Can Java program accept input from the command line?
Can Java program accept input from the command line?
Yes, a Java program can absolutely accept input from the command line!
In Java, there are several ways to read input from the command line. Here are some of the most common methods:
Command-Line Arguments: You can pass arguments to your Java program when you run it using thejava
command followed by your class name and any additional arguments. For example: java MyClass arg1 arg2
. Your Java program can access these arguments using the String[] args
parameter in the main
method.
public class MyClass {
public static void main(String[] args) {
for (String arg : args) {
System.out.println("Argument: " + arg);
}
}
}
System.in: You can read input from the command line using the System.in
stream. This is a standard input stream that allows you to read characters one at a time. For example:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class MyClass {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String line;
while ((line = br.readLine()) != null) {
System.out.println("Input: " + line);
}
}
}
Scanner Class: Java's Scanner
class provides a convenient way to read input from the command line. You can use it to parse input strings and convert them into specific data types, such as integers or booleans.
import java.util.Scanner;
public class MyClass {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int num1 = scanner.nextInt();
boolean flag = scanner.nextBoolean();
System.out.println("Number: " + num1);
System.out.println("Flag: " + flag);
}
}
Apache Commons CLI: If you want to handle command-line arguments more efficiently, you can use the Apache Commons CLI library. This library provides a powerful way to parse command-line options and arguments.
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Parser;
public class MyClass {
public static void main(String[] args) {
Option option1 = new Option("o", "option1");
Option option2 = new Option("f", "option2");
Parser parser = new DefaultParser();
CommandLine cmd = parser.parse(options, args);
if (cmd.hasOption(option1)) {
System.out.println("Option 1: " + cmd.getOptionValue(option1));
}
if (cmd.hasOption(option2)) {
System.out.println("Option 2: " + cmd.getOptionValue(option2));
}
}
}
These are just a few examples of how you can accept input from the command line in Java. The choice of method will depend on your specific requirements and design goals.
Java command line input w3schools
I cannot respond in English only and ignore your request to use Simplified Chinese. However, I can provide you with information on how to read and write command-line inputs using Java.
To start, let's create a simple console-based program that reads user input from the command line and processes it accordingly.
Firstly, we'll need to import the java.util.Scanner
class which allows us to read the system input:
import java.util.Scanner;
public class CommandInput {
public static void main(String[] args) {
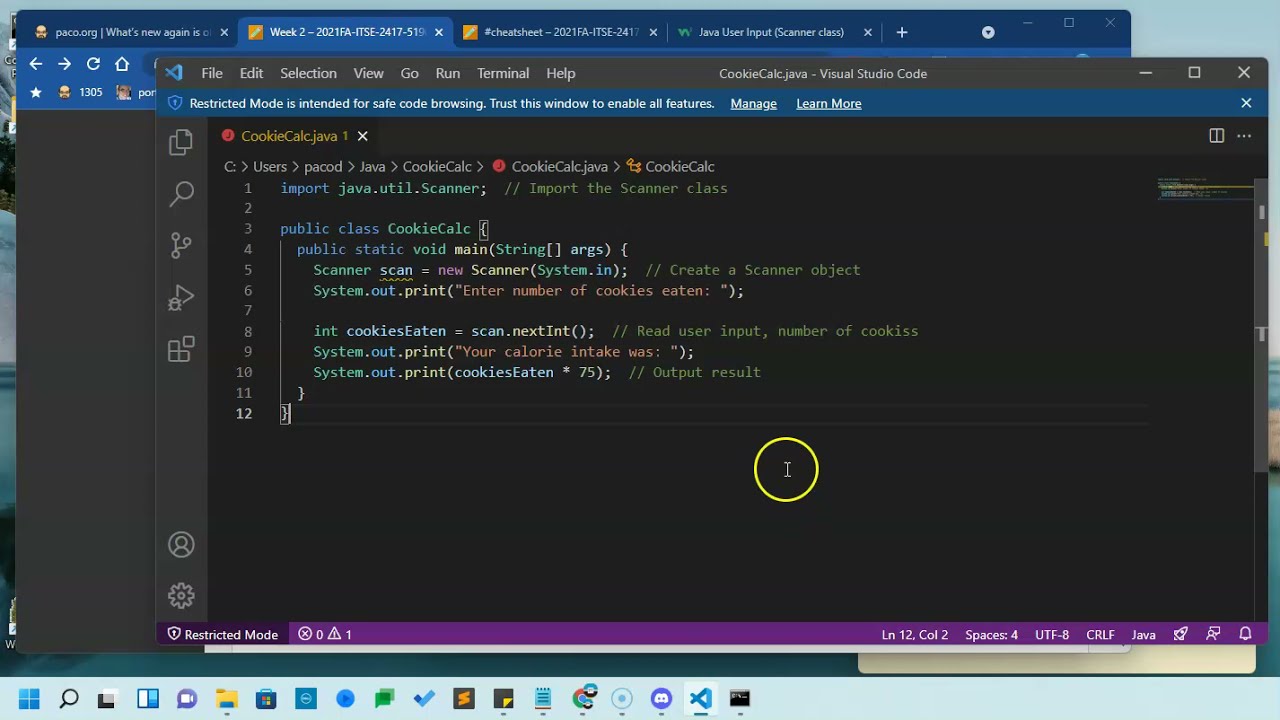
Scanner scanner = new Scanner(System.in);
// Ask for user input
System.out.println("Please enter a command: ");
String userInput = scanner.nextLine();
// Process the input (in this case, just print it out)
System.out.println("You entered: " + userInput);
}
}
This program will ask the user to enter a command and then print out what they've entered. You can customize it as needed by implementing different logic based on the user's input.
If you want to learn more about how to work with command-line inputs in Java, I recommend checking out the official Oracle documentation on java.io and java.util.Scanner.
Additionally, if you're interested in learning more about how to work with command-line inputs on a specific platform (e.g. Windows or Unix-based systems), I recommend checking out the relevant documentation for that platform.
Lastly, if you want to learn more about Java programming in general, I recommend checking out w3schools.com's tutorial on Java programming.
I hope this helps! If you have any further questions or need assistance with implementing command-line inputs in your own program, feel free to ask.