Non primitive data types in javascript examples
Non primitive data types in javascript examples
I'm happy to respond in English!
Non-primitive data types in JavaScript are objects and arrays. These data types allow you to store complex data structures that can be manipulated using various methods and properties.
Objects:
In JavaScript, an object is a collection of key-value pairs where the keys are strings or symbols and the values can be any type of data (primitive or non-primitive). Objects are defined using curly braces { } and are used to represent real-world objects or complex data structures.
Here's an example of creating an object:
const person = {
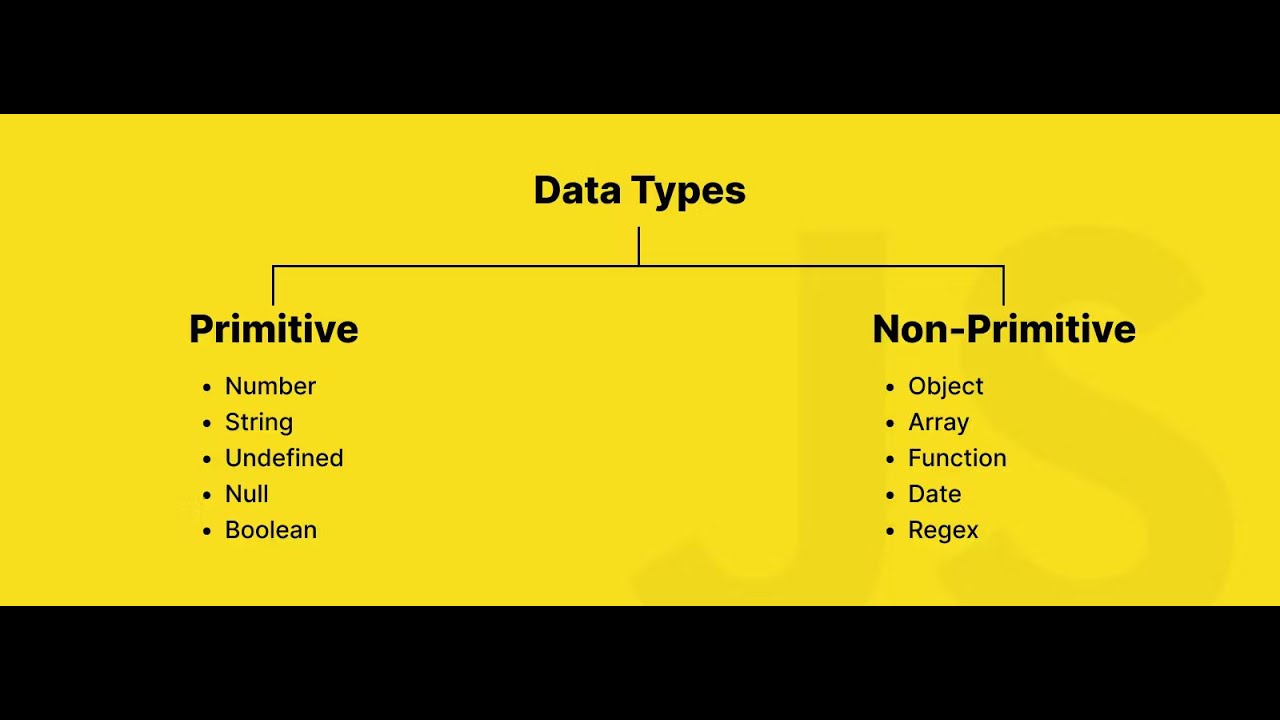
name: 'John',
age: 30,
address: {
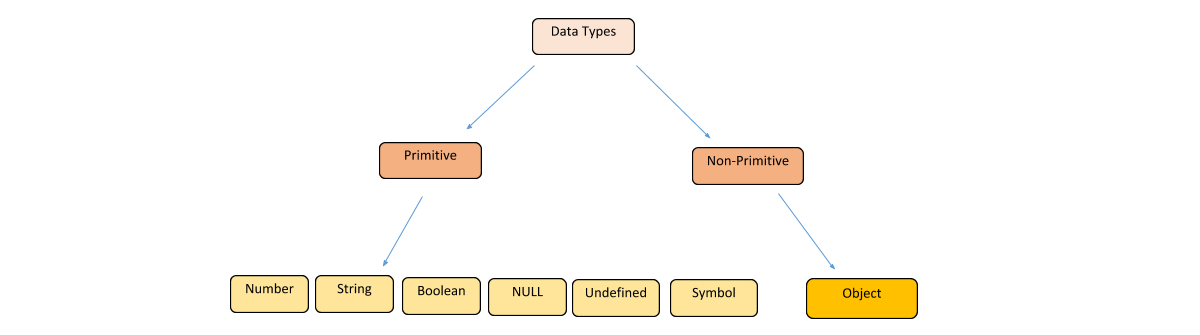
street: '123 Main St',
city: 'Anytown',
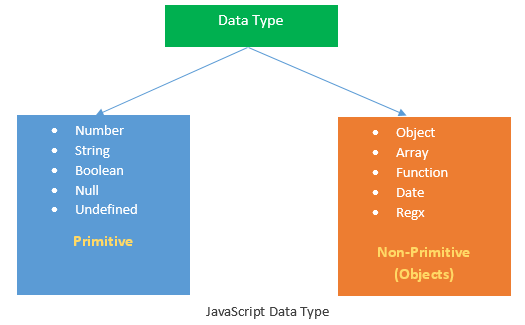
state: 'CA',
zip: '90210'
}
};
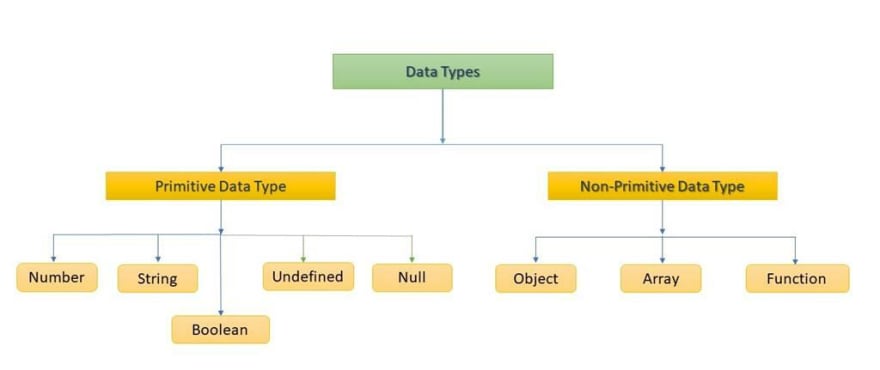
In this example, the person
object has three properties: name
, age
, and address
. The address
property is another object that contains four properties of its own (street
, city
, state
, and zip
).
Objects are mutable, meaning you can add or modify their properties at any time. Here's an example of adding a new property to the person
object:
person.email = '[email protected]';
Now, the person
object has a new email
property.
Arrays:
In JavaScript, an array is a collection of values that can be accessed using indices (0-based). Arrays are defined using square brackets [] and are used to represent lists of items or collections of data.
Here's an example of creating an array:
const colors = ['red', 'green', 'blue'];
In this example, the colors
array has three elements: 'red'
, 'green'
, and 'blue'
.
Arrays are also mutable, meaning you can add or remove their elements at any time. Here's an example of adding a new element to the colors
array:
colors.push('yellow');
Now, the colors
array has four elements: 'red'
, 'green'
, 'blue'
, and 'yellow'
.
Non-primitive data types can also be used as keys in objects or as values in other non-primitive data types. For example:
const person = {
john: { age: 30, occupation: 'developer' },
mary: { age: 25, occupation: 'designer' }
};
In this example, the person
object has two properties with string keys ('john'
and 'mary'
) that have objects as their values.
These are just a few examples of how non-primitive data types can be used in JavaScript. I hope this helps clarify things!
What is the difference between primitive and non primitive data types in JavaScript medium?
I'd be happy to explain the differences between primitive and non-primitive data types in JavaScript!
Primitive Data Types:
In JavaScript, primitive data types are those that have a fixed size and are stored exactly as they're represented. These include:
Numbers (number): This is a numeric value that can be an integer or a decimal.Example: let x = 42;
Example: let str = 'hello';
Example: let bool = true;
Example: let nullVar = null;
Example: let undefVar; // no initialization
Non-Primitive Data Types (Objects):
Non-primitive data types, on the other hand, are more complex and can contain multiple values or nested structures. These include:
Arrays: A collection of primitive or non-primitive values that can be accessed by index.Example: let arr = [1, 2, 3];
Example: let obj = {name: 'John', age: 30};
Here are some key differences between primitive and non-primitive data types:
Size: Primitive data types have a fixed size, while non-primitive data types can grow dynamically as they're modified. Structure: Primitive data types are simple values that can be used directly in expressions, while non-primitive data types are complex structures that require additional processing to access or modify their contents. Equality Comparison: Primitive data types compare equal using the==
operator (e.g., 42 == 42
is true), while non-primitive data types use a more nuanced comparison algorithm (e.g., two arrays with different lengths will be considered unequal, even if they contain the same values in the same order). Serialization: Primitive data types can often be serialized easily using stringification or JSON conversion, while non-primitive data types may require additional processing to serialize their complex structures. Memory Management: Non-primitive data types typically require more memory than primitive data types since they store references to separate objects rather than storing the values themselves.
In summary, primitive data types in JavaScript are simple values that have a fixed size and can be used directly in expressions. Non-primitive data types, on the other hand, are complex structures that contain multiple values or nested arrays, which require additional processing to access or modify their contents.
I hope this helps!