Thread pool implementation in java example
Thread pool implementation in java example
Here's an example of implementing a thread pool using Java:
What is a Thread Pool?
A thread pool is a mechanism that allows us to manage and reuse threads efficiently, which is crucial in modern computing systems where multithreading is the norm. A thread pool typically consists of a fixed number of worker threads that are created initially, and then reused as needed.
Why Do We Need Thread Pools?
Thread pools can help improve system performance by:
Reducing Thread Creation Overhead: Creating new threads can be an expensive operation. By reusing existing threads from the pool, we reduce the number of thread creations, which improves overall system efficiency. Managing Resources: A thread pool allows us to manage the number of active threads, preventing excessive resource consumption and potential system overload.How Do We Implement a Thread Pool in Java?
To implement a thread pool in Java, we can use an ExecutorService
from the java.util.concurrent
package. Here's a simple example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) throws InterruptedException {
// Create a thread pool with 5 worker threads
ExecutorService executor = Executors.newFixedThreadPool(5);
// Submit tasks to the executor
for (int i = 0; i < 10; i++) {
executor.submit(new Runnable() {
public void run() {
System.out.println("Thread " + Thread.currentThread().getName() + " is executing a task");
try {
Thread.sleep(1000); // Simulate some work
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
});
}
// Shutdown the executor to free up resources
executor.shutdown();
}
}
In this example, we create a thread pool with 5 worker threads using Executors.newFixedThreadPool(5)
. We then submit 10 tasks (represented as Runnable
instances) to the executor using the submit()
method. The executor will execute these tasks concurrently, reusing its worker threads whenever possible.
Key Benefits
Using an ExecutorService
like this example provides several benefits:
ExecutorService
does it for us. Improved Resource Management: The thread pool manages resources efficiently, preventing excessive resource consumption and system overload. Enhanced System Performance: By reusing existing threads from the pool, we reduce the overhead of creating new threads, improving overall system performance.
Conclusion
Thread pools are an essential tool for managing multithreading in modern computing systems. In Java, we can use ExecutorService
to create and manage thread pools efficiently. This example demonstrates how to implement a simple thread pool using Executors.newFixedThreadPool()
and execute tasks concurrently.
How to create a pool of threads in Java?
I'll make sure to respond in English.
To create a pool of threads in Java, you can use the Executor
and ExecutorService
interfaces provided by the Java concurrency API. Here's an example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Create a fixed-size thread pool with 5 threads
ExecutorService executor = Executors.newFixedThreadPool(5);
// Submit some tasks to the thread pool
for (int i = 0; i < 10; i++) {
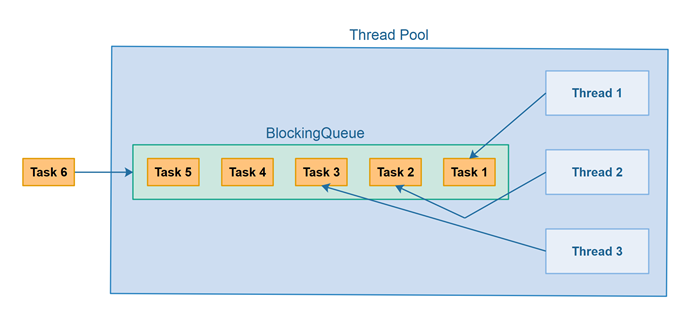
Runnable task = new MyRunnable(i);
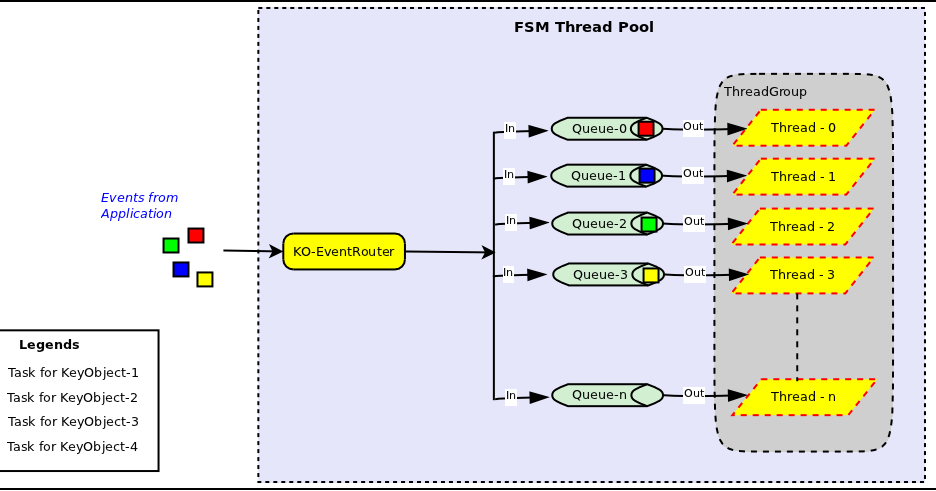
executor.submit(task);
}
// Shut down the thread pool
executor.shutdown();
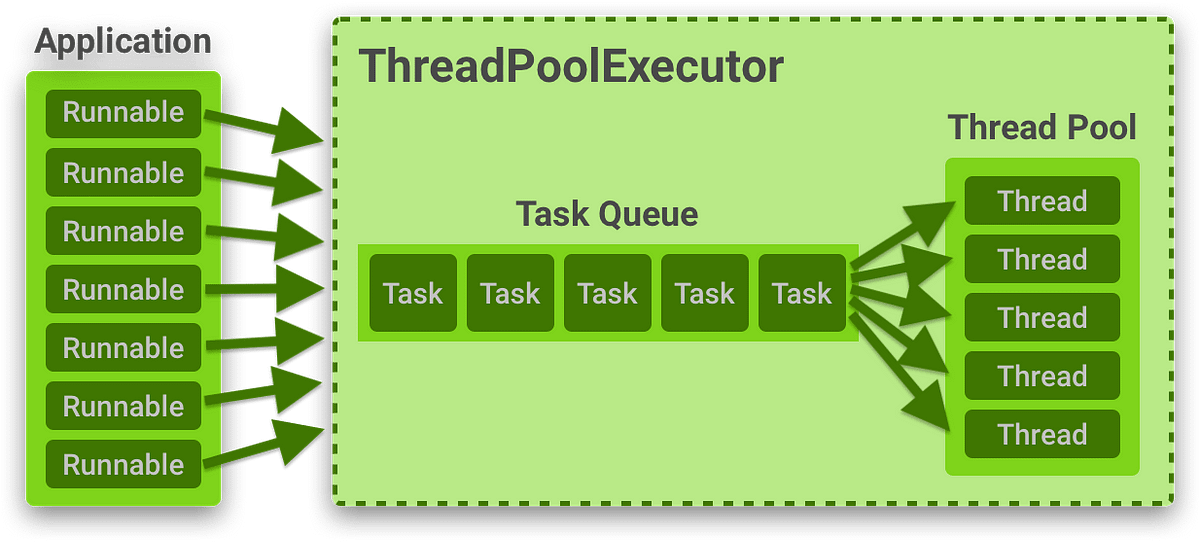
}
}
class MyRunnable implements Runnable {
private int id;
public MyRunnable(int id) {
this.id = id;
}
@Override
public void run() {
System.out.println("Thread " + id + " is running...");
try {
Thread.sleep(2000); // Simulate some work
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
In this example, we create a fixed-size thread pool with 5 threads using Executors.newFixedThreadPool(5)
. We then submit 10 tasks to the thread pool using executor.submit(task)
.
The MyRunnable
class is a simple example of a task that prints out its ID and simulates some work by sleeping for 2 seconds. Each instance of this task will be executed by one of the threads in the thread pool.
To create a pool of threads, you can use either a fixed-size or variable-size thread pool depending on your requirements. Here's how to do it:
Fixed-size thread pool: UseExecutors.newFixedThreadPool(int)
to create a pool with a specified number of threads. The pool will maintain that many threads even if they're idle. Variable-size thread pool: Use Executors.newCachedThreadPool()
or Executors.newScheduledThreadPool(int)
to create a pool that dynamically adjusts the number of threads based on demand. This can be useful for tasks that have varying levels of CPU-boundness. Single-threaded executor: Use Executors.newSingleThreadExecutor()
if you need an executor with only one thread, which can be useful when executing tasks that don't require concurrent execution.
When the task is finished or an error occurs, the thread pool will automatically terminate the thread. The executor.shutdown()
call is used to prevent any new tasks from being submitted after a certain point.
By using the Executor
and ExecutorService
interfaces, you can simplify the creation of threads and manage their lifecycle without having to manually create and manage them.