Java AtomicReference
Java AtomicReference
I'm happy to help!
Java AtomicReference is a class within the java.util.concurrent.atomic package that allows you to atomically update a reference type variable (like a String or an Object) in a multi-threaded environment. It's similar to the atomic integer operations provided by AtomicInteger, but for references.
Here are some key features and usage scenarios:
Atomic Updates: You can use AtomicReference to atomically update a reference type variable, such as swapping two references, updating a reference with a new value, or incrementing a counter.Example:
AtomicReference name = new AtomicReference<>("John");
name.compareAndSet("John", "Jane"); // atomic swap
Compare-And-Swap (CAS): The AtomicReference class provides the compareAndSet() method for CAS operations. This method checks whether the current value of the reference matches the expected value, and if so, updates it with the new value.
Example:
AtomicReference counter = new AtomicReference<>(0);
counter.compareAndSet(0, 1); // increment counter atomically
Load-Link/Store-Conditional (LL/SC): This mechanism allows you to load a reference, check whether it has changed since the last load, and if so, store the new value.
Example:
AtomicReference name = new AtomicReference<>("John");
String loadedName = name.get(); // load the current name
// ... some operations ...
if (!name.compareAndSet(loadedName, "Jane")) { // check for updates
System.out.println("Name changed!");
}
Supports volatile semantics: When updating a reference type variable using AtomicReference, you can ensure that changes are visible to other threads without the need for explicit synchronization (like locks or barriers). Thread-safety: AtomicReference is designed to work correctly in multi-threaded environments, even when multiple threads access and update the same reference type variable concurrently.
Best Practices:
Use AtomicReference only when you need atomic updates or CAS operations. When using compareAndSet() or other atomic operations, ensure that the expected value matches the current value to avoid unnecessary updates. Use volatile semantics wisely; it's not always necessary. Always consider the performance implications of using AtomicReference in your specific use case.Common Pitfalls:
Overusing AtomicReference for simple updates (e.g., setting a single variable). Failing to use compareAndSet() correctly, leading to unnecessary updates or false negatives. Ignoring performance considerations when choosing between AtomicReference and other concurrency tools.In summary, Java's AtomicReference provides a way to perform atomic updates and CAS operations on reference type variables in multi-threaded environments. By understanding its capabilities, limitations, and best practices, you can effectively use it to achieve thread-safety and correctness in your concurrent applications.
Java atomic operations tutorial
Here's a comprehensive tutorial on Java atomic operations:
Introduction
In multithreaded programming, it is crucial to ensure that multiple threads can access and update shared data safely and efficiently. One way to achieve this is by using atomic operations, which are operations that execute in a single step without interrupting other threads.
Java provides several classes for performing atomic operations: AtomicInteger
, AtomicLong
, AtomicReference
, and others. These classes provide methods for incrementing, decrementing, comparing, setting, and getting the value of an atomically accessed variable.
Atomic Operations
The following are some common atomic operations provided by Java:
Increment: Increments the current value by a specified amount.Here is an example of using AtomicInteger
for incrementing a counter:
import java.util.concurrent.atomic.AtomicInteger;
public class Counter {
private final AtomicInteger count = new AtomicInteger(0);
public int getCount() {
return count.get();
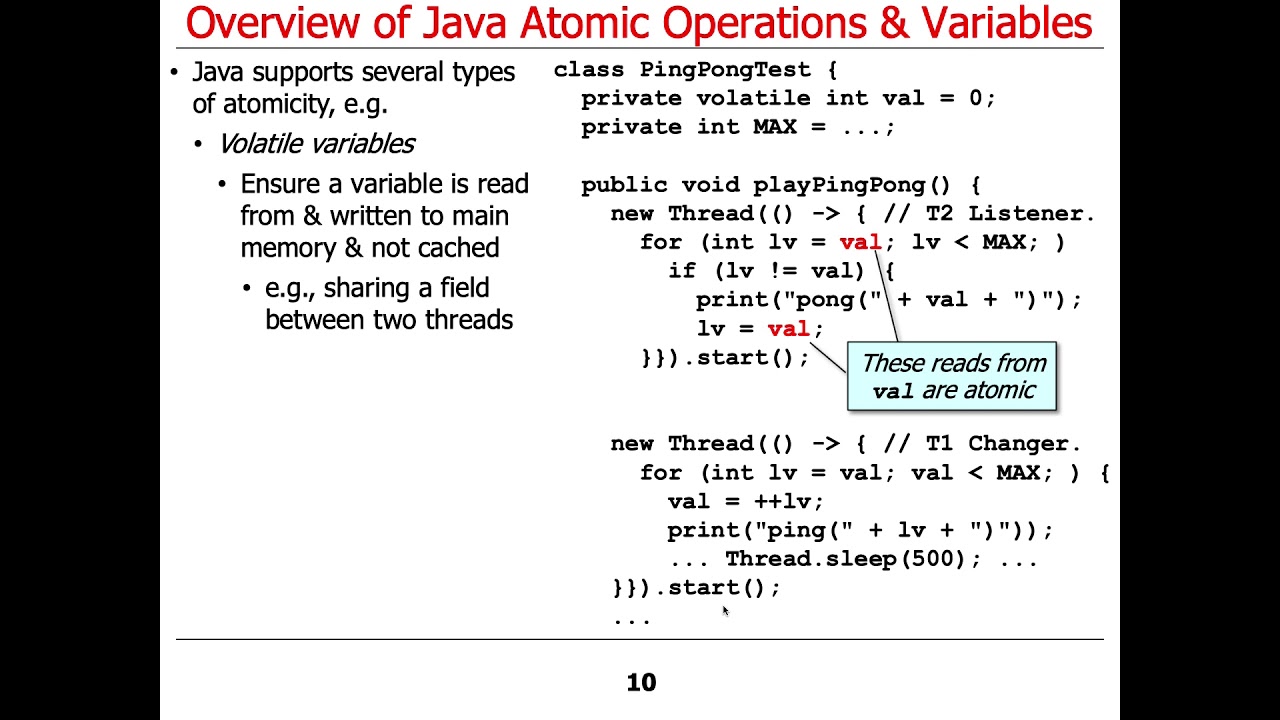
}
public void incrementCount() {
count.incrementAndGet();
}
}
Lock-Free Atomic Operations
In some cases, it's necessary to perform more complex atomic operations, such as compareAndSwap
or loadCompareStore
, which are lock-free and do not rely on synchronization primitives like locks. These operations provide better performance but can be more difficult to reason about.
Here is an example of using AtomicReference
for a compare-and-set operation:
import java.util.concurrent.atomic.AtomicReference;
public class Flag {
private final AtomicReference flag = new AtomicReference<>(false);
public boolean getFlag() {
return flag.get();
}
public void setFlag(boolean value) {
while (!flag.compareAndSet(false, value)) {
// do nothing
}
}
}
Using Atomic Operations
Here are some best practices for using atomic operations in Java:
Use the right type: UseAtomicInteger
for integer values and AtomicReference
for reference types. Use the correct operation: Choose the appropriate method based on your specific needs (e.g., increment, decrement, get-and-set, compare-and-set). Avoid unnecessary operations: Minimize the number of atomic operations performed to improve performance. Understand the semantics: Carefully consider the behavior and potential side effects of each operation.
Conclusion
In conclusion, Java's atomic operations provide a way to safely update shared data in multithreaded programs. By choosing the right type and operation, you can ensure that your code is efficient, scalable, and thread-safe. Always consider the semantics and potential side effects of each operation to avoid unexpected behavior.