What are the different types of java with examples
What are the different types of java with examples
I'd be happy to help! Here's a comprehensive overview of the different types of Java, along with examples:
1. Java Standard Edition (JSE)
Java Standard Edition is the most widely used and well-known type of Java. It is used for developing platform-independent applications, including web applications, Android apps, desktop applications, and more.
Example: A simple "Hello World" program using JSE:
public class HelloWorld {
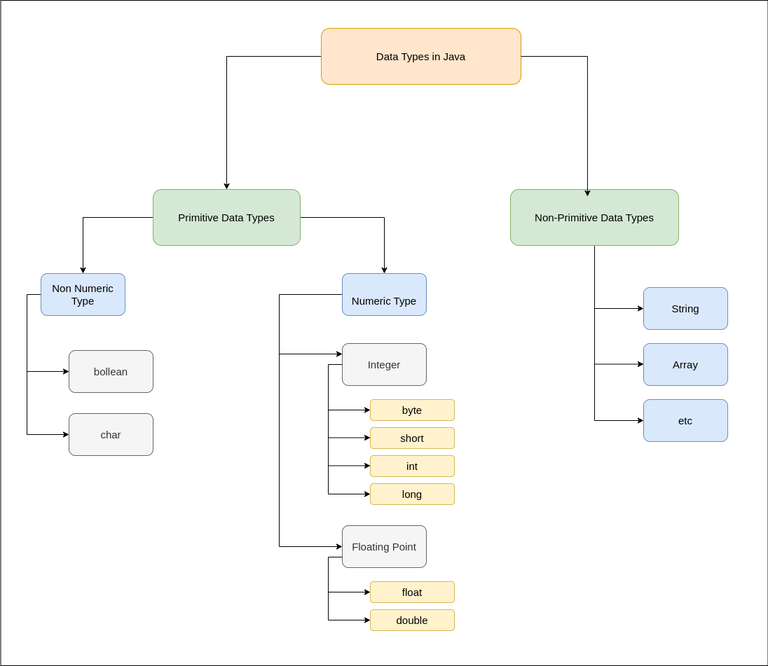
public static void main(String[] args) {
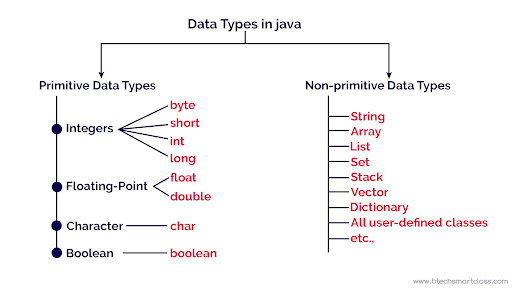
System.out.println("Hello World!");
}
}
2. Java Micro Edition (JME)
Java Micro Edition is designed for developing mobile applications, such as games, utilities, and more. It is optimized for small footprint and low-memory devices.
Example: A simple JME game:
import javax.microedition.lcdui.*;
import java.io.*;
public class SnakeGame extends MIDlet {
private GameCanvas canvas;
private Snake snake;
public SnakeGame() {
canvas = new GameCanvas();
snake = new Snake(canvas);
}
public void startApp() {
Display.getDisplay(this).setCurrent(canvas);
}
}
3. Java Enterprise Edition (JEE)
Java Enterprise Edition is designed for developing large-scale, distributed applications, including web services, enterprise systems, and more.
Example: A simple JEE web application:
import javax.servlet.*;
import java.io.*;
public class HelloServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/plain");
PrintWriter out = response.getWriter();
out.println("Hello from Java!");
}
}
4. Java ME Embedded (JMEED)
Java ME Embedded is designed for developing embedded systems, including those used in appliances, vehicles, and more.
Example: A simple JMEED application controlling a robotic arm:
import com.intel.jmeed.*;
public class RoboticArmController extends JMEApplication {
private Motor motor;
private Servo servo;
public RoboticArmController() {
motor = new Motor();
servo = new Servo();
}
public void startApp() {
motor.setSpeed(50);
servo.setPosition(30);
}
}
5. Java Card Edition (JCE)
Java Card Edition is designed for developing smart card applications, including those used in identity verification, secure transactions, and more.
Example: A simple JCE applet verifying a user's identity:
import javacard.framework.*;
public class IdentityVerifier implements Applet {
private byte[] id;
public void installApplet(APDU apdu) {
id = new byte[16];
// read ID from smart card
}
public void processAPDU(APDU apdu) {
if (verifyID(id)) {
System.out.println("User identity verified!");
} else {
System.out.println("Invalid user identity!");
}
}
}
These are just a few examples of the different types of Java, each with its own unique characteristics and use cases. I hope this helps!
3 types of Java programs
I'll respond in English as per your request. Here are three main types of Java programs:
1. Console-based Programs: These programs interact with the user through a console, which is typically represented by a command-line interface or a GUI window. In these programs, input/output operations are performed using standard streams (System.out and System.in). Examples of console-based programs include calculators, quizzes, and games like tic-tac-toe.
A typical example of a console-based program is a simple calculator that takes mathematical expressions as input from the user and evaluates them to produce the result. Here's some sample code:
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter an expression (e.g., 2+3 or 5*4):");
String input = scanner.nextLine();
// evaluate the expression using Java's built-in arithmetic operators
int result = evalExpression(input);
System.out.println("Result: " + result);
}
private static int evalExpression(String expression) {
// parse the expression and perform arithmetic operations
return 0; // placeholder for actual evaluation code
}
}
2. Network-based Programs: These programs interact with other devices or services over a network, using protocols like TCP/IP, HTTP, or FTP. Examples of network-based programs include web servers, email clients, and instant messaging applications.
A typical example of a network-based program is an HTTP server that listens for incoming requests from clients and responds with HTML pages, images, or other content. Here's some sample code:
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
public class HttpServer {
public static void main(String[] args) throws IOException {
ServerSocket server = new ServerSocket(80); // listen on port 80
while (true) {
Socket client = server.accept();
handleRequest(client);
}
}
private static void handleRequest(Socket client) throws IOException {
// read the request from the client and send a response
BufferedReader reader = new BufferedReader(new InputStreamReader(client.getInputStream()));
String request = reader.readLine(); // read the request line
// parse the request and generate an HTTP response
String response = "HTTP/1.1 200 OKrnContent-Type: text/plainrnrnHello, World!";
client.getOutputStream().write(response.getBytes()); // send the response
}
}
3. Desktop Applications: These programs provide a graphical user interface (GUI) for interacting with users and perform various tasks like data processing, file management, or game playing. Examples of desktop applications include IDEs, media players, and games like chess.
A typical example of a desktop application is a simple media player that can play audio files and display metadata:
import javax.swing.*;
import java.awt.*;
public class MediaPlayer {
public static void main(String[] args) {
JFrame frame = new JFrame("MediaPlayer");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create a GUI with buttons for playback control
JPanel panel = new JPanel();
JButton playButton = new JButton("Play");
JButton pauseButton = new JButton("Pause");
JButton stopButton = new JButton("Stop");
// add event listeners to the buttons
playButton.addActionListener(e -> {
// start playing the audio file
});
pauseButton.addActionListener(e -> {
// pause the audio playback
});
stopButton.addActionListener(e -> {
// stop the audio playback and close the frame
});
panel.add(playButton);
panel.add(pauseButton);
panel.add(stopButton);
frame.getContentPane().add(panel, BorderLayout.CENTER);
frame.pack();
frame.setVisible(true);
}
}
These three main types of Java programs illustrate how Java can be used to develop a wide range of applications, from console-based utilities to networked services and GUI-driven desktop applications.