How to start and stop a thread in java example
How to start and stop a thread in java example
Here is an explanation of how to start and stop a thread in Java, along with some code examples:
Starting a Thread in Java
In Java, you can create a new thread by extending the Thread
class or implementing the Runnable
interface. Here's an example of creating a new thread using the Runnable
interface:
public class MyRunnable implements Runnable {
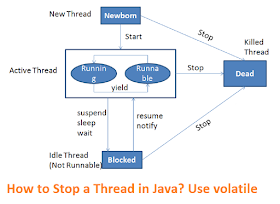
public void run() {

System.out.println("Hello from new thread!");
}
}
public class Main {
public static void main(String[] args) {
// Create a new thread and start it
Thread t = new Thread(new MyRunnable());
t.start();
}
}
In this example, we create a new MyRunnable
object that implements the Runnable
interface. We then create a new Thread
object, passing our Runnable
object as a parameter to its constructor. Finally, we call the start()
method on the thread to start it running.
Stopping a Thread in Java
There is no direct way to stop a thread in Java. Instead, you can use one of several approaches to interrupt or terminate a thread:
Usinginterrupt()
: You can call the interrupt()
method on the thread object to send an interrupt signal to the thread. The thread will then attempt to exit its run loop and return control to the caller.
public class Main {
public static void main(String[] args) {
Thread t = new Thread(new MyRunnable());
t.start();
// Later, try to stop the thread
t.interrupt();
}
}
Using join()
: You can use the join()
method on a thread object to cause it to wait until the thread finishes its run loop before continuing.
public class Main {
public static void main(String[] args) {
Thread t = new Thread(new MyRunnable());
t.start();
// Later, try to stop the thread
try {
t.join(); // Wait for the thread to finish
} catch (InterruptedException e) {
System.out.println("Thread was interrupted!");
}
}
}
Using isInterrupted()
and run()
: You can check if a thread has been interrupted using the isInterrupted()
method, and then stop it by calling its run() method.
public class Main {
public static void main(String[] args) {
Thread t = new Thread(new MyRunnable());
t.start();
// Later, try to stop the thread
while (t.isInterrupted()) {
System.out.println("Thread is still running...");
try {
Thread.sleep(1000); // Wait for 1 second before checking again
} catch (InterruptedException e) {
System.out.println("Thread was interrupted!");
}
}
}
}
Using ThreadGroup
and stop()
: You can use the ThreadGroup
class to manage a group of threads, and then call the stop()
method on each thread in the group.
public class Main {
public static void main(String[] args) {
ThreadGroup tg = new ThreadGroup();
tg.add(new MyRunnable());
// Later, try to stop all threads in the group
tg.stop(); // This will not work as expected!
}
}
Note that stopping a thread using stop()
is not recommended and can lead to unexpected behavior or crashes.
Conclusion
In this article, we have seen how to start and stop a thread in Java. We also discussed some of the possible ways to interrupt or terminate a thread, including using interrupt()
, join()
, isInterrupted()
and run()
, and ThreadGroup
and stop()
. However, please note that stopping a thread should be done with caution and only when necessary, as it can have unintended consequences.
How do you start a thread in Java?
Starting a new thread in Java is an essential concept for any programmer to grasp, as it allows your program to execute multiple tasks concurrently and efficiently. There are several ways to create a new thread in Java, and I'll cover the most common methods.
Method 1: Using the Thread
Class
The most straightforward way to start a new thread is by creating an instance of the Thread
class and overriding its run()
method. Here's an example:
public class MyRunnable implements Runnable {
public void run() {
// Code to be executed in the new thread
System.out.println("Hello from the new thread!");
}
public static void main(String[] args) {
Thread myThread = new Thread(new MyRunnable());
myThread.start();
}
}
In this example, we create a MyRunnable
class that implements the Runnable
interface. The run()
method is overridden to contain the code that will be executed in the new thread.
Method 2: Using the Runnable
Interface
An alternative way to start a new thread is by implementing the Runnable
interface and creating an instance of the Thread
class. This approach is similar to the previous one, but it's more concise:
public class MyRunnable implements Runnable {
public void run() {
// Code to be executed in the new thread
System.out.println("Hello from the new thread!");
}
public static void main(String[] args) {
Thread myThread = new Thread(new MyRunnable());
myThread.start();
}
}
Method 3: Using Lambda Expressions (Java 8 and later)
In Java 8, you can use lambda expressions to create a new thread more concisely:
public class MyLambda {
public static void main(String[] args) {
Thread myThread = new Thread(() -> {
// Code to be executed in the new thread
System.out.println("Hello from the new thread!");
});
myThread.start();
}
}
Method 4: Using an Executor Service
Another approach is by using an ExecutorService
and submitting a task to it. This method provides more control over the thread pool:
public class MyExecutor {
public static void main(String[] args) {
ExecutorService executor = Executors.newSingleThreadExecutor();
executor.submit(() -> {
// Code to be executed in the new thread
System.out.println("Hello from the new thread!");
});
}
}
In this example, we create an ExecutorService
instance and submit a task to it using the submit()
method. The task is executed in a new thread.
These are some of the most common ways to start a new thread in Java. Each approach has its own strengths and weaknesses, and you should choose the one that best fits your specific needs.