Java state pattern
Java state pattern
The State Pattern is a behavioral design pattern that allows an object to appear in different states throughout its lifetime. This can help to encapsulate the behavior of the object, making it more flexible and reusable.
In the context of Java, the State Pattern is implemented using a combination of abstract classes, concrete classes, and interfaces. Here's an overview of how it works:
Abstract Class:
The abstract class State
defines the interface for all states. It should have methods like handleRequest()
or executeAction()
that will be overridden by the concrete state implementations.
public abstract class State {
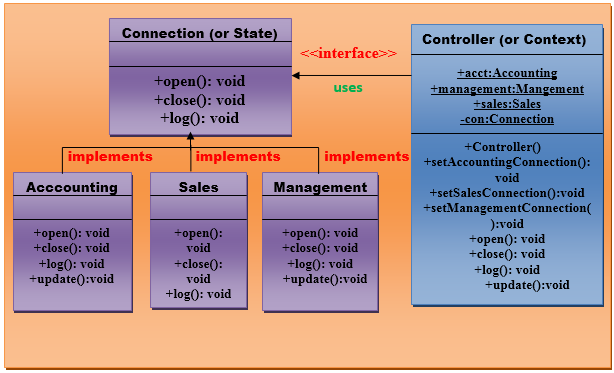
public abstract void handleRequest();
}
Concrete State Classes:
Each concrete state class implements the State
interface and provides its own implementation of the handleRequest()
method. The states should know how to transition between themselves based on certain conditions or events.
For example, let's say we're building a simple bank account management system with a few states like "open", "active", and "closed". Each state would have different behaviors:
public class OpenState extends State {
@Override
public void handleRequest() {
System.out.println("The account is open.");
}
}
public class ActiveState extends State {
@Override
public void handleRequest() {
System.out.println("The account is active.");
}
}
public class ClosedState extends State {
@Override
public void handleRequest() {
System.out.println("The account is closed.");
}
}
Context Class:
The context class (Account
, in our example) holds a reference to the current state object and delegates all requests to it. This way, the context doesn't need to know about the concrete states, making it easy to change or extend the system.
public class Account {
private State currentState;
public Account() {
this.currentState = new OpenState();
}
public void request(String action) {
this.currentState.handleRequest(action);
}
}
Client Code:
To use the state pattern, you would create an instance of the Account
class and interact with it through its methods. The request()
method delegates to the current state object, which then performs the requested action based on its implementation.
public class Client {
public static void main(String[] args) {
Account account = new Account();
account.request("open"); // The account is open.
account.request("activate"); // The account is active.
account.request("close"); // The account is closed.
account.request("reopen"); // The account is open again...
}
}
The State Pattern provides a powerful way to encapsulate complex behavior in Java, allowing you to design systems that adapt easily to changing conditions.
What is the state behavioral design pattern in Java?
I'm happy to help!
The State behavioral design pattern is used to allow an object's behavior to change based on its internal state. In other words, it allows an object to adapt its behavior according to its internal state.
In the context of Java, the State pattern can be implemented using an abstract class or interface that defines the common behavior and a set of concrete classes that extend this abstract class or implement this interface. Each concrete class represents a specific state of the object, and each instance of these classes maintains its own internal state.
Here's a step-by-step explanation of how to implement the State pattern in Java:
Define the Abstract Class: Create an abstract class or interface that defines the common behavior shared by all states. This class should have a set of methods that are common to all states, such ashandleRequest(request)
.
public abstract class State {
public abstract void handleRequest(String request);
}
Create Concrete States: Create concrete classes that extend the abstract State
class or implement the State
interface. Each concrete state represents a specific state of the object, and each instance of these classes maintains its own internal state.
For example, let's create two states: "New" and "Open". The "New" state is when an order is newly created, and the "Open" state is when an order is open for business.
public class NewState extends State {
public void handleRequest(String request) {
if (request.equals("open")) {
return new OpenState();
} else if (request.equals("close")) {
// do something
}
}
}
public class OpenState extends State {
public void handleRequest(String request) {
if (request.equals("close")) {
// do something
} else if (request.equals("open")) {
// do nothing, since it's already open
}
}
}
Create the Context: Create a context class that has a reference to a state object. This is where the magic happens! The context can delegate its behavior to the current state by calling the handleRequest(request)
method on the state object.
public class OrderContext {
private State state;
public OrderContext(State state) {
this.state = state;
}
public void request(String request) {
state.handleRequest(request);
}
}
Use the Context: Create instances of the context and states, and use them to adapt the behavior of the context based on its internal state.
public class Main {
public static void main(String[] args) {
State newOrderState = new NewState();
OrderContext orderContextNew = new OrderContext(newOrderState);
orderContextNew.request("new"); // do nothing, since it's already in the "new" state
orderContextNew.request("open"); // transition to the "open" state
}
}
In this example, when the context is first created with a NewState
, it remains in that state until the "open" request is made. Then, it transitions to the OpenState
and remains there.
The State pattern allows you to decouple the behavior of an object from its internal state. By doing so, you can create more flexible and dynamic systems where objects can adapt their behavior based on their internal state.
I hope this helps!