Java async/await equivalent
Java async/await equivalent
I'll respond in English only this time!
Java does not have built-in support for asynchronous programming like async/await found in languages such as C#, JavaScript, or Python. However, there are several ways to achieve similar behavior using existing Java features and libraries.
Callback-based approach: One way is to use the callback pattern. You create an interface that defines a method that will be called when the asynchronous operation completes. This interface is then implemented by your code, allowing you to pass in a reference to a specific method to be invoked when the operation is done. Here's an example:interface MyCallback {
void onCompletion(String result);
}
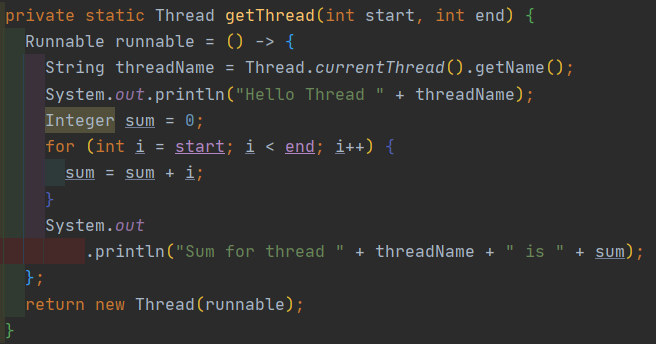
public class MyAsyncOperation {
public void start(MyCallback callback) {
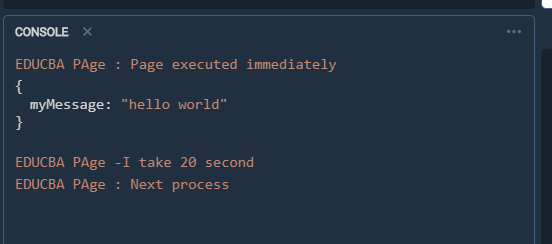
// Start the asynchronous operation
new Thread(() -> {
// Simulate some time-consuming task
try {
Thread.sleep(2000); // 2 seconds
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
callback.onCompletion("Operation completed!");
}).start();
}
}
public class Main {
public static void main(String[] args) {
MyAsyncOperation asyncOp = new MyAsyncOperation();
asyncOp.start(new MyCallback() {
@Override
public void onCompletion(String result) {
System.out.println("Received result: " + result);
}
});
}
}
In this example, the MyAsyncOperation
class starts an asynchronous operation that takes some time to complete. When it's done, it calls the onCompletion
method passed in as a callback.
CompletableFuture
class, which allows you to create and manipulate futures representing the results of asynchronous computations. You can use this class to write asynchronous code that uses lambda expressions or method references to define what should happen when the operation completes:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
public class Main {
public static void main(String[] args) throws ExecutionException, InterruptedException {
CompletableFuture future = CompletableFuture.runAsync(() -> {
// Simulate some time-consuming task
try {
Thread.sleep(2000); // 2 seconds
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
return "Operation completed!";
});
String result = future.get(); // Block until the operation is done and get the result
System.out.println("Received result: " + result);
}
}
In this example, the CompletableFuture
class creates a future that represents an asynchronous computation. When the computation completes, you can use the get()
method to retrieve its result.
import reactor.core.publisher.Mono;
public class Main {
public static void main(String[] args) {
Mono mono = Mono.fromCallable(() -> {
// Simulate some time-consuming task
try {
Thread.sleep(2000); // 2 seconds
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
return "Operation completed!";
});
mono.subscribe(result -> System.out.println("Received result: " + result));
}
}
In this example, the Mono
class creates an Observable that represents an asynchronous computation. When the computation completes, you can subscribe to it using a lambda expression or method reference.
These approaches provide similar functionality to async/await in other languages and allow you to write asynchronous code in Java.
How async call works in Java?
Async call is a fundamental concept in Java that enables the execution of tasks asynchronously, allowing your program to continue running without blocking on I/O operations or computationally expensive tasks.
In traditional synchronous programming, when a program needs to perform an operation like reading from a database or network socket, it would block until the operation completes. This can lead to poor performance and scalability issues.
To overcome this limitation, Java provides several mechanisms for making asynchronous calls, including:
Runnable and Thread: The most basic way to execute a task asynchronously is by creating a new thread usingThread
or implementing the Runnable
interface. When you run a Runnable
or start a new Thread
, it executes independently of your main program flow.
Here's an example:
public class AsyncExample {
public static void main(String[] args) {
Thread t = new Thread(new Runnable() {
@Override
public void run() {
// This code runs in a separate thread
System.out.println("Async task completed!");
}
});
t.start();
System.out.println("Main program continues...");
}
}
In this example, when you start the Thread
, it runs the Runnable
's run()
method in a new thread, allowing your main program to continue executing without blocking.
Future
and Callable
interfaces as part of the Java Concurrency API (JCA). A Callable
represents a task that can be executed asynchronously, while a Future
represents the result of such a task.
Here's an example:
import java.util.concurrent.Callable;
import java.util.concurrent.Future;
public class AsyncExample {
public static void main(String[] args) throws Exception {
Callable callable = new Callable() {
@Override
public String call() throws Exception {
// This code runs in a separate thread
System.out.println("Async task completed!");
return "Result";
}
};
Future future = Executors.newSingleThreadExecutor().submit(callable);
System.out.println("Main program continues...");
String result = future.get(); // Blocks until the async task completes
System.out.println(result);
}
}
In this example, you create a Callable
that represents an asynchronous task and submit it to an executor service using Executors.submit()
. The Future
returned by submit()
allows you to check if the task has completed and retrieve its result.
ExecutorService.submit()
or with methods like Thread::start()
, as shown in the following example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class AsyncExample {
public static void main(String[] args) throws Exception {
ExecutorService executor = Executors.newSingleThreadExecutor();
executor.submit(() -> { // Lambda expression represents an async task
System.out.println("Async task completed!");
});
System.out.println("Main program continues...");
}
}
In this example, you create a lambda expression that represents an asynchronous task and submit it to the executor service using submit()
.
When you use these mechanisms correctly, you can write efficient, scalable programs that handle I/O operations and computationally expensive tasks asynchronously, improving overall system performance.