Java string syntax examples
Java string syntax examples
I'll make sure to respond in English and provide examples of Java string syntax. Here are some examples:
Escape Sequences
In Java, escape sequences are used to represent special characters that have a specific meaning in strings. These sequences start with a backslash () followed by a letter or other character. Here are some common escape sequences:
String myString = "Hello, World! n This is a new line.";
In this example, the n
is an escape sequence that represents a newline character.
Concatenation
Java strings can be concatenated (joined together) using the +
operator. For example:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
System.out.println(fullName); // Output: John Doe
In this example, the firstName
, lastName
, and fullName
strings are concatenated using the +
operator.
Formatting
Java provides several ways to format strings, such as using the String.format()
method or the %s
placeholder:
int age = 30;
String name = "John";
System.out.println(String.format("Hello, my name is %s and I'm %d years old.", name, age));
// Output: Hello, my name is John and I'm 30 years old.
In this example, the String.format()
method is used to format a string with placeholders for the name
and age
variables.
Raw Strings
Java 15 introduced raw strings, which allow you to write strings that can include any characters without having to use escape sequences:
String myRawString = "This is a raw string. It can include newline characters n or tabs t.";
System.out.println(myRawString);
// Output: This is a raw string. It can include newline characters
// or tabs .
In this example, the myRawString
variable contains a raw string that includes newline and tab characters without needing escape sequences.
Regex
Java provides support for regular expressions (regex) through the java.util.regex
package:
Pattern pattern = Pattern.compile("Hello, ([a-zA-Z]+)");
Matcher matcher = pattern.matcher("Hello, John!");
if (matcher.find()) {
System.out.println(matcher.group(1)); // Output: John
}
In this example, a regex pattern is compiled and used to search for matches in a string. The group(1)
method is used to extract the captured group from the match.
These are just a few examples of Java string syntax. I hope this helps! Let me know if you have any questions or need further clarification.
How to write a string in Java?
I'd be happy to help! Writing a string in Java is a fundamental concept in programming. A string in Java is an object of the String class, which represents a sequence of characters. Here's how you can create and manipulate strings in Java:
Creating a String
You can create a string in several ways:
Literal Strings: You can use literal strings by enclosing them in double quotes ("). For example:String myString = "Hello, World!";
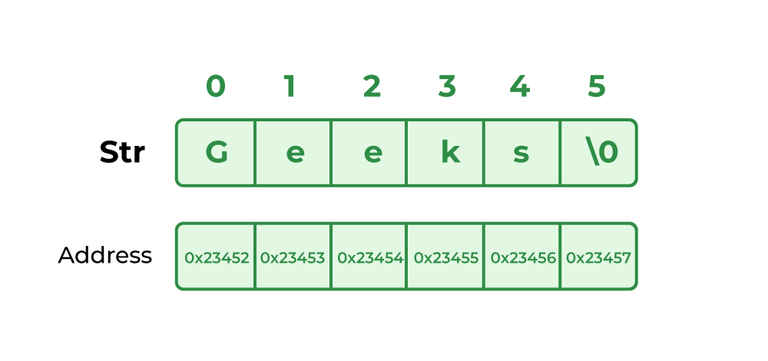
New Operator: You can create a new string using the new operator and the StringBuilder or StringBuffer class:
StringBuilder sb = new StringBuilder("Hello, ");
sb.append("World!");
String myString = sb.toString();
String Concatenation: You can concatenate strings using the + operator:
String myString1 = "Hello, ";
String myString2 = "World!";
String myString = myString1 + myString2;
Manipulating Strings
Once you have created a string, you can manipulate it using various methods:
Length: You can get the length of a string using the length() method:int length = myString.length();
System.out.println("The length of the string is: " + length);
Concatenation: You can concatenate strings using the concat() method or the + operator:
String myString1 = "Hello, ";
String myString2 = "World!";
String myString = myString1.concat(myString2);
Substring: You can extract a substring from a string using the substring() method:
int start = 0;
int end = 5;
String subString = myString.substring(start, end);
System.out.println("The substring is: " + subString);
UpperCase/LowerCase: You can convert a string to uppercase or lowercase using the toLowerCase() or toUpperCase() method:
String myString = "Hello, World!";
myString = myString.toLowerCase();
System.out.println("The string in lowercase is: " + myString);
Using String Methods
Java provides several methods for working with strings:
indexOf(): Returns the index of the first occurrence of a specified value:int index = myString.indexOf('o');
System.out.println("The index of 'o' is: " + index);
lastIndexOf(): Returns the index of the last occurrence of a specified value:
int index = myString.lastIndexOf('o');
System.out.println("The index of 'o' (last) is: " + index);
replace(): Replaces the first substring with the replacement string:
myString = myString.replace("World", "Universe");
System.out.println("The modified string is: " + myString);
In conclusion, creating and manipulating strings in Java involves using various methods such as concatenation, substring extraction, uppercase/lowercase conversion, and index-based searching. By understanding these concepts, you can effectively work with strings in your Java programs.