How to include JSON in Java?
How to include JSON in Java?
I'm happy to help!
Including JSON (JavaScript Object Notation) in a Java application can be achieved using various libraries and tools. Here are some common approaches:
JSON-simple: This is a lightweight library that provides basic JSON support, including serialization and deserialization of JSON data. You can add the following dependency to your Maven or Gradle project:
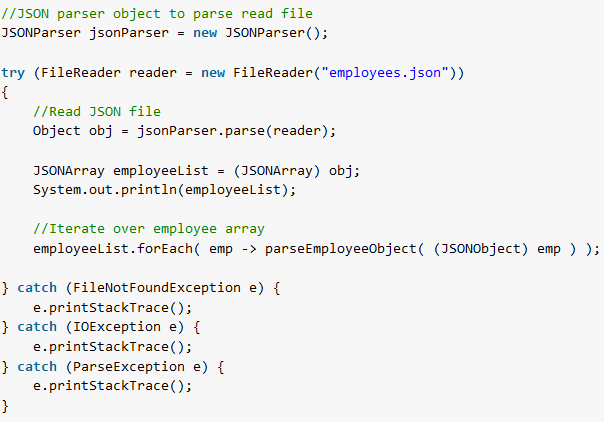
com.google.code.gson
gson
2.8.6
In your Java code, you can use the JSON
class to convert a Java object to JSON:
import com.google.gson.Gson;
import com.google.gson.JsonObject;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
JsonObject jsonObject = new JsonObject();
jsonObject.addProperty("name", "John");
jsonObject.addProperty("age", 30);
System.out.println(jsonObject.toString());
}
}
Jackson: This is a more powerful library that provides advanced JSON processing capabilities, including support for complex data structures and schema validation. You can add the following dependency to your Maven or Gradle project:
com.fasterxml.jackson.core
jackson-databind
2.12.3
In your Java code, you can use the ObjectMapper
class to convert a Java object to JSON:
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
MyJavaObject javaObject = new MyJavaObject("John", 30);
String jsonString = mapper.writeValueAsString(javaObject);
System.out.println(jsonString);
}
}
class MyJavaObject {
private String name;
private int age;
public MyJavaObject(String name, int age) {
this.name = name;
this.age = age;
}
// getters and setters
}
JSON-java: This is a Java library that provides basic JSON support, including parsing and generation of JSON data. You can add the following dependency to your Maven or Gradle project:
net.sf.json-lib
json-lib
2.4
In your Java code, you can use the JSONObject
class to create a JSON object and add properties:
import net.sf.json.JSONObject;
public class Main {
public static void main(String[] args) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "John");
jsonObject.put("age", 30);
System.out.println(jsonObject.toString());
}
}
These are just a few examples of how you can include JSON in your Java application. Each library has its own strengths and weaknesses, so choose the one that best fits your needs.
Please note that these examples assume you're using Maven or Gradle as your build tool. If you're not using one of these tools, you'll need to manually download and add the libraries to your project.
Java json maven repository
I understand your request! Here's the information you're looking for:
Java, JSON, Maven, and Repository: A Comprehensive Guide!
What is a Repository?
A repository is a centralized location where software artifacts (such as code, configurations, or documentation) are stored and managed. In the context of Java development with Maven, a repository typically refers to an online storage facility that holds libraries, dependencies, and plugins used in your projects.
Why Use a Repository?
Using a repository has numerous benefits for developers:
Easily Share Code: A repository allows you to share code across different projects or teams. Version Control: Repositories keep track of changes made to the code over time, enabling efficient version control and collaboration. Dependency Management: Repositories enable seamless installation and management of required libraries, dependencies, and plugins. Faster Development: By providing access to pre-existing code and configurations, repositories can significantly speed up development processes.Java-related Repositories
Several popular Java-related repositories include:
Maven Central Repository: The official Maven repository, hosting a vast collection of open-source libraries and dependencies. Sonatype OSS Index: A comprehensive repository providing access to thousands of open-source software components. GitHub Packages: GitHub's own package registry, allowing developers to host their own packages and dependencies.JSON-related Repositories
For JSON-related requirements:
npm Registry: The official npm (Node Package Manager) repository for JavaScript libraries and modules. Maven JSON Library: A collection of JSON-related libraries and tools available on Maven Central Repository. GitHub Packages (JSON): GitHub's package registry includes various JSON-related libraries and dependencies.Integrating Repositories with Java and Maven
To leverage these repositories in your Java development workflow:
Add Repository Links: Configure your project settings to include the desired repository links. Maven Coordinates: Use Maven coordinates (groupId, artifactId, version) to specify the library or dependency you want to use.Example: Using a Repository with Maven
Suppose we need to add the popular JSON library Jackson in our Java project:
In yourpom.xml
file, add the following repository link:
central
Maven Central Repository
https://repo.maven.apache.org/maven2
Then, include the Jackson dependency in your pom.xml
:
com.fasterxml.jackson.core
jackson-databind
2.13.3
With these steps, Maven will automatically download and integrate the Jackson library into your project.
Conclusion
By utilizing a repository, you can streamline your Java development process by leveraging pre-existing libraries, dependencies, and plugins. Maven's central repository and other JSON-related repositories provide an excellent starting point for finding and integrating the perfect tools for your projects.