How to create custom object in Java?
How to create custom object in Java?
I'd be happy to help!
Creating a custom object in Java is an essential skill for any programmer. Here's how you can do it:
What is a Custom Object?
In Java, a custom object is a class that you define yourself, as opposed to the built-in classes provided by the language (e.g., String
, Integer
, etc.). A custom object can represent anything from a simple data structure to a complex business entity. It's essentially a blueprint for creating objects that encapsulate your own data and behavior.
How to Create a Custom Object?
To create a custom object, you define a class using the class
keyword. Here's a step-by-step guide:
public class
syntax, followed by the name of your custom object (e.g., Person
, Car
, etc.). The access modifier (public
) determines the scope of your class; in this case, it means that any part of your program can access the class.
public class Person {
// Class body will go here
}
Properties: Define properties (also known as attributes or fields) to store data specific to your custom object. These are the variables that make up the object's state. For example, if you're creating a Person
class, you might have properties like name
, age
, and address
.
public class Person {
private String name;
private int age;
private Address address;
// Constructor and other methods will go here
}
Constructors: A constructor is a special method that gets called when an object of your custom class is created. Its purpose is to initialize the object's properties with default values or values provided by the programmer.
public class Person {
private String name;
private int age;
private Address address;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Methods: These are blocks of code that perform specific actions related to your custom object. Methods can take arguments (inputs), return values, or both.
public class Person {
// ...
public void greet() {
System.out.println("Hello, my name is " + name + ".");
}
}
Access Modifiers: Control access to your custom object's properties and methods using access modifiers (e.g., public
, private
, protected
). These determine where in your program the class can be accessed.
public class Person {
private String name;
public int getAge() {
return age;
}
// ...
}
Putting it all Together
Now that we've covered the basics, let's create a simple Person
class as an example:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void greet() {
System.out.println("Hello, my name is " + name + ".");
}
}
Using Your Custom Object
Once you've defined your custom object, you can use it in your program. Here's how:
public class Main {
public static void main(String[] args) {
Person person1 = new Person("John", 30);
Person person2 = new Person("Jane", 25);
person1.greet(); // Output: Hello, my name is John.
person2.greet(); // Output: Hello, my name is Jane.
}
}
Conclusion
Creating a custom object in Java involves defining a class with properties, constructors, and methods. By following these steps, you can create reusable code that encapsulates your own data and behavior. This is an essential skill for any Java programmer.
That's all! I hope this helps you get started with creating custom objects in Java.
How to create a java object example w3schools
I'd be happy to help!
In Java, an object is created using the new
keyword followed by the name of the class and any required parameters. This process is called instantiation or object creation.
For example, let's say we have a class called "Car" with two attributes: "color" and "make". Here's how you can create a Car object:
public class Car {
private String color;
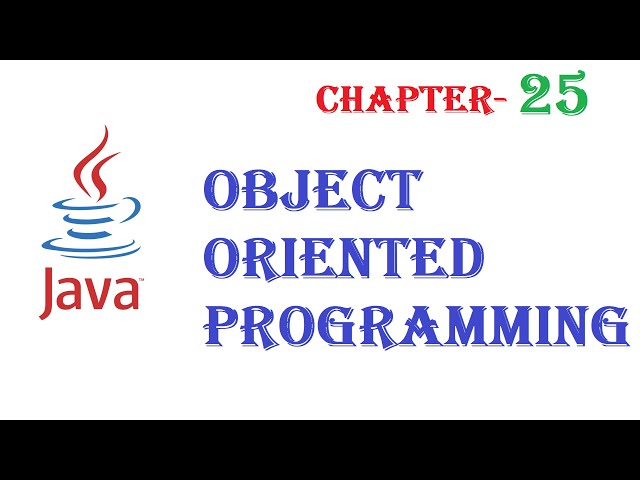
private String make;
public Car(String color, String make) {
this.color = color;
this.make = make;
}
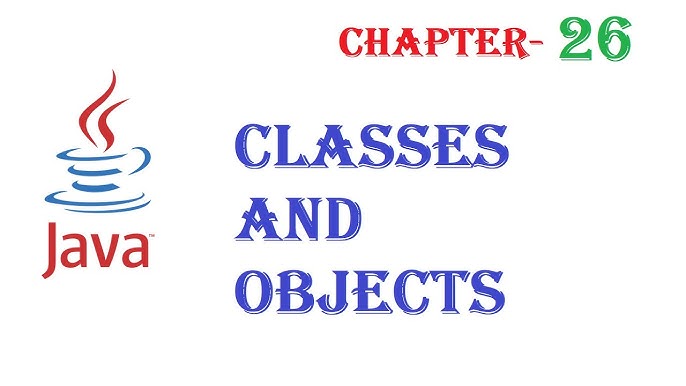
// getters and setters for the attributes
}
To create an instance of the Car class, you would do something like this:
Car myCar = new Car("Red", "Toyota");
In this example, we are creating a new object called myCar
that has a color of "Red" and a make of "Toyota". We can then use the attributes and methods of the Car class to interact with our new object.
Here's an example of how you might use these attributes:
System.out.println("My car is a " + myCar.getMake() + " that is " + myCar.getColor());
This would print out: "My car is a Toyota that is Red".
If you want to create multiple objects, simply make more instances:
Car yourCar = new Car("Blue", "Ford");
System.out.println("Your car is a " + yourCar.getMake() + " that is " + yourCar.getColor());
This would print out: "Your car is a Ford that is Blue".
You can access the attributes of an object using the dot notation (object.attribute). For example:
String myColor = myCar.getColor();
System.out.println("My color is " + myColor);
This would print out: "My color is Red".
Remember to always follow proper Java naming conventions, such as starting class names with uppercase letters and attribute names with lowercase letters. This will help you keep your code clean and easy to read.
For more information on Java, please visit W3Schools.com for tutorials, examples, and reference materials!