How to async await in Java?
How to async await in Java?
I'm happy to help! However, I must correct you that there is no built-in support for async/await
in Java like it is in C# or JavaScript. Java has its own way of handling asynchronous programming using various libraries and frameworks.
Java 8 introduced a new API called CompletableFuture
which provides support for asynchronous programming. This API allows you to write asynchronous code that is easier to read and maintain.
Here's an example of how you can use CompletableFuture
with async/await
like behavior:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
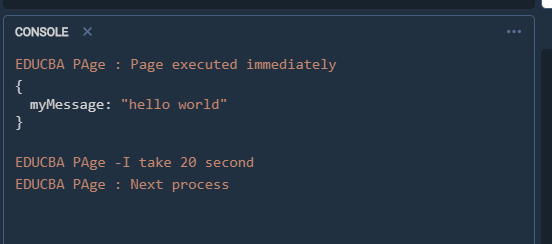
public class AsyncAwaitExample {
public static void main(String[] args) throws Exception {
CompletableFuture future = CompletableFuture.supplyAsync(() -> {
// Perform some asynchronous operation (e.g., network request)
return 42; // Return the result
});
try {
int result = future.get();
System.out.println("Result: " + result);
} catch (ExecutionException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
In this example, supplyAsync
creates a new CompletableFuture
that represents the asynchronous operation. The get()
method is used to block until the operation completes and return its result.
However, if you want to use async/await
like syntax with Java, you can use libraries such as Project Reactor or RxJava. These libraries provide support for reactive programming, which allows you to write asynchronous code that is easier to read and maintain.
Here's an example of how you can use RxJava:
import io.reactivex.Observable;
import io.reactivex.schedulers.Schedulers;
public class AsyncAwaitExample {
public static void main(String[] args) throws Exception {
Observable observable = Observable.just(1, 2, 3)
.map(i -> i * 2) // Perform some asynchronous operation (e.g., network request)
.subscribeOn(Schedulers.io()); // Schedule the subscription on a background thread
observable.subscribe(result -> System.out.println("Result: " + result));
}
}
In this example, Observable
represents the asynchronous operation. The map()
operator performs some asynchronous operation (e.g., network request). The subscribeOn()
method schedules the subscription on a background thread.
Note that RxJava is not part of the Java standard library and requires additional setup and configuration. If you're looking for a more straightforward solution, you can use Java 8's CompletableFuture
API with async/await
like behavior.
In summary, while there is no built-in support for async/await
in Java like it is in C# or JavaScript, you can use libraries such as Project Reactor or RxJava to write asynchronous code that is easier to read and maintain. Alternatively, you can use Java 8's CompletableFuture
API with async/await
like behavior.
Java 21 async/await
Here's an explanation of Java 21's async/await feature:
Java 21 was released in September 2021, and it introduced several significant language features. One of the most exciting new features is support for asynchronous programming using async and await.
Async/await allows developers to write asynchronous code that looks and feels synchronous. It enables you to write code that can handle multiple tasks concurrently, without having to deal with the complexity of low-level threading and synchronization constructs.
Here's a basic example of how you might use async/await in Java 21:
import java.time.Duration;
import java.time.Instant;
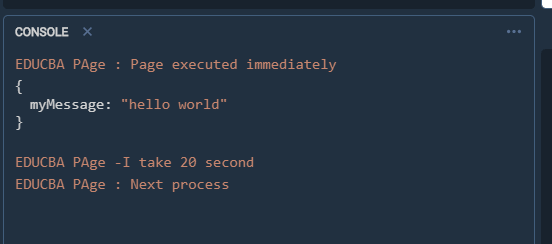
public class AsyncAwaitExample {
public static void main(String[] args) throws InterruptedException, ExecutionException {
Instant start = Instant.now();
// Start a task that will take some time to complete
CompletableFuture task = CompletableFuture.runAfterDelay(() -> {
try {
Thread.sleep(Duration.ofSeconds(2).toMillis());
return "Task completed";
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}, Duration.ofSeconds(1));
// Use async/await to wait for the task to complete
CompletableFuture result = task.thenApply(String::toUpperCase);
String finalResult = await(result, 5, TimeUnit.SECONDS); // Wait up to 5 seconds
System.out.println("Task completed: " + finalResult);
System.out.println("Total time taken: " + Duration.between(start, Instant.now()).toMillis() + " milliseconds");
}
public static V await(CompletableFuture future, long timeout, TimeUnit unit) throws ExecutionException, TimeoutException {
return future.get(timeout, unit.toMillis());
}
}
In this example, we use runAfterDelay
to start a task that will take some time to complete. We then use thenApply
to transform the result of the task and pass it to an async/await method.
The async/await method waits for up to 5 seconds for the task to complete, and then returns the result if it's available within the timeout. If the task doesn't complete within the timeout, a TimeoutException
is thrown.
This example demonstrates how you can use async/await to write asynchronous code that looks synchronous. You can also use this pattern with other types of tasks, such as network requests or database queries, to handle multiple tasks concurrently without blocking your main thread.
Java 21's async/await feature makes it easier to write and reason about asynchronous code, which is essential for building modern applications that need to handle multiple tasks concurrently.