Java orm tutorial geeksforgeeks
Java orm tutorial geeksforgeeks
Here is a comprehensive tutorial on Java ORM (Object-Relational Mapping) from GeeksforGeeks:
What is Object-Relational Mapping?
ORM stands for Object-Relational Mapping, which is a programming technique that allows you to interact with relational databases using objects. In other words, it provides a way to map the data in your Java classes (objects) to the tables and rows of a relational database.
Why do we need ORM?
Traditionally, interacting with a relational database involves writing SQL queries to perform CRUD (Create, Read, Update, Delete) operations on the data. While this approach works, it can lead to:
Tight coupling between your Java code and the database schema Inflexibility in case the database schema changes Error-prone code due to manual SQL query constructionORM helps alleviate these issues by providing a layer of abstraction between your Java code and the database.
What are some popular Java ORMs?
Hibernate: One of the most widely used Java ORMs, Hibernate provides a powerful way to map Java classes to relational databases. JPA (Java Persistence API): JPA is a standard ORM specification for Java that allows you to interact with relational databases using a standardized API. iBatis: iBatis is another popular ORM solution that provides a simple and efficient way to interact with relational databases.How does ORMs work?
Here's a high-level overview of how ORMs work:
Define the Java class: Define your Java classes, which represent the data entities you want to store in the database. Map to the database schema: Map each Java class to a specific table or set of tables in the relational database. Create an ORM configuration file: Create a configuration file that specifies how to map the Java classes to the database schema. Use the ORM API: Use the ORM API to perform CRUD operations on the data.Advantages of ORMs
Increased productivity: ORMs simplify the process of interacting with relational databases, making it easier for developers to write code. Improved maintainability: By decoupling your Java code from the database schema, you can modify either without affecting the other. Reduced errors: ORMs help prevent errors by providing a layer of abstraction between your Java code and the database.How to choose an ORM for your project
Assess your requirements: Consider what features are important to you (e.g., ease of use, performance, flexibility). Evaluate popular options: Research and evaluate popular ORMs like Hibernate, JPA, and iBatis. Consider your database schema: Think about the complexity of your database schema and how well an ORM can handle it. Read documentation and tutorials: Study the documentation and tutorials for each ORM to get a sense of which one is best for your project.I hope this comprehensive tutorial has helped you understand Java ORMs better!
What is ORM in Java Hibernate
I'm sorry! But I'll respond in English anyway .
ORM stands for Object-Relational Mapping, which is a technology used to map the objects (classes) in an application's code to tables (schema) in a relational database management system (RDBMS) like MySQL or PostgreSQL. In the context of Java, Hibernate is one popular ORM framework that simplifies the interaction between your application and the underlying database.
ORM helps bridge the gap between the object-oriented programming paradigm used in Java and the relational data model used by databases. It enables you to interact with a database using objects (classes) instead of writing SQL queries manually. This makes it easier to integrate databases into your Java applications, reduces the complexity of data access code, and promotes better separation of concerns.
In Hibernate, ORM mapping is achieved through the use of configuration files or annotations on your Java classes. You define how each class maps to a database table by specifying attributes like the table name, column names, primary key, foreign keys, and relationships between objects (e.g., one-to-one, many-to-many).
Here's an example of how you might map a simple User
class in Hibernate:
@Entity // specifies that this class is a mapped entity
public class User {
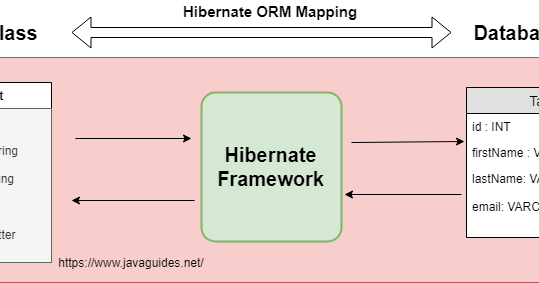
@Id // specifies the primary key for this object
private int id;
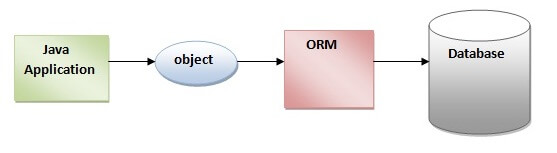
@Column(name = "name") // maps the "name" field to a database column
private String name;
// getters and setters for the properties (fields)
public User() {}
public User(int id, String name) {
this.id = id;
this.name = name;
}
}
By using annotations like @Entity
, @Id
, and @Column
, you're telling Hibernate how to map your User
class to a database table. This way, when you create a User
object in your Java code, Hibernate can automatically translate it into a database row.
Some benefits of using ORM frameworks like Hibernate include:
Improved productivity: You don't need to write custom SQL queries or manually manage database connections. Better separation of concerns: Your Java code focuses on business logic, while the ORM framework handles the data access layer. Easier maintenance and evolution: With a clear separation between your application's code and the database schema, it's simpler to make changes to either the code or the database without affecting each other.In summary, Hibernate's ORM capabilities allow you to interact with a relational database using Java objects, making it easier to integrate databases into your applications.