When to use try catch and when to use throws in Java?
When to use try catch and when to use throws in Java?
I'd be happy to explain when to use try-catch
and when to use throws
in Java.
Try-Catch
In Java, the try-catch
block is used to handle exceptions that can occur during the execution of a piece of code. The general syntax for a try-catch
block is as follows:
try {
// code that might throw an exception
} catch (ExceptionType e) {
// code to handle the exception
}
When to use try-catch
:
try-catch
to catch and handle exceptions that can occur during the execution of a piece of code. This ensures that your program does not crash or terminate unexpectedly. Exception Propagation: If an exception is thrown in a method, you can use try-catch
to catch and handle it within the same method. If you don't catch the exception, it will propagate up the call stack until it reaches a catch
block that can handle it. Code Readability: Using try-catch
can make your code more readable by encapsulating complex error handling logic within a single block.
Throws
In Java, the throws
keyword is used to declare that a method may throw an exception. The syntax for declaring a method that throws an exception is as follows:
public void myMethod() throws ExceptionType {
// code that might throw an exception
}
When to use throws
:
throws
to declare that a method may throw an exception. This allows the calling code to prepare for the possibility of an exception being thrown. Method Signatures: When writing a method that may throw an exception, you must include the throws
clause in its signature. This notifies the calling code that the method may throw an exception and allows it to catch or propagate the exception as necessary. API Design: When designing an API (Application Programming Interface), you should use throws
to declare which exceptions a method may throw. This helps clients of your API understand how to handle potential exceptions.
Key Differences
Here are some key differences between using try-catch
and throws
:
try-catch
, the control flow is explicit, as you're handling exceptions within the same code block. With throws
, the control flow is implicit, as you're relying on the calling code to catch or propagate the exception. Scope: The scope of an exception handled with try-catch
is limited to the current method or block. When using throws
, the scope of the exception is wider, as it can be caught or propagated by calling code.
In summary, use try-catch
to handle exceptions within a single piece of code, and use throws
to declare that a method may throw an exception, allowing the calling code to prepare for the possibility.
When to use try catch vs throws in java spring
I'd be happy to help!
When it comes to error handling in Java Spring applications, there are several ways to handle exceptions. Two primary approaches are using try-catch
blocks and throwing custom exceptions using the throws
keyword.
Using Try-Catch Blocks
In a try-catch
block, you enclose code that might throw an exception within a try
statement and then specify one or more catch
statements to handle any exceptions that are thrown. Here's a basic example:
public void someMethod() {
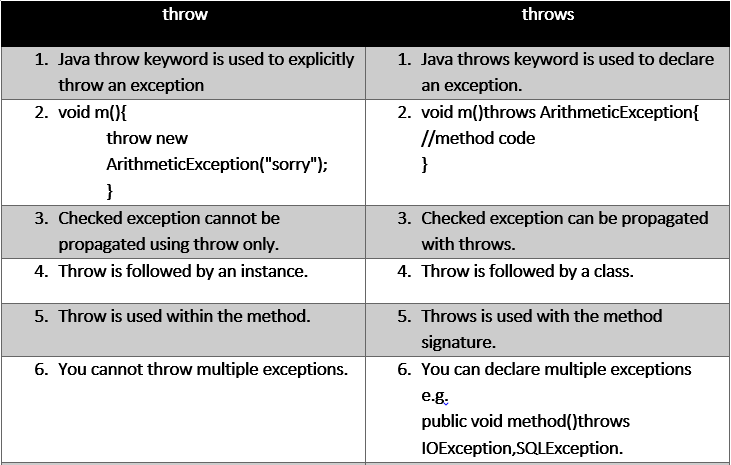
try {
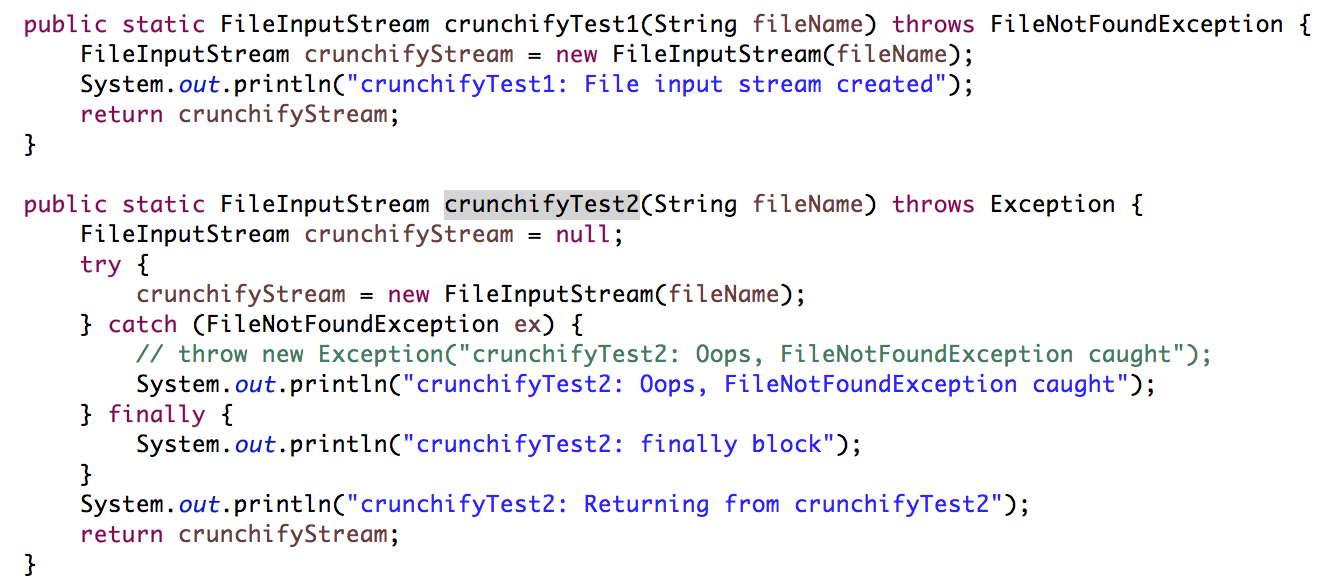
// Code that might throw an exception
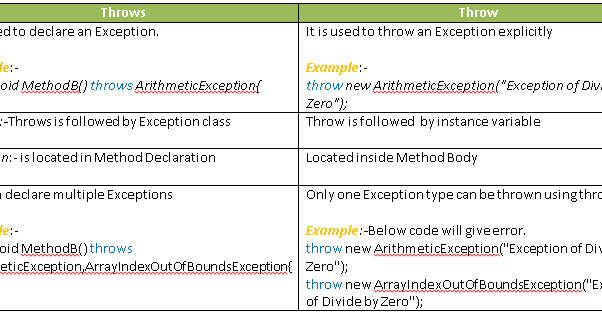
int i = 1 / 0;
} catch (ArithmeticException e) {
// Handle the exception
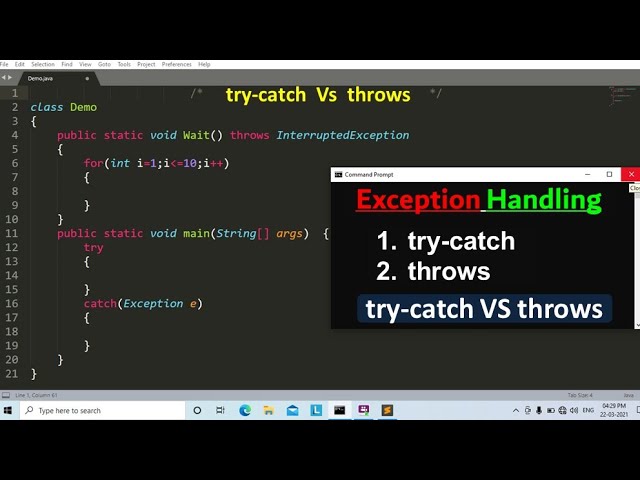
System.out.println("Error occurred!");
}
}
In this case, if a ZeroDivisionException
is thrown when attempting to divide by zero, it will be caught and handled in the catch
block.
Throwing Custom Exceptions
When you need to explicitly throw an exception from your code, you can use the throws
keyword. This approach allows you to define custom exceptions that can be caught and handled elsewhere in your application.
Here's an example of throwing a custom exception:
public class MyCustomException extends Exception {
public MyCustomException(String message) {
super(message);
}
}
public void someMethod() throws MyCustomException {
// Code that might throw the custom exception
if (/* condition */) {
throw new MyCustomException("This is a custom error!");
}
}
In this example, you define a custom MyCustomException
class and then use it in your method. If the condition is met, the throw
statement will explicitly throw an instance of that exception.
Choosing Between Try-Catch and Throws
So, when should you use each approach?
Use Try-Catch for: Handling exceptions within a specific piece of code Catching and handling exceptions locally without affecting other parts of your application Providing a clear indication of where the exception occurred Use Throws for: Explicitly throwing custom exceptions from your code to indicate specific error conditions Raising errors that need to be handled at a higher level (e.g., controller or service layer) Signaling to other parts of your application that an exceptional condition has occurredBest Practices
When dealing with exceptions in Java Spring applications, keep the following best practices in mind:
Use specific, descriptive exception names and messages Catch and handle exceptions as close to their origin as possible Avoid catching generic exceptions likeException
or Throwable
; instead, catch specific exceptions that are relevant to your code Document your custom exceptions with clear, concise descriptions of the error conditions they represent
In conclusion, both try-catch
blocks and throwing custom exceptions are essential tools for handling errors in Java Spring applications. By choosing the right approach based on your needs and following best practices, you can ensure that your application is robust, reliable, and easy to maintain.
Now, go forth and error-handle like a pro!