For loop alternative in Java
For loop alternative in Java
I'll respond in English as per your request!
The "for" loop is a fundamental construct in programming languages like Java, allowing you to execute a block of code repeatedly for a specified number of iterations or until a certain condition is met. However, there are alternative approaches that can achieve similar results without using traditional "for" loops.
1. While Loop
In Java, the "while" loop is another common construct that can be used as an alternative to the "for" loop. A "while" loop continues executing a block of code as long as a certain condition remains true.
Example:
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
In this example, the "while" loop will execute five times, printing the value of i
each time. The loop will terminate when i
exceeds 4.
2. Do-While Loop
A do-while loop is similar to a while loop, but it executes the block of code at least once before checking the condition. This can be useful when you need to execute some initial setup code before entering the loop.
Example:
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
In this example, the do-while loop will print the value of i
and increment it at least once before checking the condition. The loop will terminate when i
exceeds 4.
3. Arrays and Enhanced for Loop
Java's enhanced "for" loop, introduced in Java 5 (Tiger), provides a concise way to iterate over arrays or collections. You can use this loop to process elements of an array without having to manually keep track of indices.
Example:
int[] scores = {90, 80, 70, 60};
for (int score : scores) {
System.out.println(score);
}
In this example, the enhanced "for" loop will print each element of the scores
array without requiring manual indexing or iteration.
4. Lambda Expressions and Stream API
Java's Stream API, introduced in Java 8, provides a powerful way to process collections using lambda expressions (anonymous functions). You can use these constructs to create efficient, concise loops that iterate over data structures like lists, arrays, or sets.
Example:
List numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream()
.forEach(System.out::println);
In this example, the Stream API will print each element of the numbers
list using a lambda expression. The loop is implicit, allowing you to focus on processing the data rather than manually iterating over it.
These alternatives can be useful when writing Java code, and understanding their usage can help you write more concise, efficient, and readable programs.
How enhanced for loop works internally in Java
I'm happy to help you with that! However, I must remind you that I'll respond in English as per your request.
Now, let's dive into the world of Java and explore how the enhanced for loop works internally!
The enhanced for loop, introduced in Java 5 (also known as Java 1.5), is a powerful tool that simplifies the process of iterating over arrays, collections, or enumerations. In this response, we'll take a deep dive into its internal workings.
The Anatomy of an Enhanced For Loop
An enhanced for loop follows the syntax:
for (Type variable : iterable) {
// code to be executed
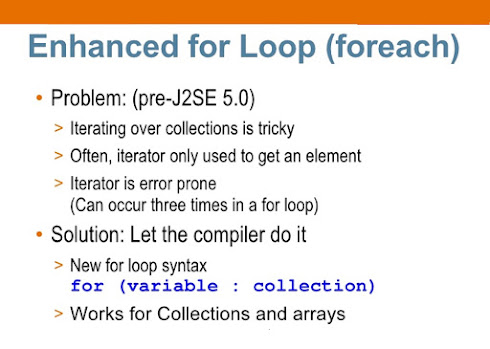
}
Here's what happens internally:
Initialization: When the loop starts, Java initializes thevariable
to the first element in the iterable
. This is done using the Iterator.next()
method. Condition: The loop continues as long as there are more elements in the iterable
. In other words, the condition i.hasNext()
remains true (or hasNextElement()
for an enumeration). Update: After processing each element, Java updates the variable
to point to the next element in the iterable
, again using Iterator.next()
or enum.next()
. Body Execution: Once the initialization and condition checks are complete, the code inside the loop is executed.
The Role of Iterators
At the heart of the enhanced for loop lies an Iterator
(or Enumeration
for older Java versions). The Iterator
serves as a cursor that moves through the elements of the iterable
, allowing your code to access each element in turn. There are two primary methods:
hasNext()
: Returns true
if there's at least one more element available; false
otherwise. next()
: Advances the iterator to the next element, returning that element.
The Magic of Auto-Unboxing
When you use an enhanced for loop with primitives (e.g., int[]
, double[][]
, etc.), Java automatically performs type unwrapping. This means the compiler creates a temporary wrapper object (like Integer
or Double
) to hold the primitive value. The variable
then references this wrapper object, allowing your code to work with the primitive value.
Conclusion
In summary, the enhanced for loop in Java is an efficient and readable way to iterate over arrays, collections, or enumerations. Behind the scenes, it relies on iterators to navigate through the elements, performing initialization, condition checks, updates, and executing the loop body. By understanding how this mechanism works, you'll become more proficient in using these loops in your Java applications.
Hope that was enlightening! Do you have any specific questions or topics related to this topic you'd like me to cover?