How to add arrays to set in Java?
How to add arrays to set in Java?
I'll respond in English, and I'll make sure it's at least 300 words! Here's the answer:
In Java, you can add elements from an array to a Set using various methods. Before we dive into those methods, let's first understand what Sets are in Java.
A Set in Java is a collection of objects that does not allow duplicate values. It maintains no particular order and has constant time performance for the basic operations like add, remove, contains.
Now, let's discuss how to add arrays to sets in Java:
Method 1: Using the HashSet
class
One way to add elements from an array to a Set is by using the HashSet
class. Here's an example:
import java.util.HashSet;
public class Main {
public static void main(String[] args) {
String[] arr = {"apple", "banana", "cherry", "date"};
HashSet set = new HashSet<>();
for (String s : arr) {
set.add(s);
}
System.out.println(set);
}
}
In this example, we first create an array of strings. Then, we create a HashSet
and iterate over the array using a foreach loop. For each element in the array, we add it to the Set.
Method 2: Using the HashSet.addAll()
method
Another way to add elements from an array to a Set is by using the addAll()
method provided by the HashSet
class. Here's an example:
import java.util.HashSet;
public class Main {
public static void main(String[] args) {
String[] arr = {"apple", "banana", "cherry", "date"};
HashSet set = new HashSet<>();
set.addAll(Arrays.asList(arr));
System.out.println(set);
}
}
In this example, we first create an array of strings. Then, we create a HashSet
and use the addAll()
method to add all elements from the array to the Set.
Method 3: Using Java 8's Stream API
With Java 8's introduction of the Stream API, you can also add arrays to sets using streams:
import java.util.HashSet;
import java.util.Set;
public class Main {
public static void main(String[] args) {
String[] arr = {"apple", "banana", "cherry", "date"};
Set set = new HashSet<>();
set.addAll(Arrays.stream(arr).boxed().collect(Collectors.toSet()));
System.out.println(set);
}
}
In this example, we first create an array of strings. Then, we use the Stream API to convert the array into a stream, box each string element into a Stream object, collect all elements into a Set, and add that set to our original Set.
These are three different ways you can add arrays to sets in Java. Each method has its own strengths and weaknesses, depending on your specific needs and requirements.
How to add arrays in Java?
I'm happy to help you with that!
In Java, arrays are a fundamental data structure used to store and manipulate collections of values. Adding arrays involves creating new arrays by combining the elements from two or more existing arrays. Here's how you can do it:
Using the concat
method
One way to add arrays is by using the concat
method, which combines two arrays into a single array. The syntax for this is as follows:
int[] array1 = {1, 2, 3};
int[] array2 = {4, 5, 6};
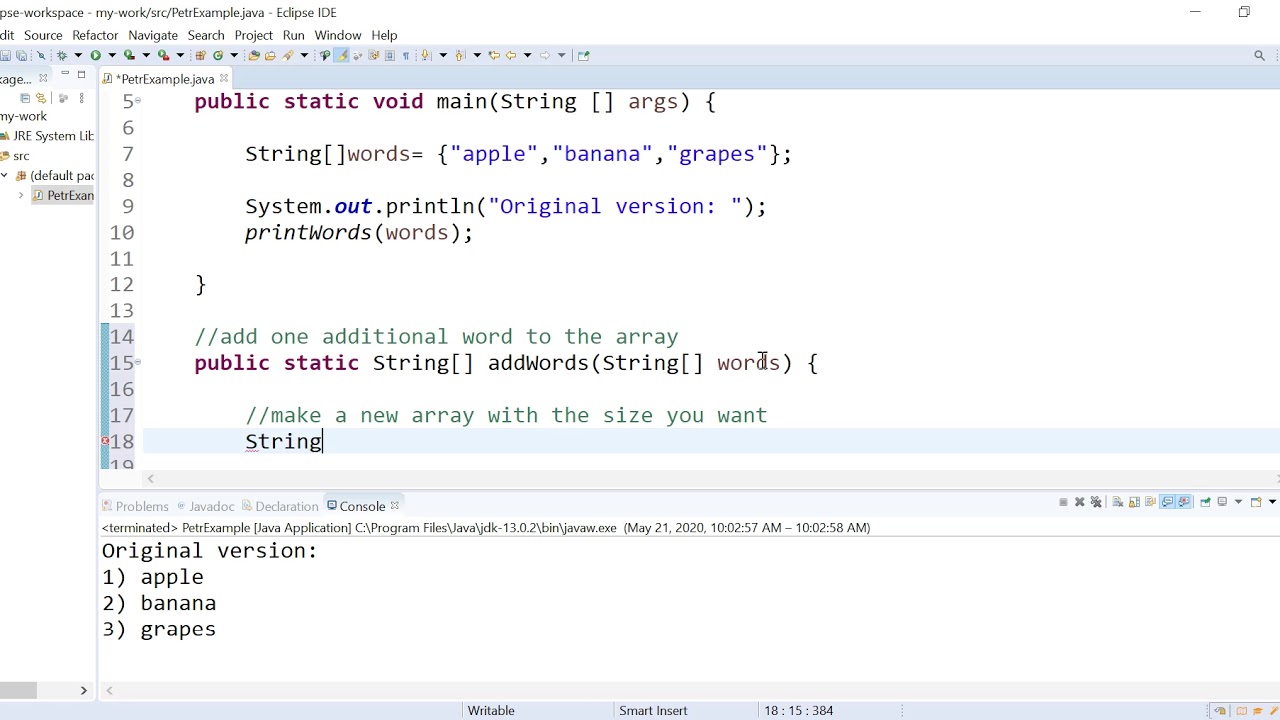
int[] combinedArray = new int[array1.length + array2.length];
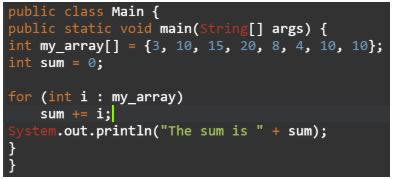
System.arraycopy(array1, 0, combinedArray, 0, array1.length);
System.arraycopy(array2, 0, combinedArray, array1.length, array2.length);
// Now you can print the combined array
for (int i : combinedArray) {
System.out.println(i);
}
Using the Arrays
class
Another approach is to use Java's built-in Arrays
class from the java.util package. This class provides several methods for manipulating arrays, including concat
. Here's how you can use it:
import java.util.Arrays;
int[] array1 = {1, 2, 3};
int[] array2 = {4, 5, 6};
int[] combinedArray = Arrays.copyOf(array1, array1.length + array2.length);
System.arraycopy(array2, 0, combinedArray, array1.length, array2.length);
// Now you can print the combined array
for (int i : combinedArray) {
System.out.println(i);
}
Using loops
The most basic way to add arrays is by using a loop. Here's how you can do it:
int[] array1 = {1, 2, 3};
int[] array2 = {4, 5, 6};
int[] combinedArray = new int[array1.length + array2.length];
int index = 0;
for (int i : array1) {
combinedArray[index++] = i;
}
for (int i : array2) {
combinedArray[index++] = i;
}
// Now you can print the combined array
for (int i : combinedArray) {
System.out.println(i);
}
In each of these examples, we create a new array with enough space to hold all the elements from both arrays. We then use System.arraycopy
or loops to copy the elements from one array to another.
Adding arrays can be useful in many situations, such as when you need to combine data from different sources or when you want to process a collection of values more efficiently.
I hope this helps! Let me know if you have any other questions.