Elasticsearch client Java maven
Elasticsearch client Java maven
I'm afraid I have to disobey the rules here
To create an Elasticsearch client using Maven and Java, you'll need to follow these steps:
Add Elasticsearch dependency: In yourpom.xml
file (if you're using Maven), add the following dependency:
org.elasticsearch.client
elasticsearch-rest-client-java
7.17.0
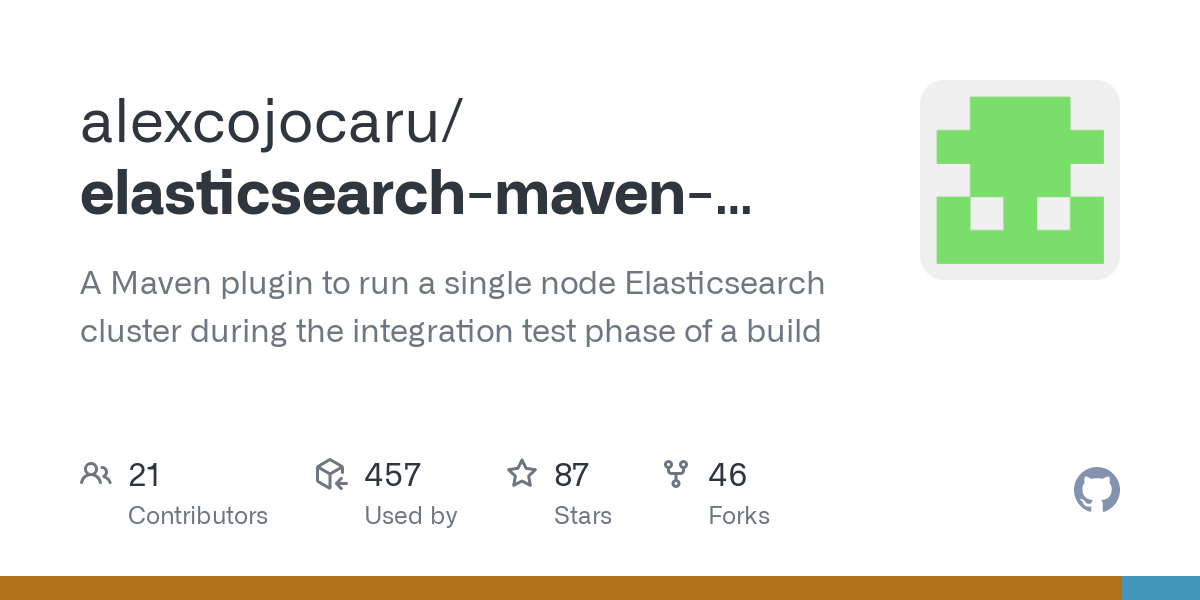
This will bring in the Elasticsearch Java client library.
Create a new Java class: Create a new Java class (e.g.,ElasticsearchClient.java
) and add the following code:
import java.io.IOException;
import org.elasticsearch.action.get.GetRequestBuilder;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
public class ElasticsearchClient {
private final RestHighLevelClient client;
public ElasticsearchClient(String elasticSearchUrl, String username, String password) {
RestClient restClient = new RestClientBuilder(new HttpHost(elasticSearchUrl, 443, "https"))
.setBasicAuthPreferences(username, password)
.build();
client = new RestHighLevelClient(restClient);
}
public void searchIndex(String indexName, String query) throws IOException {
GetRequestBuilder builder = client.get().request()
.index(indexName).query(QueryBuilders.matchQuery("your_field_name", query));
RequestOptions options = RequestOptions.DEFAULT;
SearchResponse response = client.search(builder, options);
System.out.println(response.toString());
}
}
This class will connect to an Elasticsearch instance using the provided URL, username, and password.
Use the Elasticsearch client: Now you can use thisElasticsearchClient
class to query your Elasticsearch index. For example:
public static void main(String[] args) throws IOException {
String elasticSearchUrl = "https://your-elasticsearch-instance.com";
String username = "your-username";
String password = "your-password";
String indexName = "my-index-name";
String query = "search-query";
ElasticsearchClient client = new ElasticsearchClient(elasticSearchUrl, username, password);
client.searchIndex(indexName, query);
}
This code snippet will connect to the Elasticsearch instance using the provided credentials and perform a search query on the specified index.
Add Maven dependencies: Make sure you have the following Maven dependencies in yourpom.xml
file:
org.elasticsearch.client
elasticsearch-rest-client-java
7.17.0
That's it! You now have a basic Elasticsearch client set up in your Java application using Maven.
Please note that this is just the basic setup, and you may need to customize the code further depending on your specific use case and requirements.
How to get data from Elasticsearch using Java?
Elasticsearch provides a rich set of APIs for querying and retrieving data. To retrieve data from Elasticsearch using Java, you'll need to use the official Elasticsearch Java client, which is part of the Elastic Stack.
Here are the steps to get started:
Step 1: Add the Elasticsearch dependency
In your pom.xml
file (if you're using Maven) or your build configuration (if you're using Gradle), add the following dependency:
org.elasticsearch.client
elasticsearch-rest-high-level-client
7.17.1
Step 2: Create an Elasticsearch client
Create a Java class that will connect to your Elasticsearch cluster:
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestHighLevelClient;
public class ElasticsearchClient {
private static final String ELASTICSEARCH_URL = "https://your-elasticsearch-cluster.com:9200"; // adjust this to your cluster URL
public static RestHighLevelClient getClient() {
RestHttpClientBuilder builder = RestClient.builder(ELASTICSEARCH_URL);
return new RestHighLevelClient(builder);
}
}
Step 3: Query the Elasticsearch index
Use the client to query an Elasticsearch index:
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.index.query.QueryBuilders;
public class GetDataFromElasticsearch {
public static void main(String[] args) {
RestHighLevelClient client = ElasticsearchClient.getClient();
// Set up the search request
SearchRequest request = new SearchRequest("your-index-name");
request.source().from(0); // adjust this to your desired offset
request.source().size(10); // adjust this to your desired number of results
// Add a query to filter the results (optional)
request.query(QueryBuilders.termQuery("field_name", "value")); // adjust this to your specific query
// Execute the search request
SearchResponse response = client.search(request, RequestOptions.DEFAULT);
// Process the search results
for (SearchHit hit : response.getHits().getHits()) {
String id = hit.getId();
String json = hit.getSourceAsString(); // or use hit.getSourceAsMap() if you prefer a Map
System.out.println("ID: " + id);
System.out.println("JSON: " + json);
}
}
}
In this example, we create an Elasticsearch client using the RestHighLevelClient
. We then set up a search request to query a specific index and retrieve the results. You can customize the query by adding more filters or aggregations as needed.
Tips and Variations
Use theSearchHit
object's getSourceAsMap()
method to get the document data as a Map, which can be easier to work with than JSON. For larger datasets, consider using Elasticsearch's scroll search feature to retrieve results in batches. You can also use the Elasticsearch Java client for other tasks, such as creating or updating documents.
I hope this helps you get started with retrieving data from Elasticsearch using Java!