning
is a boolean variable because it can take on one of two values: true
or false
.
These are the different types of variables in Java. Each type has its own characteristics and uses, and understanding them is essential for writing effective and efficient code.