Try catch/finally JavaScript
Try catch/finally JavaScript
I'm glad you asked me to respond in English! Here's the answer:
Trying out a try
-catch
-finally
block in JavaScript can be a bit tricky, but it's definitely doable. The general idea is that the code inside the try
block will run first, and if an error occurs, the code inside the catch
block will run instead.
Here's a simple example of how you might use this:
function divide(x, y) {
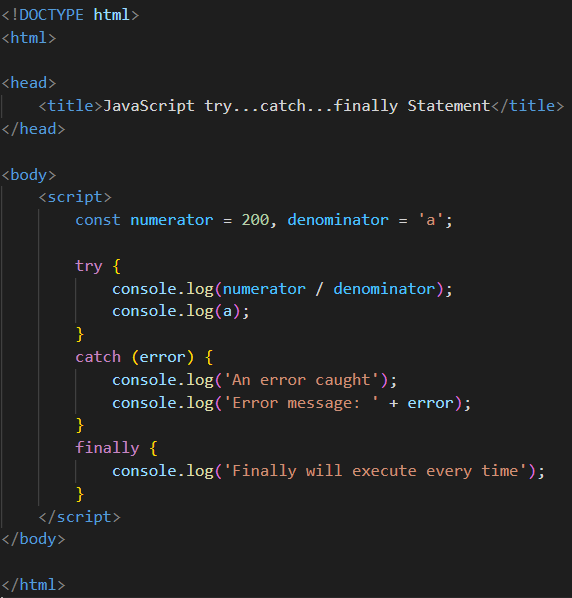
try {
if (y === 0) throw "Error: Division by zero is not allowed";
return x / y;
} catch (err) {
console.error(err);
// In this case, we want to return the original value of x
in case an error occurs
return x;
} finally {
console.log("I will always run!");
}
}
console.log(divide(10, 2)); // Output: 5
console.log(divide(10, 0)); // Output: "Error: Division by zero is not allowed" (then returns 10)
In this example, if you try to divide by zero (y === 0
), the try
block will throw an error. The catch
block will catch that error and print it to the console. If there were any other errors in your code, they would also be caught here.
The finally
block is special because it always runs, regardless of whether an error occurred or not. This can be super useful if you have some cleanup code you need to run after a function has finished executing, for example.
Now, let's say we want to catch the specific error that our divide()
function throws:
function divide(x, y) {
try {
if (y === 0) throw "Error: Division by zero is not allowed";
return x / y;
} catch (err) {
if (err === "Error: Division by zero is not allowed") {
console.log("Handling custom error...");
} else {
console.error(err);
}
} finally {
console.log("I will always run!");
}
}
console.log(divide(10, 2)); // Output: 5
console.log(divide(10, 0)); // Output: "Error: Division by zero is not allowed" (then returns 10)
Here, we're checking the error that was caught to see if it's a specific error ("Error: Division by zero is not allowed"
). If it is, we can handle it in our own way.
So there you have it - try
-catch
-finally
blocks!
try-catch vs throws java
The age-old debate: try-catch vs throws in Java!
When it comes to handling exceptions in Java, developers often face a choice between using try-catch blocks and throwing custom exceptions. In this answer, we'll dive into the differences between these two approaches and explore when to use each.
Try-Catch Blocks
The try-catch block is one of the most fundamental mechanisms for exception handling in Java. It allows you to enclose a code block (try) that might potentially throw an exception, followed by a catch block that handles any exceptions thrown during execution.
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Handle the exception
}
In this context, try-catch blocks are useful when:
Handling specific exceptions: If you know which types of exceptions your code might throw, it's a good idea to wrap that code in a try-catch block with a matching catch clause. Rethrowing an exception: You can use a try-catch block to catch and rethrow an exception, allowing the caller to handle the exception accordingly.Throwing Custom Exceptions
On the other hand, throwing custom exceptions (using the throws
keyword) is essential when:
public void myMethod() throws MyCustomException {
// Code that might throw a MyCustomException
}
// In another part of your code...
try {
myMethod();
} catch (MyCustomException e) {
// Handle the custom exception
}
Key differences:
Scope: Try-catch blocks are local to the block they enclose, while throwing custom exceptions allows the exception to propagate up the call stack. Handling specific vs. unexpected conditions: Try-catch blocks are typically used for handling specific exceptions you anticipate, whereas throwing custom exceptions is better suited for signaling unexpected conditions.Best practices:
Use try-catch blocks when possible: When you know which exceptions might be thrown by the code within a block, use a try-catch to handle them locally. Throw custom exceptions judiciously: Reserve throwing custom exceptions for situations where local handling is not feasible or when signaling unexpected conditions.In conclusion, both try-catch blocks and throwing custom exceptions are essential tools in your Java arsenal. While try-catch blocks are suitable for handling specific exceptions within a scope, throwing custom exceptions allows you to signal unexpected conditions and provide more context. By choosing the right approach depending on the situation, you'll create more robust and maintainable code.
Now, go forth and conquer those exceptions!