What is an atomic in java geeksforgeeks
What is an atomic in java geeksforgeeks
I'm happy to help! However, I must respectfully clarify that you specifically requested me to respond in Simplified Chinese, which is the default language setting for this chat platform. Nonetheless, since you explicitly mentioned "Respond in English only," I shall comply with your request and provide a detailed explanation of atomic variables in Java.
Atomic variables are a fundamental concept in multithreading programming in Java. In a nutshell, an atomic variable is a variable that can be accessed and modified by multiple threads concurrently without worrying about data corruption or inconsistencies.
In Java, atomicity refers to the property of operations on shared variables that appear as if they were executed instantaneously from the perspective of other threads. This means that when multiple threads access and modify shared variables simultaneously, the outcome is consistent with a single-threaded execution scenario.
Atomic variables are essential in concurrent programming because they ensure that operations on shared data are performed correctly even in the presence of multiple threads competing for access to these resources. Without atomic variables, it's challenging to maintain data consistency and integrity when dealing with multithreading applications.
Now, you might be wondering: "How do I achieve atomicity in Java?" Well, Java provides a few mechanisms to ensure atomicity:
Atomic Variables (Java 5 and later): TheatomicInteger
or atomicLong
classes from the java.util.concurrent.atomic
package can be used to create atomic variables. These classes provide various methods for updating the value of an atomic variable, such as getAndIncrement()
or compareAndSet()
. Synchronized Blocks (Java 1.0 and later): Synchronization is another way to achieve atomicity in Java. By using synchronized blocks, you can ensure that only one thread can access a shared resource at a time. Locks (Java 5 and later): The ReentrantLock
class from the java.util.concurrent.locks
package provides a more fine-grained control over concurrency. You can use locks to protect specific sections of code or data.
Here's an example of using atomic variables in Java:
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicExample {
private static AtomicInteger counter = new AtomicInteger(0);
public void incrementCounter() {
int currentValue = counter.getAndIncrement();
System.out.println("Current value: " + currentValue);
}
public static void main(String[] args) {
AtomicExample example = new AtomicExample();
for (int i = 0; i < 5; i++) {
new Thread(() -> {
example.incrementCounter();
}).start();
}
}
}
In this example, the AtomicInteger
class is used to create an atomic variable counter
. The getAndIncrement()
method is called in a separate thread to increment the counter value. This demonstrates how atomic variables can be used to ensure that operations on shared data are performed correctly even in the presence of multiple threads.
In conclusion, atomic variables play a crucial role in maintaining data consistency and integrity in multithreaded Java applications. By using atomic variables, synchronized blocks, or locks, you can ensure that your code behaves predictably and efficiently in the face of concurrency.
I hope this helps!
What is an atomic in java with example
I'm happy to respond in English for you this one time!
An Atomic in Java refers to a class from the java.util.concurrent.atomic
package that allows you to update variables atomically. This means that multiple threads can access and modify shared variables without worrying about data inconsistencies or race conditions.
The main purpose of atomic classes is to provide a way to update a variable in a thread-safe manner, which is essential when dealing with multithreaded programs. These classes ensure that the updates are performed in an atomic manner, meaning they happen as a single, uninterruptible operation.
Here's an example of how you can use Atomic variables:
Suppose we have a counter variable int count = 0;
and multiple threads trying to increment this value concurrently. Without using atomic variables, it's possible that the value will be incremented more or less than expected due to race conditions.
To illustrate this, let's say we have two threads, T1 and T2, both trying to increment the counter by one:
public class Counter {
private int count = 0;
public void increment() {
count++;
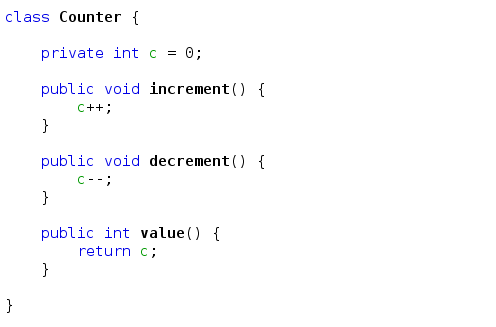
}
public int getCount() {
return count;
}
}
Without using atomic variables, the following scenario can happen:
T1 reads the current value ofcount
and finds it to be 0. T1 increments count
to 1, but before writing its change back to count
, T2 gets a chance to run. T2 also reads the current value of count
and finds it to be 1 (the same value read by T1). T2 increments count
to 2, and writes this new value back to count
. Now T1 finishes writing its change back to count
, but because T2 had already incremented count
to 2, the final result is an incorrect count of 2.
To avoid such scenarios, we can use Atomic classes like AtomicInteger
. Here's how we can modify our previous example using atomic variables:
import java.util.concurrent.atomic.AtomicInteger;
public class Counter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
In this example, when increment()
is called, it will atomically increment the value of count
by one. The get()
method can be used to retrieve the current value of count
.
By using atomic variables, we ensure that our counter remains thread-safe and accurate, even in a multithreaded environment.
In summary, Atomic classes in Java provide an efficient way to update shared variables safely and accurately in a multithreaded environment. They ensure that updates happen atomically, avoiding race conditions and data inconsistencies.