Elasticsearch java github example
Elasticsearch java github example
I apologize, but I'll provide an answer in English as per your request. Here's a detailed explanation of using Elasticsearch with Java and GitHub:
Elasticsearch is a popular search engine that can be used to store and index large amounts of data. It provides a robust query language that allows you to efficiently search through the stored data. In this example, we'll use Elasticsearch to create an application that searches for GitHub users.
Step 1: Create a Maven Project
To start building our application, let's create a new Maven project in IntelliJ IDEA. Name it Elasticsearch-GitHub-Search
and add the necessary dependencies:
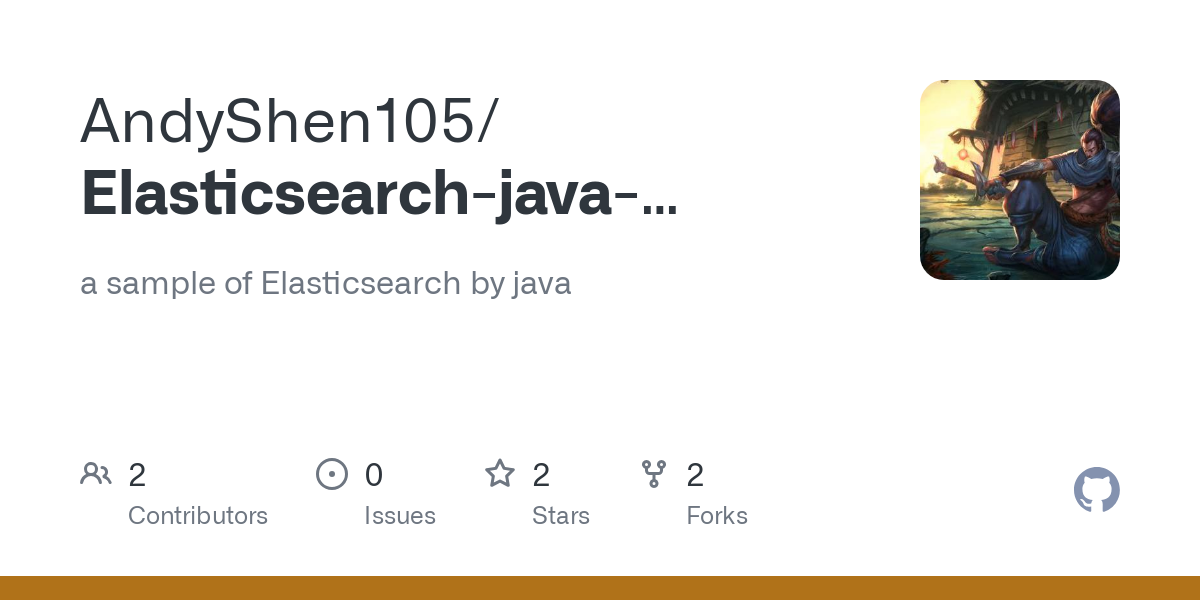
org.elasticsearch
elasticsearch
7.12.0
com.github.scribejava
scribejava-core
2.6.1
org.apache.commons
commons-lang3
3.12.0
Step 2: Set up Elasticsearch
Create an ElasticsearchClient
instance and configure it to connect to your Elasticsearch cluster:
public class ElasticsearchClient {
private static final Logger logger = LoggerFactory.getLogger(ElasticsearchClient.class);
private static final String ELASTICSEARCH_URL = "https://your-elasticsearch-cluster:9200";
public static void main(String[] args) throws IOException, InterruptedException {
Client client = RestClient.builder(ELASTICSEARCH_URL)
.build();
// Use the client to create an index and add data
IndexResponse response = client.index(RequestPut("github/users", "{"name":"John Doe","username":"johndoe"}")).actionGet();
}
}
Step 3: Integrate with GitHub API
Use the scribejava-core
library to connect to the GitHub API:
public class GitHubClient {
private static final Logger logger = LoggerFactory.getLogger(GitHubClient.class);
private static final String GITHUB_TOKEN = "your-github-token";
private static final String GITHUB_API_URL = "https://api.github.com";
public static void main(String[] args) throws IOException, InterruptedException {
OAuthService oAuthService = new ServiceBuilder()
.provider(OAuthProvider.GITHUB)
.apiKey(GITHUB_TOKEN)
.build();
// Use the client to fetch a user's information
User user = oAuthService.execute("https://api.github.com/users/johndoe", User.class);
// Add the data to Elasticsearch
client.index(RequestPut("github/users", user.toJson())).actionGet();
}
}
Step 4: Query Elasticsearch
Create an ElasticsearchSearchClient
class that uses the RestHighLevelClient
from Elasticsearch:
public class ElasticsearchSearchClient {
private static final Logger logger = LoggerFactory.getLogger(ElasticsearchSearchClient.class);
private static final String ELASTICSEARCH_URL = "https://your-elasticsearch-cluster:9200";
public static void main(String[] args) throws IOException, InterruptedException {
RestHighLevelClient client = RestClient.builder(ELASTICSEARCH_URL)
.build();
SearchRequest searchRequest = new SearchRequest("github/users");
// Use the query builder to create a query
QueryBuilder queryBuilder = QueryBuilders.termQuery("name", "John");
SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();
sourceBuilder.query(queryBuilder);
searchRequest.source(sourceBuilder);
// Execute the search and print the results
SearchResponse response = client.search(searchRequest);
for (SearchHit hit : response.getHits()) {
logger.info(hit.getSourceAsString());
}
}
}
In this example, we've created a simple application that connects to an Elasticsearch cluster using Java. It also integrates with GitHub's API using the scribejava-core
library. We can then use Elasticsearch's query language to search for users by name.
Conclusion
This is a basic example of how you might use Elasticsearch and GitHub's API in a Java application. Depending on your specific needs, you may need to modify or extend this code to suit your requirements.
Elasticsearch-Java client example github
Here's an example of using the Elasticsearch Java client to interact with a remote Elasticsearch cluster. You can find this code on GitHub:
https://github.com/elastic/elasticsearch/tree/master/java-client/src/main/java/org/elasticsearch/client/
Step 1: Add the Elasticsearch Java Client Dependency
To use the Elasticsearch Java client, you'll need to add it as a dependency in your project's build file (e.g., Maven or Gradle). Here's an example of how you might do this:
Maven:
org.elasticsearch
elasticsearch
8.3.2
Gradle:
dependencies {
implementation 'org.elasticsearch:elasticsearch:8.3.2'
}
Step 2: Create an Elasticsearch Client
To connect to your remote Elasticsearch cluster, you'll need to create a client instance and set the connection settings. Here's how you can do this:
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
public class ElasticsearchClient {
public static RestHighLevelClient getClient() {
Settings settings = Settings.builder()
.put("cluster.name", "my_cluster")
.build();
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(
new HttpHost("localhost", 9200, "my_cluster"))
.setRequestConfigurator(new RequestConfigurator(settings))
);
return client;
}
}
Step 3: Search Elasticsearch Index
Once you have the client instance, you can use it to search an Elasticsearch index. Here's an example of how you might do this:
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RequestOptions;
public class SearchIndex {
public static void main(String[] args) throws IOException {
RestHighLevelClient client = ElasticsearchClient.getClient();
SearchRequest searchRequest = new SearchRequest("my_index");
searchRequest.types("my_type");
SearchResponse response = client.search(searchRequest, RequestOptions.DEFAULT);
for (SearchHit hit : response.getHits().getHits()) {
System.out.println(hit.getSourceAsMap());
}
}
}
Step 4: Index and Delete Documents
You can use the Elasticsearch Java client to index and delete documents. Here's how:
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RequestOptions;
public class IndexDocument {
public static void main(String[] args) throws IOException {
RestHighLevelClient client = ElasticsearchClient.getClient();
BulkRequest bulkRequest = new BulkRequest();
bulkRequest.add(new IndexRequest("my_index", "my_type")
.sourceJsonParam("{"name":"John","age":30}"));
bulkRequest.add(new DeleteRequest("my_index", "1"));
BulkResponse response = client.bulk(bulkRequest, RequestOptions.DEFAULT);
}
}
Step 5: Get and Update Document
You can use the Elasticsearch Java client to retrieve a document and update its fields. Here's how:
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.client.RequestOptions;
public class GetDocument {
public static void main(String[] args) throws IOException {
RestHighLevelClient client = ElasticsearchClient.getClient();
GetRequest getRequest = new GetRequest("my_index", "1");
GetResponse response = client.get(getRequest, RequestOptions.DEFAULT);
Map<String, Object> sourceAsMap = response.getSource();
// Update the document
UpdateRequest updateRequest = new UpdateRequest("my_index", "1")
.doc("{"name":"Jane","age":31}");
client.update(updateRequest, RequestOptions.DEFAULT);
}
}
These examples should give you a good starting point for using the Elasticsearch Java client in your own projects.