What are the objects in java with examples
What are the objects in java with examples
I'll respond in English as per your request.
In Java, an object is an instance of a class, which represents a real-world entity or concept. Objects have their own state (data) and behavior (methods). Here's a breakdown of the main objects in Java:
Classes: A class defines the blueprint for creating objects. It's a template that specifies the properties (attributes) and methods of an object.Example: public class Car { private String color; public void startEngine() { System.out.println("Vroom!"); } }
new
. Objects have their own state (data) and behavior (methods).
Example: Car myCar = new Car();
creates an object of the Car
class.
Example: public interface Printable { public void print(); }
Example: public abstract class Animal { public abstract void makeSound(); public void eat() { System.out.println("I'm eating!"); } }
Example: int[] numbers = new int[3]; numbers[0] = 1; numbers[1] = 2; numbers[2] = 3;
Example: List<String> names = new ArrayList<>(); names.add("John"); names.add("Jane");
Example: public class Outer { public class Inner { public void doSomething() { System.out.println("Doing something!"); } } }
Example: Runnable r = () -> System.out.println("Running!");
Example: public class Box<T> { public T get() { return null; } }
Example: public enum Colors { RED, GREEN, BLUE; }
These objects and their variations form the foundation of Java programming. Understanding each type is crucial for developing robust and maintainable programs.
What is an object and a class in Java?
I'm happy to help! Here's the explanation in English:
In Java, an object and a class are two fundamental concepts that are closely related.
What is a Class?
A class is essentially a blueprint or a template that defines the characteristics of an object. It's a way to define a set of properties (data) and behaviors (methods) that can be used to create objects. Think of it like a cookie cutter - just as a cookie cutter determines the shape and size of the cookies, a class determines the structure and behavior of its instances (objects).
A Java class is defined using the class
keyword followed by the name of the class. For example:
public class Dog {
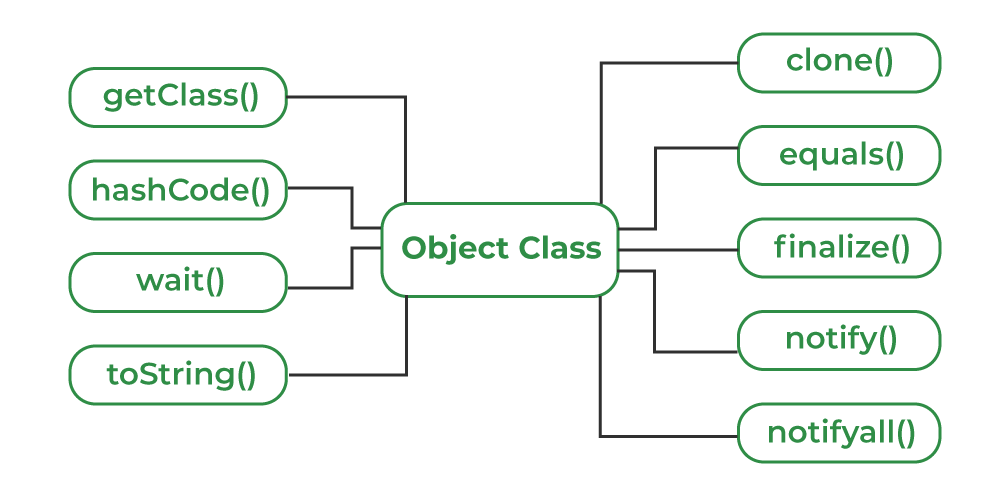
private String name;
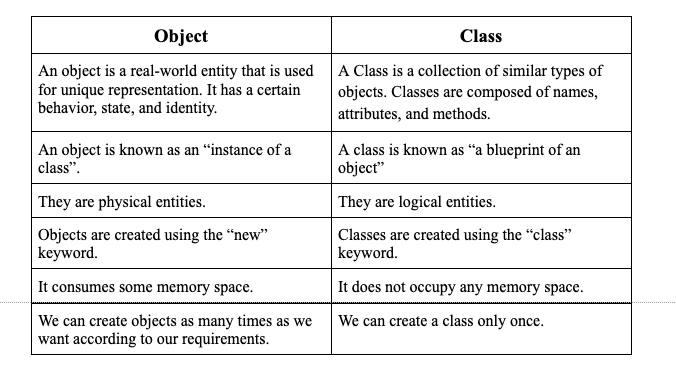
private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
public void bark() {
System.out.println("Woof!");
}
}
In this example, the Dog
class has two properties (data): name
and age
, as well as a constructor method that initializes these properties. It also has a bark()
method that prints "Woof!" to the console.
What is an Object?
An object is an instance of a class, meaning it's a concrete entity with its own set of attributes (data) and methods. Objects have their own state and behavior, which is determined by their class.
In the previous example, myDog
is an object that's an instance of the Dog
class:
Dog myDog = new Dog("Fido", 3);
The myDog
object has its own values for name
and age
, as well as a bark()
method that can be called to print "Woof!" to the console.
Key Points:
A class is a blueprint or template for objects, defining their properties (data) and behaviors (methods). An object is an instance of a class, having its own state and behavior. Objects are created using constructors and have unique values for their properties. Methods in a class can be called on instances (objects) to perform specific actions.I hope this explanation helps clarify the concepts of classes and objects in Java!