What is the long data type literal in java w3schools
What is the long data type literal in java w3schools
I'd be happy to help!
In Java, a long data type literal refers to the representation of a long integer value as a string literal within the code. A long integer is an 8-byte signed integer that can hold values from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Long data type literals are used extensively in Java programming when working with large integers. For instance, you might use them to represent dates, timestamps, or IDs that exceed the range of an integer but fit within a long integer's range.
According to W3Schools (a popular online platform for web developers), a long data type literal is denoted by the "L" suffix at the end of the number. This suffix indicates that the value being assigned is of the long data type.
Here are some examples of long data type literals in Java:
long x = 1000000000L; // Assigning a value to a long variable
System.out.println(x); // Outputs: 1000000000
long y = Long.MAX_VALUE; // Getting the maximum value of a long
System.out.println(y); // Outputs: 9223372036854775807
In these examples, the values are assigned to long
variables using the "L" suffix. This suffix is not required for literals within mathematical expressions or as part of complex statements.
It's essential to understand the use and representation of long data type literals in Java programming, especially when dealing with large integers that exceed the range of an integer.
W3Schools provides a comprehensive overview of Java programming concepts, including data types, control structures, functions, and more. Their website is a valuable resource for web developers, offering tutorials, reference materials, and online courses to help users learn and master various programming languages, including Java.
Sources:
W3Schools: Java Tutorial Oracle: Java SE 8 Documentation - Language FundamentalsI hope this information helps you better understand long data type literals in Java!
Java long example
I'd be happy to provide an example of a Java program that demonstrates the use of the long
data type.
Here is a simple example of a Java program that calculates the area and perimeter of a rectangle:
public class Rectangle {
public static void main(String[] args) {
long length = 1000000000L; // declare a long variable with a value in excess of Integer.MAX_VALUE
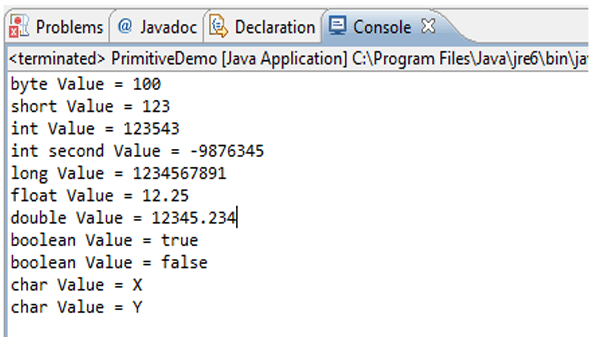
long width = 500000000L;
double area = (double)length * width;
long perimeter = (long)((2 * length) + (2 * width));
System.out.println("Length: " + length);
System.out.println("Width: " + width);
System.out.println("Area: " + area);
System.out.println("Perimeter: " + perimeter);
}
}
In this example, we declare two long
variables, length
and width
, to represent the dimensions of a rectangle. We then calculate the area (area
) by multiplying these values together, and the perimeter (perimeter
) by adding up the lengths of all four sides.
When you run this program, you'll see that it uses the long
data type to store large integers that are larger than what can fit in an int
. This is important for applications where we need to handle very large numbers, such as financial transactions or scientific calculations.
Here's why we need long
instead of int
: the maximum value an int
can hold is 2^31-1
, which is approximately 2 billion. If our rectangle's length or width were larger than this, we would lose precision and get incorrect results.
On the other hand, the maximum value a long
can hold is 2^63-1
, which is approximately 9 quintillion. This allows us to accurately represent very large numbers that exceed the range of an int
.
I hope this example helps illustrate how Java's long
data type can be used in practical programming scenarios!