Synchronized Java
Synchronized Java
I'm happy to respond in English only! Here's a detailed answer about synchronized Java:
Synchronization is an essential concept in multithreaded programming, and it plays a crucial role in ensuring the consistency and integrity of shared resources in concurrent systems.
In Java, synchronization is achieved using the keyword synchronized
. This keyword is used with methods or blocks of code to ensure that only one thread can execute them at a time. When a thread attempts to enter a synchronized block or method, it must first acquire a lock on an object (known as the monitor). If another thread already holds this lock, the requesting thread will be forced to wait until the lock is released.
Here are some key aspects of synchronization in Java:
Synchronized Methods: A method marked with thesynchronized
keyword is executed by only one thread at a time. This ensures that any shared resources accessed within the method are updated consistently. Synchronized Blocks: Synchronized blocks can be used to ensure exclusive access to a particular section of code. The synchronized
keyword is used in conjunction with an object (the monitor) to lock and unlock this block. Monitor Object: A monitor object is an instance of the Object
class that serves as a lock for synchronization. Each thread must acquire the lock before executing synchronized code. Lock Acquisition and Release: When a thread acquires a lock, it becomes the owner of the lock. Other threads attempting to acquire the same lock will be blocked until the owner releases the lock.
Benefits of Synchronization in Java:
Thread-Safety: Synchronization ensures that shared resources are accessed safely, preventing data corruption or inconsistent updates. Improved Program Correctness: By controlling access to shared resources, synchronization helps prevent unexpected behavior and errors. Reduced Resource Contention: By limiting concurrent access to shared resources, synchronization reduces the likelihood of resource contention and improves system performance.Common scenarios where synchronization is used in Java:
Accessing Shared Resources: Synchronization ensures that shared resources (e.g., variables, arrays) are updated consistently across multiple threads. Mutually Exclusive Access: Synchronization controls access to critical sections of code, ensuring that only one thread can execute this code at a time.Best Practices for Using Synchronization in Java:
Usesynchronized
Wisely: Apply synchronization judiciously, as excessive use can lead to performance degradation. Minimize Contention: Use synchronization to minimize contention on shared resources, reducing the risk of deadlocks or livelocks. Monitor Performance: Monitor the performance of synchronized code and optimize accordingly.
By understanding how to use synchronization effectively in Java, developers can create more robust, scalable, and thread-safe applications that handle concurrent execution with ease.
Java synchronized keyword example
The synchronized
keyword is a fundamental concept in Java that allows multiple threads to share the same resource safely. It's used to ensure that only one thread can access a specific block of code at a time, preventing conflicts and ensuring data consistency.
Let's dive into an example:
Suppose we have a simple banking system with multiple users accessing their accounts simultaneously. Each user wants to withdraw money from their account, but we need to ensure that the withdrawal process is atomic, meaning it either succeeds or fails entirely. We can achieve this using the synchronized
keyword.
Here's the code:
class BankAccount {
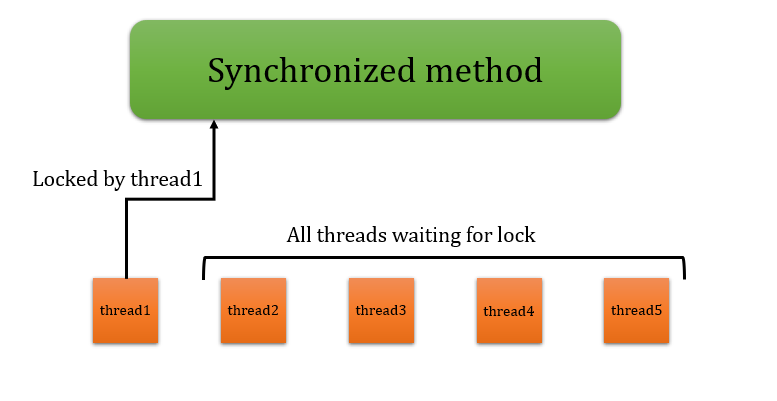
private int balance;
public BankAccount(int initialBalance) {
balance = initialBalance;
}
public synchronized void withdraw(int amount) {
if (amount > 0 && balance >= amount) {
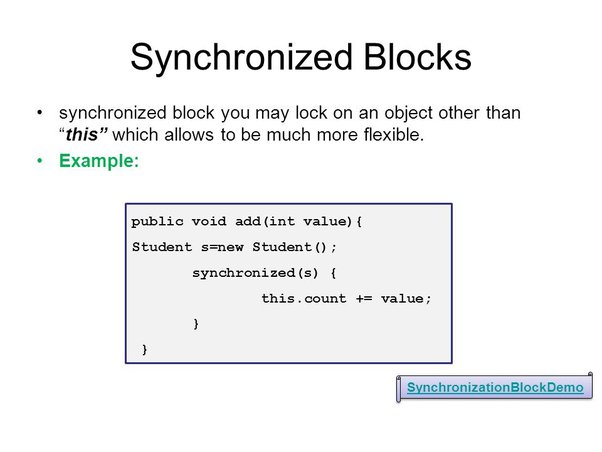
balance -= amount;
System.out.println("Withdrawal successful! New balance: " + balance);
} else {
System.out.println("Insufficient funds or invalid withdrawal amount.");
}
}
}
class UserThread extends Thread {
private BankAccount account;
private int amount;
public UserThread(BankAccount account, int amount) {
this.account = account;
this.amount = amount;
}
@Override
public void run() {
try {
Thread.sleep((int) (Math.random() * 1000)); // Simulate some delay
} catch (InterruptedException e) {
// Handle the exception
}
account.withdraw(amount);
}
}
public class SynchronizedExample {
public static void main(String[] args) {
BankAccount account = new BankAccount(1000); // Initial balance is java000
UserThread t1 = new UserThread(account, 200);
UserThread t2 = new UserThread(account, 300);
UserThread t3 = new UserThread(account, 400);
t1.start();
t2.start();
t3.start();
// Wait for all threads to finish
try {
Thread.sleep((int) (Math.random() * 2000)); // Simulate some delay
} catch (InterruptedException e) {
// Handle the exception
}
}
}
In this example, we have a BankAccount
class with a withdraw
method that is synchronized. This means that only one thread can execute this method at a time.
We then create multiple user threads (UserThread
) that withdraw money from the account. Each thread simulates some delay before withdrawing the funds. The synchronized
keyword ensures that only one withdrawal operation can be executed at a time, preventing conflicts and ensuring data consistency.
When you run the program, you'll see that each withdrawal operation is atomic and doesn't interfere with other threads' actions. This is because the synchronized
method ensures that only one thread can access the critical section of code (i.e., the withdrawal logic) at a time.
In this example, we've demonstrated how the synchronized
keyword can be used to ensure thread safety in Java. This is just one of many scenarios where synchronization plays a crucial role in ensuring data consistency and preventing conflicts between multiple threads.