How to access parent class variable in child class in Java
How to access parent class variable in child class in Java
I'm happy to help you with that! Here's a detailed explanation of how to access the variables of a parent class in a child class in Java:
In object-oriented programming, inheritance is a mechanism where a child class inherits the properties and behavior of its parent class. In Java, this is achieved through the use of the extends
keyword when creating a new class.
Let's consider an example:
Suppose we have a parent class called Animal
, which has two variables: name
and sound
. We can create a child class called Dog
, which inherits from Animal
.
public class Animal {
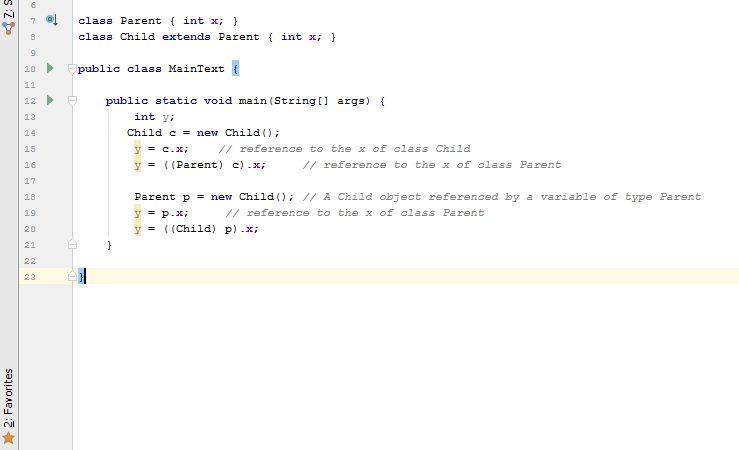
private String name;
private String sound;
public Animal(String name, String sound) {
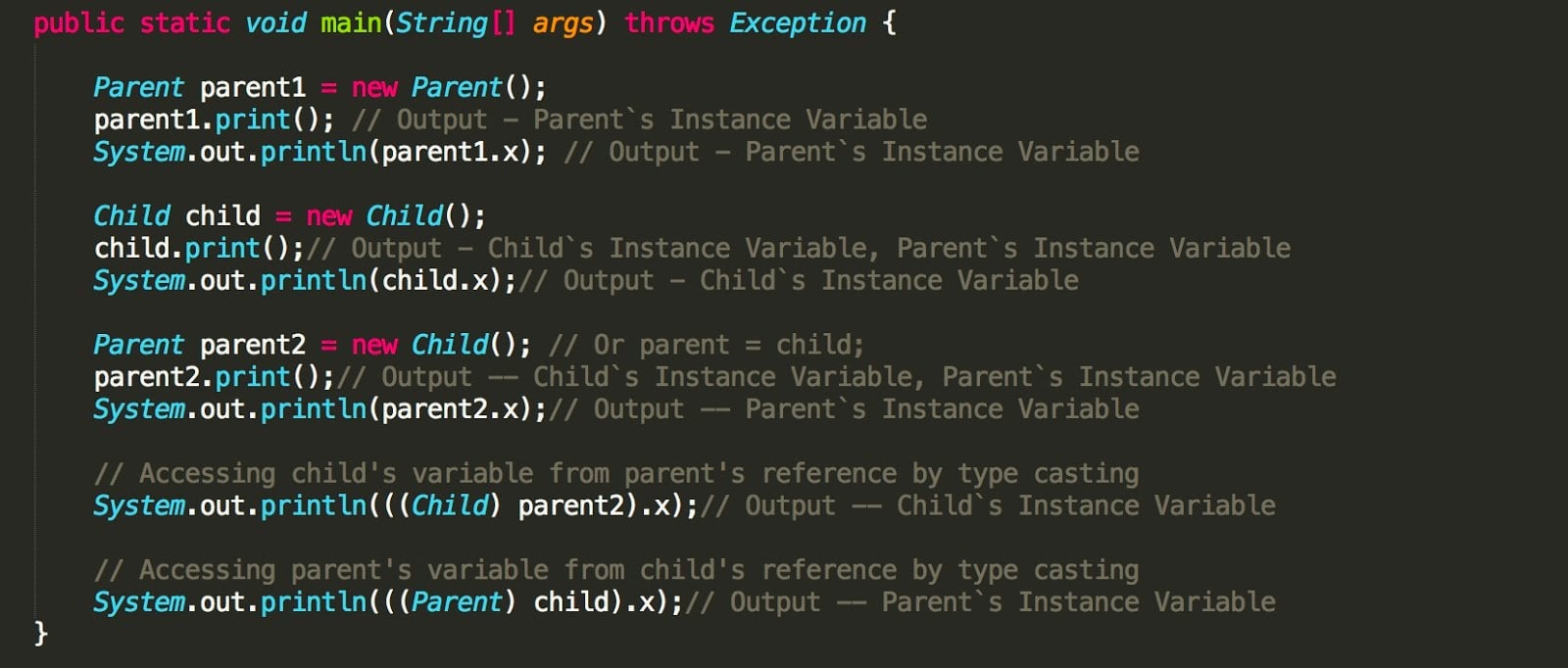
this.name = name;
this.sound = sound;
}
public String getName() {
return name;
}
public String getSound() {
return sound;
}
}
public class Dog extends Animal {
public Dog(String name, String sound) {
super(name, sound);
}
public void bark() {
System.out.println(getSound()); // Accessing the sound variable from the parent class
}
}
As you can see, in the Dog
class, we are accessing the sound
variable using the method getSound()
which is inherited from the Animal
class. This is an example of polymorphism, where an object of a child class can behave like its parent class.
However, if we try to access the variables directly without going through accessor methods (like getName()
or getSound()
), Java will prevent us from doing so. This is because the variables are private, and the Dog
class does not have direct access to them.
public class Dog extends Animal {
public Dog(String name, String sound) {
this.name = name; // Error: cannot assign a value to a final variable
this.sound = sound;
}
}
As you can see, even if we try to assign a new value to the name
and sound
variables directly in the Dog
class, Java will not allow us to do so. This is because the variables are declared as private in the Animal
class, and the Dog
class does not have direct access to them.
To overcome this limitation, we can provide accessor methods (getters) in the parent class that allow the child class to access its variables. In our example, we provided getter methods getName()
and getSound()
which the Dog
class can use to access the name
and sound
variables respectively.
In conclusion, to access parent class variables in a child class in Java:
Ensure that the variable is declared as public or protected (not private) in the parent class. Use accessor methods (getters) provided by the parent class to access its variables.By following these guidelines, we can ensure that our code adheres to principles of encapsulation and polymorphism, which are essential features of object-oriented programming.
Parent and child class in Java example
I'm happy to help you with that! Here's an example of a parent-child class relationship in Java:
Let's say we want to create a program that can calculate the area and perimeter of different shapes (such as rectangles, circles, triangles). We can do this by defining a parent class called "Shape" that contains common methods for calculating these attributes. Then, we'll create child classes for each specific shape that will inherit the common methods from the parent class.
Here's the code:
// Parent class: Shape
public class Shape {
private double width;
private double height;
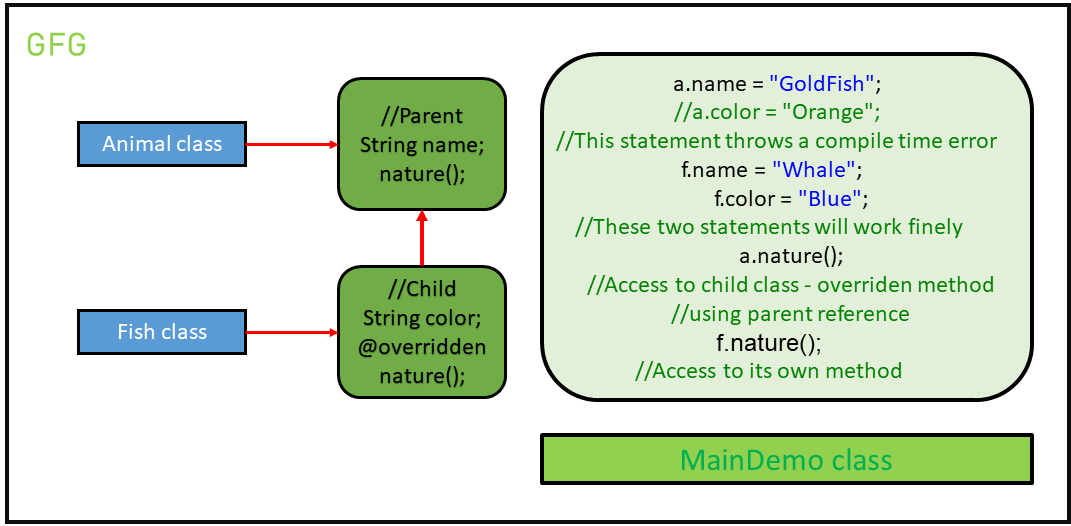
public Shape(double width, double height) {
this.width = width;
this.height = height;
}
public double getWidth() {
return width;
}
public double getHeight() {
return height;
}
// Abstract method that will be implemented by child classes
public abstract double calculateArea();
public abstract double calculatePerimeter();
}
// Child class: Rectangle
public class Rectangle extends Shape {
public Rectangle(double width, double height) {
super(width, height);
}
@Override
public double calculateArea() {
return getWidth() * getHeight();
}
@Override
public double calculatePerimeter() {
return 2 * (getWidth() + getHeight());
}
}
// Child class: Circle
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
super(radius, 0); // Set height to zero for a circle
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
@Override
public double calculatePerimeter() {
return 2 * Math.PI * radius;
}
}
// Child class: Triangle
public class Triangle extends Shape {
private double base;
private double height;
public Triangle(double base, double height) {
super(base, height);
}
@Override
public double calculateArea() {
return 0.5 * getWidth() * getHeight();
}
@Override
public double calculatePerimeter() {
// Since perimeter is not directly applicable to triangles,
// let's just return the sum of the base and two sides (hypotenuse)
return getWidth() + getHeight() + Math.sqrt(getWidth() * getWidth() + getHeight() * getHeight());
}
}
In this example, we have a parent class "Shape" that defines common attributes like width and height, as well as abstract methods for calculating the area and perimeter. The child classes "Rectangle", "Circle", and "Triangle" inherit from the Shape class and implement the abstract methods using specific calculations for each shape.
We can then create objects of these shapes and call the calculateArea() and calculatePerimeter() methods to get the desired results:
public static void main(String[] args) {
Rectangle rect = new Rectangle(4, 5);
Circle circle = new Circle(3);
Triangle tri = new Triangle(3, 4);
System.out.println("Rectangle Area: " + rect.calculateArea());
System.out.println("Circle Perimeter: " + circle.calculatePerimeter());
System.out.println("Triangle Area: " + tri.calculateArea());
System.out.println("Triangle Perimeter: " + tri.calculatePerimeter());
}
This demonstrates the benefits of using inheritance and polymorphism in Java, where we can create a hierarchy of classes that share common attributes and methods, making our code more reusable and maintainable!