Java Metaspace
Java Metaspace
I'll respond in English this time.
What is Java Metaspace?
Java Metaspace is a crucial component of the Oracle Java Virtual Machine (JVM). Introduced in Java SE 8, it replaces the traditional PermGen space and provides significant improvements in garbage collection, heap size management, and overall JVM performance. In essence, Metaspace is an area within the heap where metadata about classes, interfaces, and methods are stored.
Key Features of Java Metaspace:
Metadata Storage: Metaspace stores information about classes, interfaces, methods, and other program elements, such as method signatures, field types, and constructor details. Garbage Collection: Unlike PermGen, which had a fixed size and was prone to memory leaks, Metaspace uses generational garbage collection, allowing it to dynamically adjust its size based on the needs of your application. Heap Size Management: The JVM automatically manages Metaspace's heap size to ensure that metadata is stored efficiently without consuming excessive memory. Memory-Efficient: Metaspace compresses and caches metadata to minimize memory usage, reducing the risk of OutOfMemoryErrors (OOMs). Improved Performance: By optimizing memory allocation and garbage collection, Java Metaspace enhances overall JVM performance, making it more responsive and efficient.How Does Java Metaspace Work?
When your application loads a class or interface, the JVM stores its metadata in the Metaspace area of the heap. This process involves the following steps:
Class Loading: The JVM loads the requested class or interface into memory. Metadata Generation: The JVM generates and caches metadata about the loaded classes and interfaces. Metaspace Allocation: The JVM allocates space within Metaspace for storing the generated metadata. Garbage Collection: The JVM performs garbage collection on Metaspace to remove any unused or redundant metadata.Benefits of Using Java Metaspace:
Improved Performance: By optimizing memory allocation and garbage collection, Java Metaspace enhances overall JVM performance. Reduced Memory Usage: Metaspace's compressed and cached metadata minimize memory consumption, reducing the risk of OOMs. Enhanced Heap Management: The JVM dynamically adjusts Metaspace's heap size based on application needs, ensuring efficient storage of metadata.In conclusion, Java Metaspace is a significant enhancement to the JVM, providing improved garbage collection, heap size management, and overall performance. By understanding how Metaspace works and its benefits, developers can better optimize their applications for robustness and efficiency.
Static and non static memory allocation in Java
I'd be happy to help you with that!
In Java, memory allocation is divided into two categories: static and non-static.
Static Memory Allocation
When an object of a class is created using the new
keyword, it is allocated on the heap, which is managed by the garbage collector. This type of allocation is called dynamic or runtime memory allocation. The lifetime of such objects depends on when they are no longer needed and can be garbage collected.
For example:
public class MyClass {
public int x;
}
If you create an instance of MyClass
using new
, it will be allocated on the heap:
MyClass obj = new MyClass();
obj.x = 10; // assign value to x
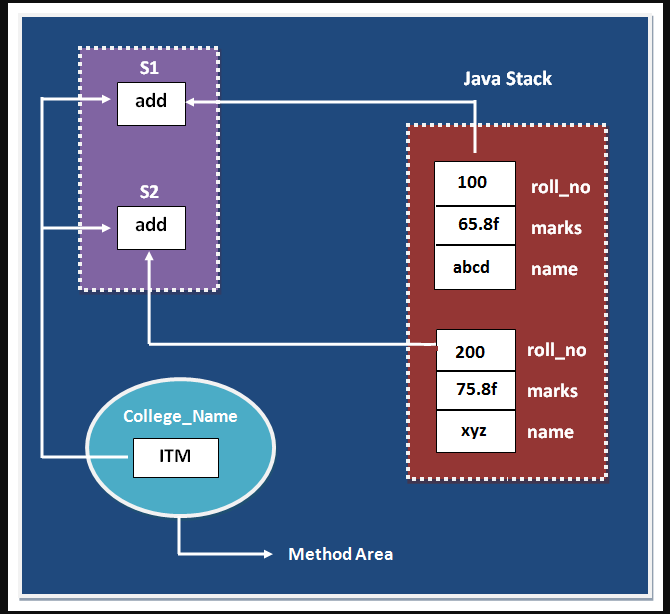
In this case, memory is allocated at runtime when the object is created. The garbage collector will eventually free up the memory once the object is no longer needed.
Non-Static (Instance) Memory Allocation
When you create an instance of a class using the new
keyword, each instance has its own copy of the non-static variables and methods. This type of allocation is also known as dynamic or runtime memory allocation. The lifetime of such objects depends on when they are no longer needed and can be garbage collected.
For example:
public class MyClass {
public int x;
public void foo() {
System.out.println("Hello!");
}
}
If you create multiple instances of MyClass
, each instance will have its own copy of the x
variable and the foo()
method. The memory required to store these variables and methods is allocated at runtime when the objects are created.
Static Memory Allocation
When a variable or method is declared as static, it is shared by all instances of the class. Static members are stored in a separate area of memory called the static area or method area, which is managed by the JVM. This type of allocation occurs once at compile-time, and the same memory location is used for all objects.
For example:
public class MyClass {
public static int y;
public void bar() {
System.out.println(y);
}
}
If you create multiple instances of MyClass
, they will share the same y
variable. The memory required to store the y
variable is allocated at compile-time and remains in memory throughout the lifetime of the program.
Comparison
Here's a summary of the differences between static and non-static (instance) memory allocation:
| | Static Memory Allocation | Non-Static (Instance) Memory Allocation |
| --- | --- | --- |
| Lifetime | Compile-time, shared by all instances | Runtime, depends on garbage collection |
| Memory Location | Shared method area | Heap, managed by garbage collector |
| Variables and Methods | Shared by all instances | Instance-specific |
In conclusion, static memory allocation is used for variables and methods that are shared across all instances of a class, while non-static (instance) memory allocation is used to create separate copies of variables and methods for each instance. Understanding the differences between these two types of memory allocation is crucial when designing and implementing Java programs.