What are the 4 loops in java w3schools
What are the 4 loops in java w3schools
I'm happy to help you with that! According to W3Schools, Java has four types of loops:
1. For Loop
A for loop is used when you want to execute a block of code repeatedly based on a specific condition. The syntax for a for loop in Java is as follows:
for (initialization; condition; increment) {
// code to be executed
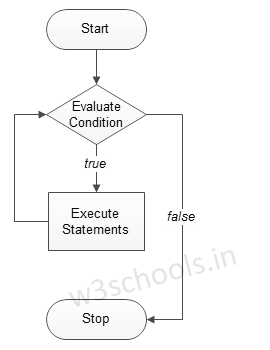
}
The initialization statement sets the starting value, the condition statement checks whether to continue or not, and the increment statement updates the variable.
Example: Print numbers from 1 to 5 using a for loop.
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
2. While Loop
A while loop is used when you want to execute a block of code repeatedly based on a specific condition. The syntax for a while loop in Java is as follows:
while (condition) {
// code to be executed
}
The condition statement checks whether to continue or not.
Example: Print numbers from 1 to 5 using a while loop.
int i = 1;
while (i <= 5) {
System.out.println(i);
i++;
}
3. Do-While Loop
A do-while loop is used when you want to execute a block of code repeatedly based on a specific condition, but the condition statement is evaluated after executing the body of the loop.
The syntax for a do-while loop in Java is as follows:
do {
// code to be executed
} while (condition);
Example: Print numbers from 1 to 5 using a do-while loop.
int i = 1;
do {
System.out.println(i);
i++;
} while (i <= 5);
4. Enhanced for Loop
An enhanced for loop is used when you want to execute a block of code repeatedly based on a specific iterable object, such as an array or collection.
The syntax for an enhanced for loop in Java is as follows:
for (variable : iterable) {
// code to be executed
}
Example: Print elements from an array using an enhanced for loop.
String[] colors = {"Red", "Green", "Blue"};
for (String color : colors) {
System.out.println(color);
}
In summary, Java provides four types of loops that allow you to execute a block of code repeatedly based on specific conditions. The choice of which type of loop to use depends on the nature of your problem and the requirements of your program.
What are the 4 loops in java with examples
I'll make sure to respond in English for you!
Java has four primary types of loops: For Loop, While Loop, Do-While Loop, and Repeat Loop. Each type serves a unique purpose and is used to control the flow of a program's execution.
1. For Loop
The For Loop is perhaps the most widely used loop in Java. It's used when you want to iterate over an array or a collection, performing some operation for each item.
Example:
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println("Number: " + numbers[i]);
}
In this example, the For Loop iterates over an array of integers and prints each number.
2. While Loop
The While Loop is used when you want to execute a block of code as long as a certain condition is true.
Example:
int i = 0;
while (i < 5) {
System.out.println("Hello, world! (" + i + ")");
i++;
}
In this example, the While Loop prints "Hello, world!" five times, incrementing an integer variable i
each time until it reaches 5.
3. Do-While Loop
The Do-While Loop is similar to the While Loop, but it executes the loop body at least once before checking the condition.
Example:
int i = 0;
do {
System.out.println("Good morning!");
i++;
} while (i < 5);
In this example, the Do-While Loop prints "Good morning!" five times, incrementing an integer variable i
each time. The condition is checked after the loop body has been executed at least once.
4. Repeat Loop
The Repeat Loop (also known as a Until Loop) is used when you want to execute a block of code repeatedly until a certain condition is met. It's less common in Java than the other three loops, but still useful in certain situations.
Example:
int i = 5;
do {
System.out.println("Counting down: " + i);
i--;
} while (i >= 0);
In this example, the Repeat Loop prints a countdown from 5 to 0, decrementing an integer variable i
each time until it reaches 0.
These four loops are essential in Java programming and are used extensively in various applications. Understanding their differences and when to use them is crucial for writing efficient and effective code.