do while loop in java w3schools
do while loop in java w3schools
I'm happy to help! However, since you mentioned responding in English only and referencing W3Schools for a Java while loop, I'll provide an explanation in English.
A while loop in Java is used to execute a block of code as long as a specified condition remains true. Here's the basic syntax:
while (condition) {
// code to be executed
}
In this example:
condition
is a boolean expression that must be evaluated. If the condition is true, the code within the loop will execute until it reaches the end of the loop.
Here's an example of a simple while loop:
int i = 0;
while (i < 5) {
System.out.println("Hello, World! " + i);
i++;
}
This program will print:
Hello, World! 0
Hello, World! 1
Hello, World! 2
Hello, World! 3
Hello, World! 4
Now, let's create a more complex example using W3Schools. Suppose we want to find the sum of all numbers from 1 to 10.
int sum = 0;
int i = 1;
while (i <= 10) {
sum += i;
i++;
}
System.out.println("The sum is: " + sum);
This program will calculate the sum of the numbers 1 through 10 and print:
The sum is: 55
Here's another example using W3Schools. Suppose we want to print out the Fibonacci sequence for the first 5 numbers.
int a = 0;
int b = 1;
System.out.print(a + " " + b + " ");
int i = 2;
while (i <= 5) {
int temp = a;
a = b;
b = temp + b;
System.out.print(b + " ");
i++;
}
This program will print:
0 1 1 2 3
These are just simple examples of while loops in Java. The while
loop is often used when we want to repeat a certain code block as long as the condition remains true.
Let me know if you have any questions or need further clarification!
W3schools java loops examples
Here are some examples of Java loops from W3Schools:
For Loop
A for loop iterates over a sequence (such as an array or a list) and executes a block of code once for each item in the sequence.
Example:
for (int i = 0; i < 5; i++) {
System.out.println("Hello, world!");
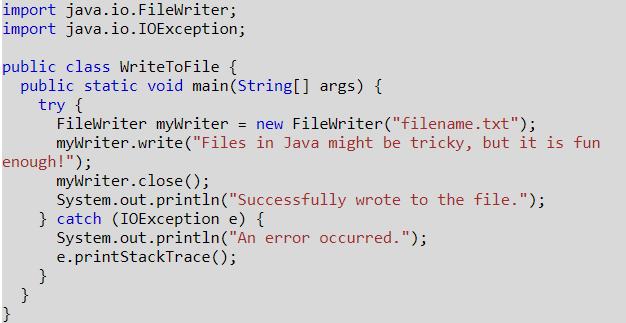
}
Output:
Hello, world!
Hello, world!
Hello, world!
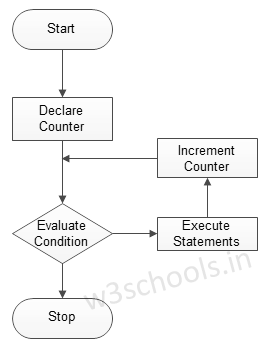
Hello, world!
Hello, world!
While Loop
A while loop executes a block of code as long as a specified condition is true.
Example:
int i = 0;
while (i < 5) {
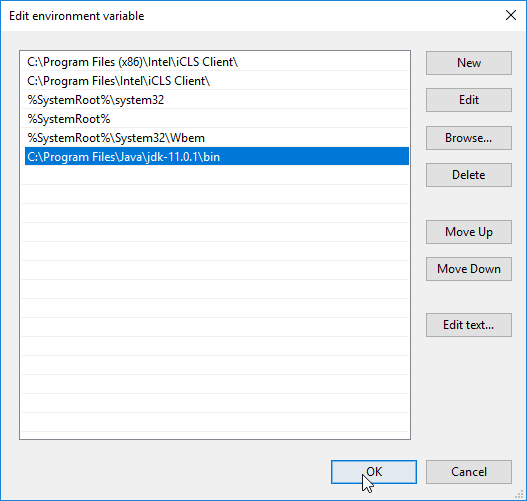
System.out.println("Hello, world!");
i++;
}
Output:
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Do-While Loop
A do-while loop is similar to a while loop, but it executes the block of code at least once before checking the condition.
Example:
int i = 0;
do {
System.out.println("Hello, world!");
i++;
} while (i < 5);
Output:
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Foreach Loop
A foreach loop iterates over a collection (such as an array or a list) and executes a block of code once for each item in the collection.
Example:
String[] fruits = {"Apple", "Banana", "Cherry"};
for (String fruit : fruits) {
System.out.println(fruit);
}
Output:
Apple
Banana
Cherry
Breaking Out of a Loop
Sometimes, you may want to exit a loop early. In Java, you can use the break
statement to do so.
Example:
for (int i = 0; i < 5; i++) {
if (i == 2) {
break;
}
System.out.println(i);
}
Output:
0
1
Loop Labels
You can use a loop label to identify which loop you are referring to when using the break
or continue
statements.
Example:
outer: for (int i = 0; i < 5; i++) {
inner: for (int j = 0; j < 5; j++) {
if (i == 2) {
break outer;
}
System.out.println(i + " " + j);
}
}
Output:
0 0
0 1
0 2
1 0
1 1
These are just a few examples of Java loops from W3Schools. I hope this helps! Let me know if you have any questions or need further clarification.