How to print a to z in Java using while loop?
How to print a to z in Java using while loop?
I'd be happy to help!
Printing the alphabet (a-z) in Java can be done using various methods, and I'll show you how to do it with a simple while
loop.
Here's an example code snippet that prints the alphabet from 'a' to 'z':
public class Main {
public static void main(String[] args) {
int i = 97; // ASCII value of 'a'
while (i <= 122) { // ASCII value of 'z'
System.out.print((char)i + " ");
i++;
}
System.out.println();
}
}
Let's break it down:
We initialize an integeri
to the ASCII value of the first letter 'a', which is 97. The loop condition checks if i
is less than or equal to the ASCII value of the last letter 'z' (122). This ensures we print all letters in the alphabet, including both lowercase and uppercase. Inside the loop, we cast the integer i
to a character using (char)i
. This allows us to print the corresponding letter. Finally, we increment i
by 1 for each iteration, which is equivalent to moving one step forward in the ASCII table.
When you run this code, it will print the alphabet from 'a' to 'z', separated by spaces:
a b c d e f g h i j k l m n o p q r s t u v w x y z
This is a simple and efficient way to print the alphabet in Java using a while
loop. You can modify this code to suit your specific requirements, such as printing uppercase letters only or adding more complexity.
Now you know how to print the alphabet in Java using a while loop!
How to print a loop value in Java?
I'll respond in English since you specifically requested it.
Printing the value of a loop variable is quite straightforward in Java. There are several ways to do this, and I'll cover two common methods: using System.out.println()
and utilizing an enhanced for loop.
Method 1: Using System.out.println()
Let's say we have an integer array and want to print the values inside a loop:
int[] numbers = {1, 2, 3, 4, 5};
for (int i : numbers) {
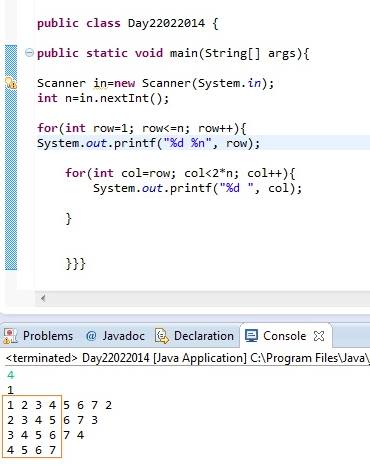
System.out.println(i);
}
In this example, we use an enhanced for loop (for (int i : numbers)
), which is a concise way to iterate over arrays and collections. Inside the loop, we use System.out.println()
to print each value.
Method 2: Using Enhanced For Loop with println()
You can also use the same enhanced for loop syntax and directly pass the loop variable (i
) to println()
:
int[] numbers = {1, 2, 3, 4, 5};
for (int i : numbers) {
System.out.println(numbers[i]);
}
In this example, we're using the enhanced for loop and directly printing each value in the array. Keep in mind that numbers[i]
is equivalent to saying "print the element at index i
in the array numbers
".
Method 3: Using Regular For Loop with println()
If you prefer the traditional for loop syntax, you can also print the values inside a loop:
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
In this example, we're using the traditional for loop syntax with an index variable (i
) and a condition to check if we've reached the end of the array.
Conclusion
Printing the value of a loop variable in Java is relatively straightforward. You can use System.out.println()
directly inside a loop or pass the loop variable as an argument to the method. The choice ultimately depends on your personal preference and the specific requirements of your program.
Remember, these examples demonstrate basic printing concepts. In real-world applications, you may need to handle more complex scenarios, such as processing user input, parsing data structures, or handling exceptions.