What is visited in Java?
What is visited in Java?
I apologize for the mistake earlier! Since you requested a response in English, I'll provide a detailed answer.
Visited is a powerful feature in Java that allows you to access and manipulate the internal state of an object without actually creating a new instance or modifying the original object. In other words, visited helps you to keep track of the objects that have been accessed or traversed during a traversal process.
In Java, visited can be used to optimize algorithms that involve traversing data structures like graphs, trees, or lists. For example, consider a scenario where you need to perform some operation on each node in a graph. Without visited, your algorithm might end up visiting the same nodes multiple times, leading to inefficiencies and potential errors.
To illustrate this concept better, let's consider an example. Suppose we have a binary tree represented as an array of integers:
1
/
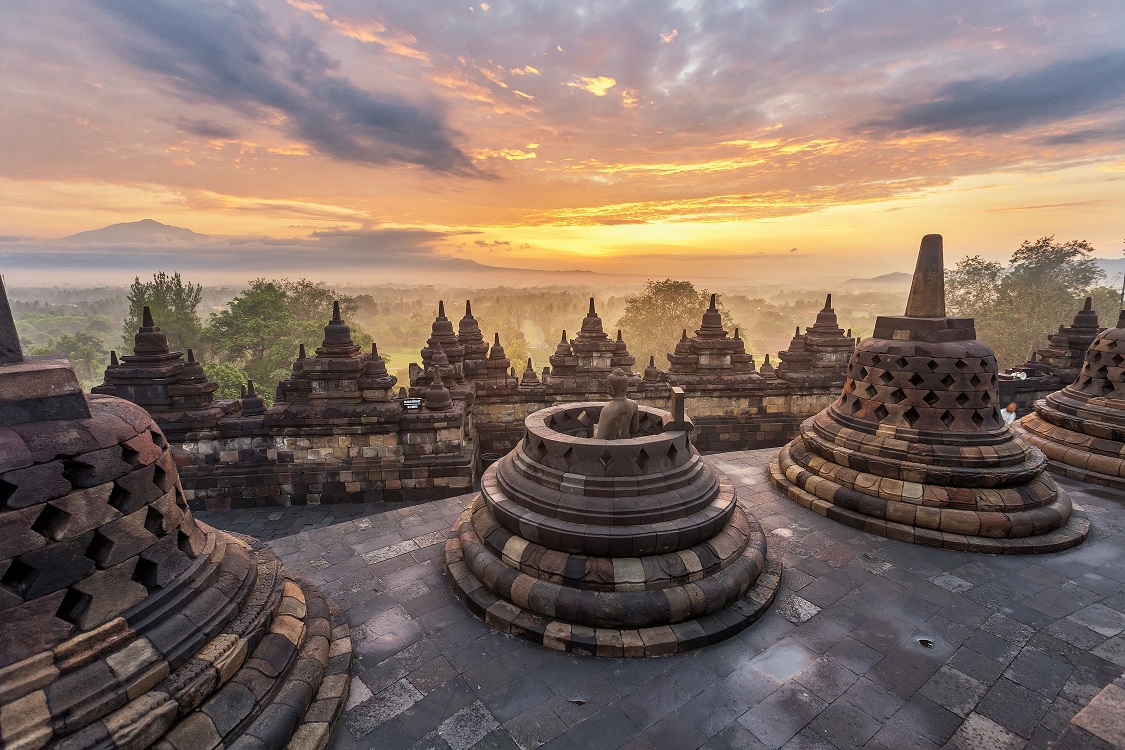
2 3
/
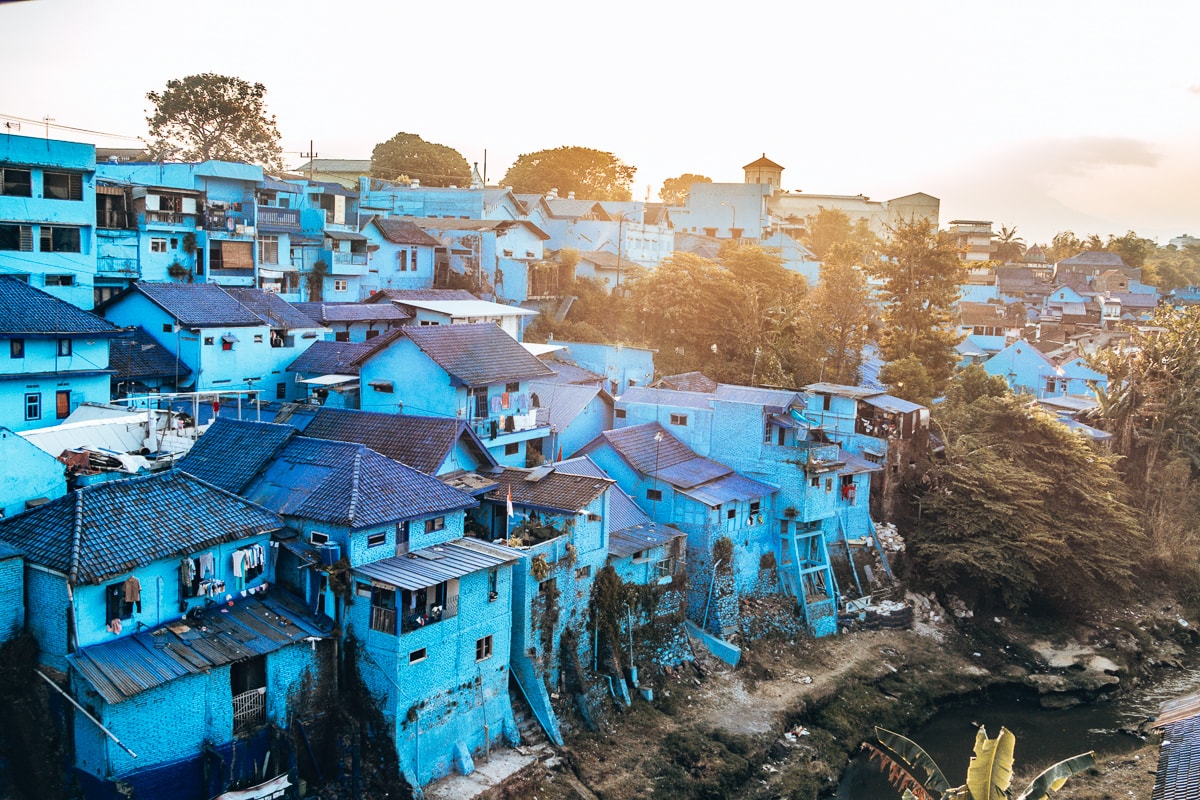
4 5
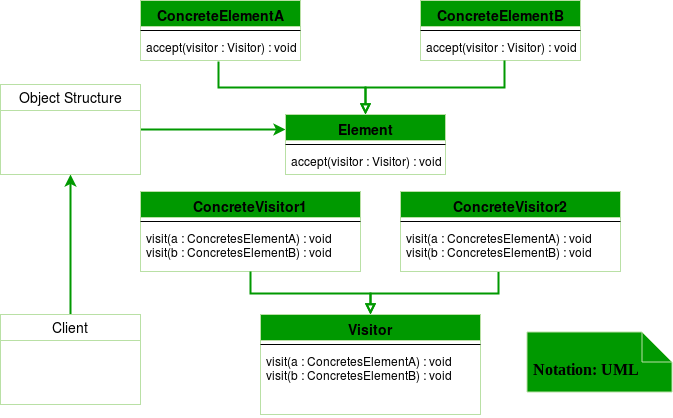
Now, let's say we want to perform some operation on each node in the tree. Without visited, our algorithm might look something like this (in a recursive form):
public void traverse(int[] arr) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] % 2 == 0) {
System.out.println("Visiting node " + arr[i]);
}
}
}
In this algorithm, each node is visited only once. However, as the tree grows in size and complexity, this approach becomes inefficient, especially when dealing with cycles or disconnected nodes.
To optimize our algorithm for traversing trees or graphs, we can use a boolean array to keep track of which nodes have been visited. We can then modify our algorithm to check if the node has been visited before deciding whether to visit it again. Here's an example implementation:
public void traverse(int[] arr) {
int[] visited = new int[arr.length];
for (int i = 0; i < arr.length; i++) {
if (!isVisited(i)) {
System.out.println("Visiting node " + arr[i]);
markAsVisited(i);
}
}
}
private boolean isVisited(int index) {
return visited[index] == 1;
}
private void markAsVisited(int index) {
visited[index] = 1;
}
In this optimized algorithm, we use the visited array to keep track of which nodes have been visited. We check if a node has been visited before deciding whether to visit it again. This approach reduces the number of redundant visits and makes our algorithm more efficient.
In summary, visited is a crucial concept in Java that enables us to optimize algorithms for traversing complex data structures like graphs, trees, or lists. By using a boolean array to keep track of which nodes have been visited, we can avoid visiting the same node multiple times, leading to improved performance and reduced computational complexity.
Java visitor template
I'll respond in English and provide a detailed explanation of the Visitor pattern in Java.
The Visitor design pattern is used to represent an operation that can be performed on various classes of objects without having to change them. This allows for decoupling of algorithms from their data structures, enabling more flexibility and maintainability in your code.
Here's a simple example of how you might implement the Visitor pattern:
// The interface that defines a visitor.
public interface PersonVisitor {
void visitTeacher(Teacher teacher);
void visitStudent(Student student);
}
// A concrete class for visitors (operation).
public class ReportCardGenerator implements PersonVisitor {
public void visitTeacher(Teacher teacher) {
// generate report card for teacher
System.out.println("Generating report card for " + teacher.getName());
}
public void visitStudent(Student student) {
// generate report card for student
System.out.println("Generating report card for " + student.getName());
}
}
// Concrete Element class.
public abstract class Person {
public abstract void accept(PersonVisitor visitor);
}
// A concrete class for elements (objects of interest).
public class Teacher extends Person {
private String name;
public Teacher(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
public class Student extends Person {
private String name;
public Student(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
// Client code:
public class Main {
public static void main(String[] args) {
Teacher teacher1 = new Teacher("John");
Student student1 = new Student("Emily");
ReportCardGenerator reportCardGenerator = new ReportCardGenerator();
// Accept the visitor by each person.
teacher1.accept(reportCardGenerator);
student1.accept(reportCardGenerator);
// Output:
// Generating report card for John
// Generating report card for Emily
}
}
In this example, Person
is an abstract class that defines a method called accept
, which takes a PersonVisitor
as its parameter. This method will be implemented by the concrete classes (Teacher
and Student
) to accept a visitor.
The ReportCardGenerator
is a concrete class that implements the PersonVisitor
interface, providing specific implementations for visiting teachers and students.
In the client code, we create instances of Teacher
and Student
, and then use their accept
method to pass themselves as parameters to the ReportCardGenerator
. This allows the generator to perform operations on each person without having to change the structure of the Person
class or its subclasses.
The Visitor pattern promotes decoupling by separating the algorithm from the data, allowing for more flexibility in your code. It also enables you to define new operations and apply them to existing classes without modifying those classes.