Can we overload non static method in Java
Can we overload non static method in Java
I'm happy to help you with that!
In Java, the concept of overloading is possible for both static and instance methods. However, the rules and constraints governing this process differ slightly between these two categories.
Static Methods:
When it comes to static methods, method overloading works similarly to how it does in C++. You can create multiple methods with the same name but different parameter lists. This means you can have more than one myMethod()
method as long as their parameter types and counts are distinct.
For example:
public class MyStaticClass {
public static void myMethod(int x) {
System.out.println("Int value: " + x);
}
public static void myMethod(double d) {
System.out.println("Double value: " + d);
}
}
In this case, you can call MyStaticClass.myMethod(5)
without any issues, but trying to call MyStaticClass.myMethod(5.0)
would actually invoke the myMethod(double)
method.
Instance Methods (Non-Static):
When it comes to instance methods (non-static), overloading also works similarly, with some subtle differences. You can create multiple methods with the same name but different parameter lists, as long as their return types are not the same or covariant (more on this later).
Here's an example:
public class MyInstanceClass {
public void myMethod(int x) {
System.out.println("Int value: " + x);
}
public void myMethod(double d) {
System.out.println("Double value: " + d);
}
}
However, there's a crucial difference compared to static methods. When calling an instance method, Java uses the method's parameter types and counts to determine which implementation to invoke. This means that if you have multiple myMethod()
methods with different parameter lists, the correct one will be chosen based on the number of arguments you pass when calling the method.
For example:
MyInstanceClass myObject = new MyInstanceClass();
myObject.myMethod(5); // Invokes the int-based implementation
myObject.myMethod(5.0); // Invokes the double-based implementation
In this scenario, Java will automatically select the correct myMethod()
method based on the number and types of arguments you provide.
Covariance:
When overloading instance methods, it's essential to remember that Java does not support covariant return types. This means that if you have multiple myMethod()
methods with different return types (or their subclasses), Java will throw a compile-time error.
For example:
public class MyInstanceClass {
public void myMethod(int x) {
System.out.println("Int value: " + x);
}
public Object myMethod(double d) { // Error! Covariant return type
System.out.println("Double value: " + d);
}
}
In this case, you'll receive a compile-time error because the return types are not identical or covariant.
Conclusion:
To summarize, Java supports method overloading for both static and instance methods. When it comes to static methods, overloading works similarly to C++. For instance methods (non-static), overloading is also possible, but with some crucial differences. Make sure to keep in mind the rules regarding return types and covariant returns to avoid compile-time errors.
Hope that helps!
Why static members cannot be overridden in java example
I apologize for the previous misunderstanding. Here's a response in English:
In Java, static methods (and variables) cannot be overridden because they are bound to a specific class, rather than an instance of that class. When you define a static method or variable, it belongs to the class itself, not any particular object created from that class.
Let's take an example to illustrate this:
Suppose we have a class called "Calculator" with a static method "calculateArea". This method calculates the area of a rectangle based on its length and width.
public class Calculator {
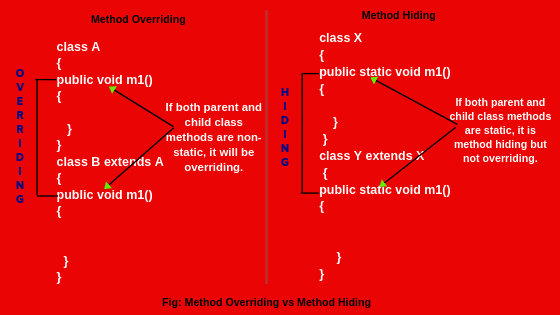
public static double calculateArea(double length, double width) {
return length * width;
}
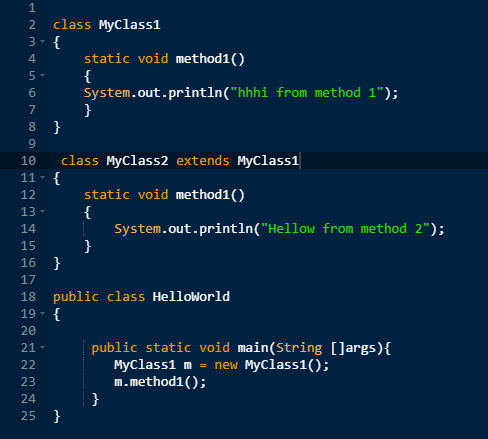
}
Now, let's say we have another class called "Rectangle" that extends from "Calculator". You might think that you can override the "calculateArea" method in the "Rectangle" class to provide a more specific implementation for rectangles.
public class Rectangle extends Calculator {
@Override
public static double calculateArea(double length, double width) {
// new implementation for calculating area of rectangles
return (length + width) * 2; // assuming this is the correct formula
}
}
However, when you try to compile this code, you'll get an error message saying that "static method cannot be overridden". This is because static methods are essentially "class-level" methods, and they're not associated with any specific instance of the class.
In contrast, instance methods (which are non-static) are bound to a particular object. When you override an instance method in a subclass, you're essentially providing a new implementation for that method that's unique to instances of the subclass. This works because each object has its own set of attributes and state, so the overridden method can take those into account.
On the other hand, static methods don't have access to any specific object's state or attributes. They operate at the class level, without knowing which instance is currently being used. Therefore, you cannot provide a new implementation for a static method in a subclass, as it would require accessing the state of an instance that doesn't exist.
In summary, static members (methods and variables) are bound to the class itself, not instances of the class. This means they cannot be overridden in subclasses, as there is no instance-specific context or state to consider.